In today’s guide, we will explore two crucial modules, “datetime
” and “time
” that enables you to get the current date and time in Python.
The “datetime
” module offers multiple functionalities including date and time manipulation, formatting, and approaches to handle time zones.
On the other hand, the “time
” module is good at performing simpler time-related operations and Unix timestamps.
So, let’s start this guide and check out the methods for retrieving the current date and time in the program with the discussed modules.
How to Get Current Date and Time in Python
In order to get or fetch the current date and time in Python, you can utilize the “datetime
” or the “time
” module. More specifically, the datetime
module offers more functionalities and is considered ideal for most date and time-related operations.
Whereas, the time module supports simpler functions that can be primarily used for timing and benchmarking purposes.
What is a “datetime” Module in Python
As discussed earlier, the “datetime
” module is recommended for performing the tasks related to the date and time-related operations.
Additionally, it can be used for fetching the current date and time in Python. To do so, check out the below-provided instructions.
1. Import the “datetime” Module
First of all, it is required to import the “datetime
” module for working with date and time functions. For the corresponding purpose, add the following import statement as follows.
import datetime
2. Get Current Date in Python
The “date.today()
” method of the “datetime
” module can be invoked for getting the current date. This method outputs a date object which represents the current or today’s date.
import datetime current_date = datetime.date.today() print("Current Date:", current_date)
As you can see, the print() function displays the current date stored in the “current_date
“.
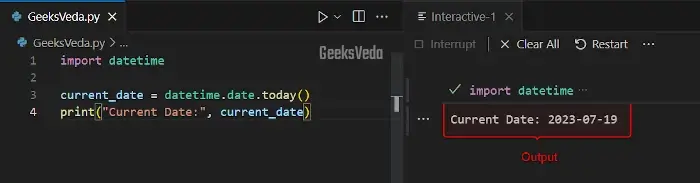
3. Get Current Time
The “datetime
” module offers another method “datetime.now()
” that can retrieve the current time. It outputs a datetime object that signifies today’s date and current time.
import datetime current_time = datetime.datetime.now() print("Current Time:", current_time)
It can be observed that today’s date and the current time have been shown on the console.
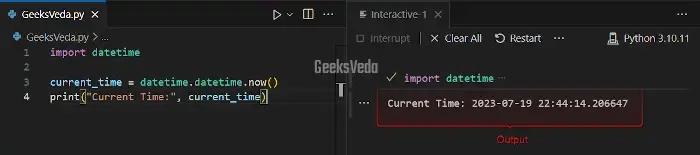
4. Combine Date and Time
There exist scenarios where it is required to combine a particular date with a certain time for creating a single “datetime
” object.
For instance, in the given code, firstly we have created a date object named “date_obj
” and a time object named “time_obj
“.
Then, we displayed the combined date and time using the “datetime.combine()
” method.
import datetime date_obj = datetime.date(2023, 7, 19) time_obj = datetime.time(12, 34, 56) combined_datetime = datetime.datetime.combine(date_obj, time_obj) print("Combined DateTime:", combined_datetime)
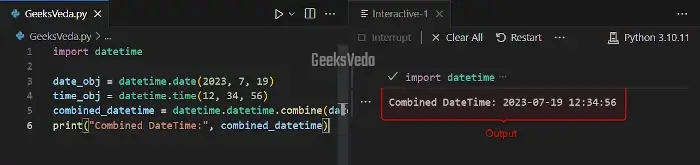
5. Format Dates and Times
Python offers the strftime() method for formatting dates and times as per our needs. In this method, format codes can be added for specifying the desired format.
Here, in the given program, firstly we will retrieve the current date and time by invoking the “datetime.now()
” method.
After that, it will be formatted into the defined format “%Y-%m-%d %H:%M:%S
“.
import datetime current_datetime = datetime.datetime.now() formatted_datetime = current_datetime.strftime("%Y-%m-%d %H:%M:%S") print("Formatted DateTime:", formatted_datetime)
The following output displayed the formatted date and time on the console.
