Being the most powerful programming language, Python offers a type conversion system that enables you to handle multiple data types.
It also includes both implicit and explicit conversion. So, in today’s guide, we will learn about data types, type conversion, and how to adapt to different values effortlessly in Python.
Let’s unlock the mystery of Python-type conversion!
What is Data Types in Python
Data types in Python refer to the classification of data values that determine the operations or actions that can be performed on them. Moreover, as Python is a dynamically-typed language, this signifies that it is not required to explicitly declare the variable type. Instead, Python decides their data type based on the values assigned at runtime.
Python Immutable vs Mutable Data Types
Python data types are categorized into two categories: Immutable and Mutable.
Immutable data types are those values that cannot be changed or modified after creation. More specifically, if you try to modify an immutable object, a new object is created in memory.
Here are some of the examples of Python immutable data types:
- int (integer): x = 5
- float (floating-point number): pi = 3.14
- str (string): name = Sharqa
- tuple: coordinates = (30, 40)
Whereas, the mutable Python data types can be modified or changed after creation. Note that, when you change a mutable object, it changes in place, and the memory address also remains the same.
Look at the given examples of Python mutable data types:
- list: numbers = [7,8,9]
- dict (dictionary): author = {‘name’: ‘Sharqa’, ‘age’: 25}
- set: colors = {‘pink’, ‘blue’, ‘white’}
What is Type Inference in Python
Type inference is a Python feature that enables the interpreter to automatically find out the data type of the variable based on the assigned value. It makes Python a concise and highly flexible language because you do not have to declare types explicitly.
More specifically, type inference enhances readability, simplifies coding, and also minimizes the chances of getting a type-related error.
Here is an example of type inference:
# Type inference in Python x = 5 # x is inferred as an integer name = "Sharqa" # name is inferred as a string pi = 3.14 # pi is inferred as a float
Python Implicit Type Conversion
Type Coercion or Implicit type conversion is known as the automatic conversion of one data type to another. This conversion is done by the Python interpreter and it occurs when an expression comprises an operand having different data types.
In this process, Python tries to make a conversion that avoids data loss and also preserves the most relevant information.
For instance, in the provided code, Python will perform an implicit type conversion for converting the “num_int
” integer to a float before adding it to “num_float
“.
Lastly, the “result
” variable will be of float type.
# Implicit type conversion num_int = 5 num_float = 3.14 result = num_int + num_float print(result)
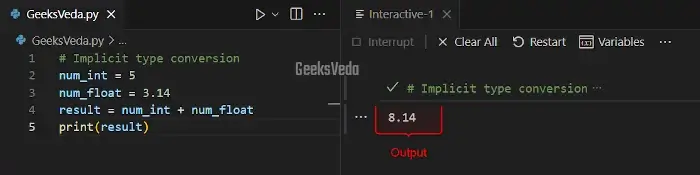
Python Explicit Type Conversion
Explicit conversion or Type Casting is known as the manual conversion of one data type into another. This can be done by the programmer or developers. As discussed earlier, Python handles the conversion automatically in implicit conversion.
However, the explicit type conversion offers you control over how the conversion is performed.
int()
“, “float()
“, “list()
“, “str()
“, “tuple()
”, “set()
“, and “dict()
” can be utilized for the typecasting as per requirements.Here, we have invoked the str() function for converting the “num_int
” integer to a string. The resultant value will be displayed by calling the print() function.
# Explicit type conversion num_int = 10 num_str = str(num_int) print(num_str)
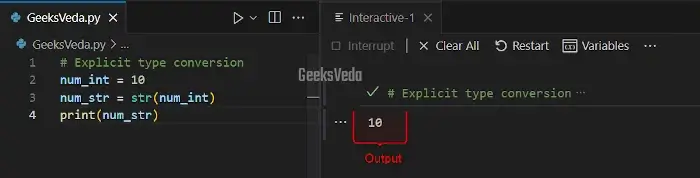
This type of conversion can be particularly useful when you need to ensure the data type before performing a particular operation.
Python Numeric Type Conversion
Numeric type conversion is based on the approach of converting different numeric data types, like floats
, integers
, and complex
numbers.
It can be done by utilizing the built-in functions of Python.
1. Convert Integer to Float
Python allows you to convert an integer to float using the “int()
” function and float to int with the “float()
” method respectively.
For example, in the given program, we will convert the “num_int
” integer to float and the value of the float variable “num_float
” to an integer.