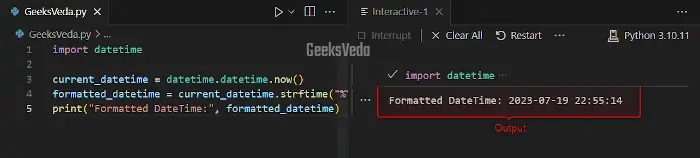
6. Handling Time Zones
Working with time zones is essential for dealing with global data and time conversions. For this purpose, Python provides the “pytz
” library for handling time zones effectively.
According to the following code, we retrieved the current date and time with “datetime.now()
” and then localized it to the “America/New_York
” with the “timezone.localize()
” method.
In the end, the localized date and time will be printed on the console.
import datetime import pytz current_datetime = datetime.datetime.now() timezone = pytz.timezone('America/New_York') localized_datetime = timezone.localize(current_datetime) print("Localized DateTime:", localized_datetime)
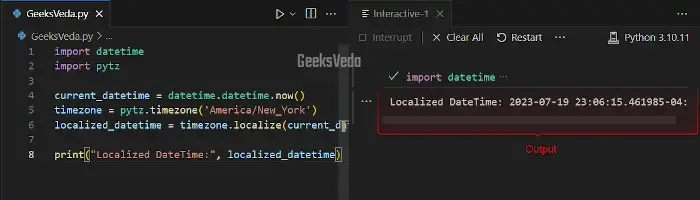
What is the “time” Module in Python
Python’s “time
” module offers simple functions to work with the operations or actions related to time. More specifically, it can be utilized for tasks in which you want to measure the code execution time, handle basic functionalities, and timestamp format.
1. Import the “time” Module
In order to use the “time
” module, import it first by adding the following statement.
import time
2. Get the Current Unix Timestamp
The Unix timestamp signifies the number of seconds elapsed since January 1, 1970 (epoch time). For getting the Unix timestamp, the “time()
” function of the time module can be invoked.
Now, let’s obtain the current Unix timestamp with the “time.time()
” method and then display it on the console.
import time current_timestamp = time.time() print("Current Unix Timestamp:", current_timestamp)
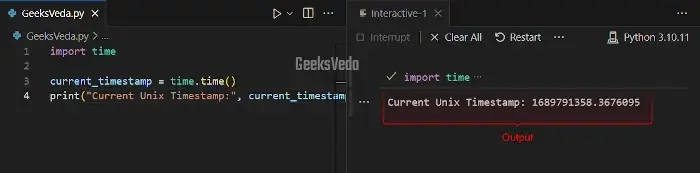
3. Convert Unix Timestamp to Readable Format
Unix timestamps are considered for calculations, however, they are not human-readable. Therefore, the “ctime()
” function can be invoked for converting the Unix timestamp into a readable or human format.
In this program, firstly, we fetched the current Unix timestamp with the “time.time()
” method and then converted it to human-readable format with the “ctime()
” method as follows.
import time current_timestamp = time.time() readable_time = time.ctime(current_timestamp) print("Readable Time:", readable_time)
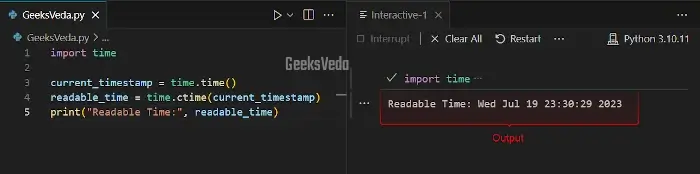
Log Timestamp in Python Applications
Logging timestamps in Python applications is essential for debugging and tracking events.
Additionally, the “datetime
” module supports an easy way for adding timestamps for logging messages.
The below example demonstrates the method to log messages with timestamps in Python with the “logging
” module. We have configured the logging here with the format for including the timestamps.