# Numeric Type Conversion - Integers and Floats num_int = 10 num_float = 3.14 converted_int = int(num_float) converted_float = float(num_int) print("Converted int value:", converted_int) print("Converted float value:", converted_float)
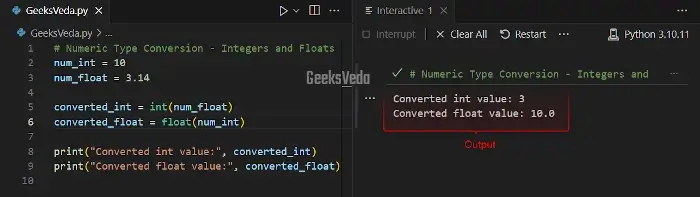
2. Convert Real and Imaginary Parts to Complex Numbers
Additionally, Python also permits you to create complex numbers from real and imaginary parts using the “complex()
” function, as we did here.
# Numeric Type Conversion - Complex Numbers real_num = 2 imaginary_num = 3 complex_num = complex(real_num, imaginary_num) print(complex_num)
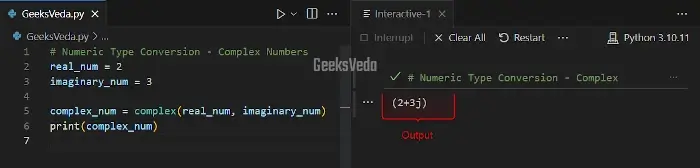
Python String Type Conversion
In String type conversion, you can convert the strings to the desired data type. For instance, convert string to integers, floats, or other numeric values.
For instance, we will now convert two strings “num_str
” and float_str
” to integer and float data types using the “int()
” and “float()
” functions, respectively.
# String Type Conversion num_str = "35" float_str = "3.14" # Converting strings to numeric data types num_int = int(num_str) num_float = float(float_str) print(num_int) print(num_float)
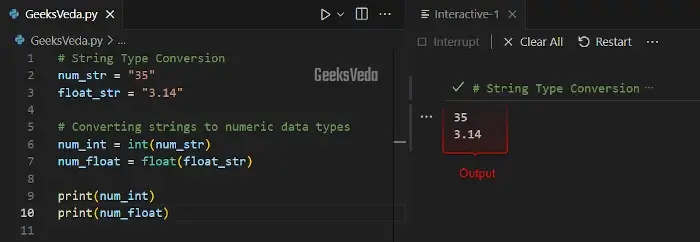
Python Boolean Type Conversion
In this type of conversion, you can convert values to boolean data types, such as “True
” or “False
“. Remember that, in Python, some particular values can be truthy or false, which signifies that they can be implicitly converted to boolean values as listed below:
- Truthy Values: Non-empty, non-zero, non-null values are considered True.
- Falsy Values: Empty, zero, or None values are considered False.
In this example, we have converted a “truthy_value
” and a “falsy_value
” to their respective boolean values.
# Boolean Type Conversion # Truthy values truthy_value = "Hi GeeksVeda User" is_true = bool(truthy_value) # Falsy values falsy_value = 0 is_false = bool(falsy_value) print(is_true) print(is_false)
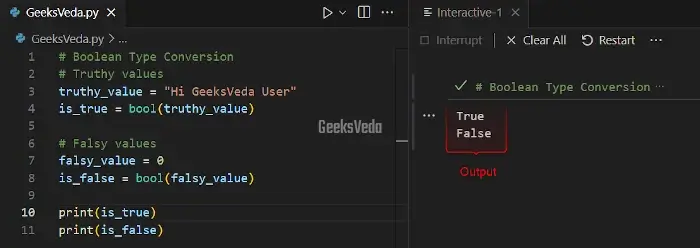
Python Lists Type Conversion
The Lists are the most Python versatile data structures that can be needed to convert into other types, such as sets, tuples, or dictionaries.
For example, we will now convert an “authors_list
” having three authors’ name and then convert it to a tuple, a set, and a dictionary with “tuple()
“, and “set()
” functions, and using the indexes as keys with the enumerate() function for dictionary conversion, respectively.