As a result, it logs messages at different levels such as INFO, WARNING, and ERROR, with the corresponding timestamps.
import logging import datetime # Configure logging logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s') # Log messages with timestamps logging.info('Application started') logging.warning('This is a warning message') logging.error('An error occurred')
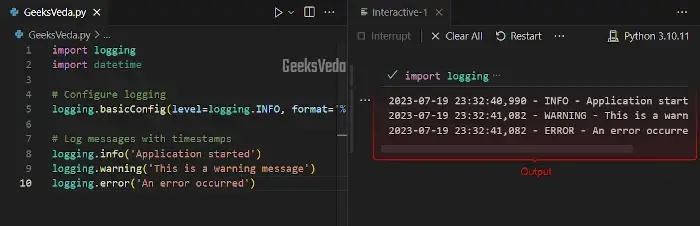
Schedule Tasks with Date and Time in Python
You can also utilize the “datetime” module to schedule tasks or operations at specific dates and times. Moreover, this functionality can help you to automate tasks like sending scheduled emails or data backups.
For instance, here, we will schedule a task for the defined date and time with “datetime()
“. Then, it waits until the current time equals the specified time because of the added loop and time.sleep() method.
Lastly, when the scheduled time is reached, the task will run and the current time will be displayed.
import datetime import time # Schedule a task for a specific date and time scheduled_time = datetime.datetime(2023, 7, 30, 10, 30, 0) while datetime.datetime.now() < scheduled_time: time.sleep(1) print("Task executed at:", datetime.datetime.now())
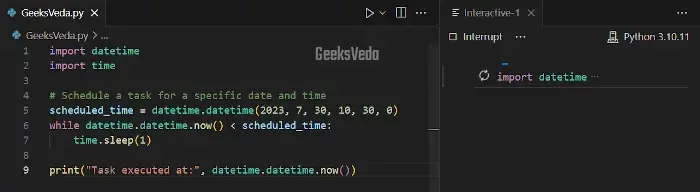
Handling Time-based Events in Python
In applications, time-based events can be managed with the help of the “datetime
” module for triggering particular actions at predefined times or intervals.
Here, firstly, we have defined a time interval with the “datetime.timedelta()
” method and then performed the specified event repeatedly at the given interval.
More specifically, the for loop and the time.sleep()
method can be added for this purpose. Lastly, the current time has been displayed each time the event occurs.
import datetime import time # Define the time interval for the event (every 5 seconds in this example) time_interval = datetime.timedelta(seconds=5) # Perform the event at the specified interval while True: # Your code for the time-based event here... print("Time-based event occurred at:", datetime.datetime.now()) time.sleep(time_interval.total_seconds())
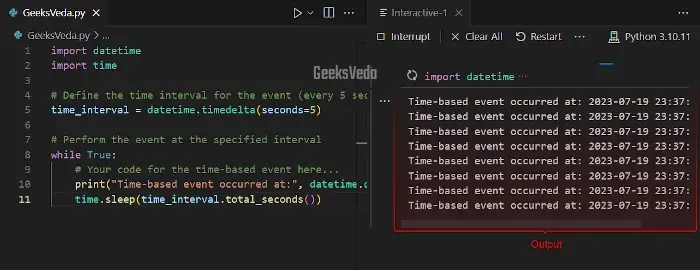
Python “datetime” VS “time” Module
Check out the provided table for comparing “datetime
” and “time
“approach based on the enlisted features:
Feature | “datetime” Approach | “time” Approach |
Functionalities | Extensive date and time handling | Basic time-related operations |
Current/Today’s Date | datetime.date.today() | N/A (not available in “time” module) |
Current Time | datetime.datetime.now() | time.time() |
Combining Date and Time | datetime.datetime.combine() | N/A (not available in “time” module) |
Formatting Dates and Times | strftime() method | N/A (not available in “time” module) |
Handling Time Zones | pytz library (or datetime.astimezone()) | N/A (not available in “time” module) |
That’s all from this effective guide for getting the current date and time in Python.
Conclusion
Python’s “datetime
” and “time
” modules offer versatile tools for managing dates, times, and time-related tasks. More specifically, the “datetime
” module offers several functionalities for date and time manipulation, formatting, and time zone handling.
Whereas, the “time
” module focuses on simple time-related operations and Unix timestamps.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!