# Lists Type Conversion authors_list = ['Ravi', 'Sharqa', 'Tulsi'] # Converting a list to a tuple authors_tuple = tuple(authors_list) # Converting a list to a set authors_set = set(authors_list) # Converting a list to a dictionary with index as keys authors_dict = {index: fruit for index, fruit in enumerate(authors_list)} print(authors_tuple) print(authors_set) print(authors_dict)
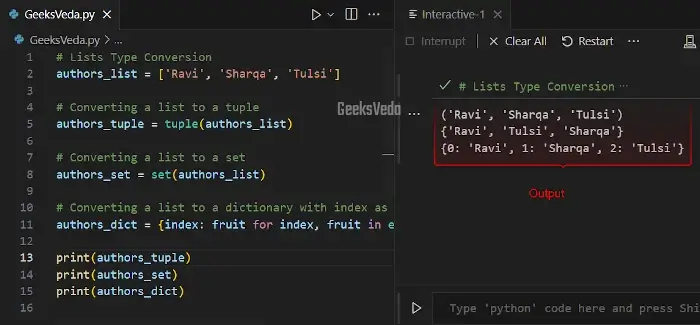
Python Tuple Type Conversion
Tuples
are immutable. However, you can convert them to other data types, such as lists and sets, as demonstrated below.
# Tuple Type Conversion coordinates_tuple = (40, 50) # Converting a tuple to a list coordinates_list = list(coordinates_tuple) # Converting a tuple to a set coordinates_set = set(coordinates_tuple) print(coordinates_list) print(coordinates_set)
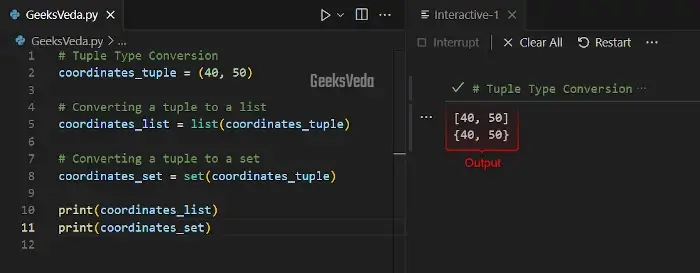
Python Set Type Conversion
Sets
are the unordered collection but can be converted into lists, types, or dictionaries as demonstrated as follows.
# Set Type Conversion colors_set = {'pink', 'blue', 'white'} # Converting a set to a list colors_list = list(colors_set) # Converting a set to a tuple colors_tuple = tuple(colors_set) # Converting a set to a dictionary with set elements as keys and None as values colors_dict = {color: None for color in colors_set} print(colors_list) print(colors_tuple) print(colors_dict)
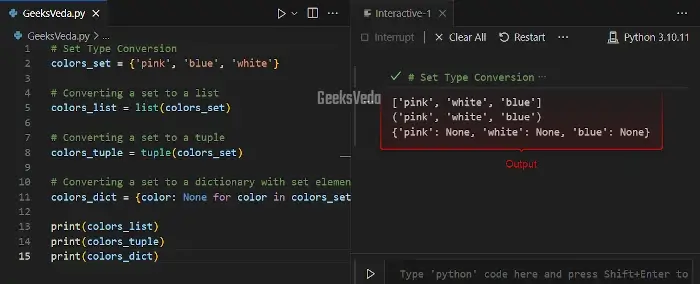
Python Dictionary Type Conversion
Last but not least, Python also permits you to convert dictionaries into lists, tuples, or sets as per data manipulation requirements.
For instance, in the provided code, we first converted the “person_dict” dictionary into a list of keys using the list() function. After that, we invoked the “tuple()” function to convert the original dictionary to a tuple of values.
Lastly, the “person_dict
” has been converted into a set of key values with the “set()
” function.
# Dictionary Type Conversion person_dict = {'name': 'Sharqa', 'age': 25, 'city': 'Islamabad'} # Converting a dictionary to a list of keys keys_list = list(person_dict.keys()) # Converting a dictionary to a tuple of values values_tuple = tuple(person_dict.values()) # Converting a dictionary to a set of key-value pairs (tuples) items_set = set(person_dict.items()) print(keys_list) print(values_tuple) print(items_set)
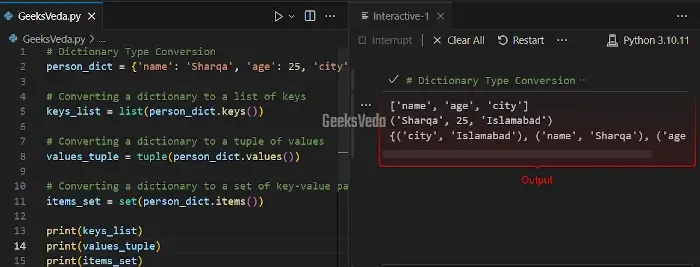
That’s all from today’s guide related to Python type conversion.
Conclusion
Python type conversion concept plays an essential part in manipulating data in programs. It also ensures the capability of handling several data types.
Additionally, implicit type conversion can be applied for simplifying the operations by automatically converting the values when required. On the other hand, explicit type conversion enables you to control the data types.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!