Printing messages to the console is a fundamental skill for JavaScript developers, as it is often used for debugging, logging, and testing code. Using the various JavaScript console methods available, you can customize the output and gain valuable insights into your code’s behavior.
The following is the console method example.
console.log("This is a message");
Printing messages to the console is necessary for several reasons, such as:
- Debugging – When you encounter errors in your code, you can use console messages to help identify the source of the problem and troubleshoot your code.
- Logging – You can use console messages to track the progress of your code and monitor important variables or events.
- Testing – You can use console messages to verify that your code is working as expected and to compare the actual output with the expected output.
To print messages to the console using JavaScript, you should understand the language’s syntax and the development environment you are working with.
This article will explore six different methods for printing custom messages to the console in JavaScript, along with code samples and explanations.
1. Using JavaScript console.log() Method
To print any message in the console using JavaScript, you can use the console.log()
method, which takes one or more parameters and outputs them to the console.
Here’s an example of console.log()
method:
console.log("This is a log message");
Running the above code in your developer console browser or a Node.js environment will print “This is a message” to the console.
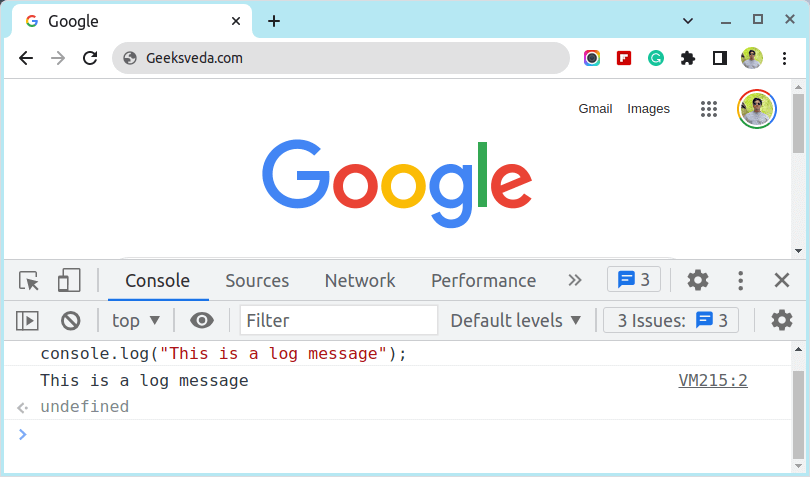
2. Using JavaScript console.warn() Method
The console.warn()
method displays a warning message to the console in yellow.
Here’s an example of console.warn()
method:
console.warn("This is a warning message");
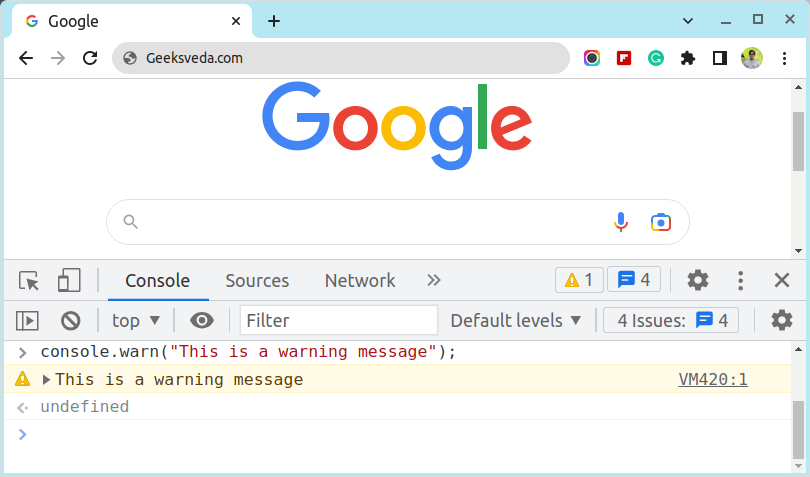
3. Using JavaScript console.error() Method
The console.error()
method displays an error message to the console, usually displayed in red.
Here’s an example of console.error()
method:
console.error("This is an error message");
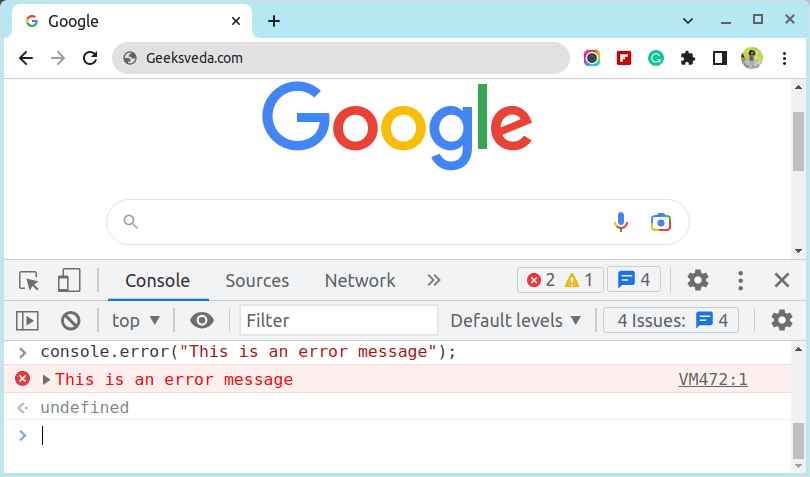
4. Using JavaScript console.info() Method
When you decide to use the console.error()
method, it outputs an information message to the console, usually displayed in blue.
Here’s an example of console.info()
method:
console.info("This is an info message");
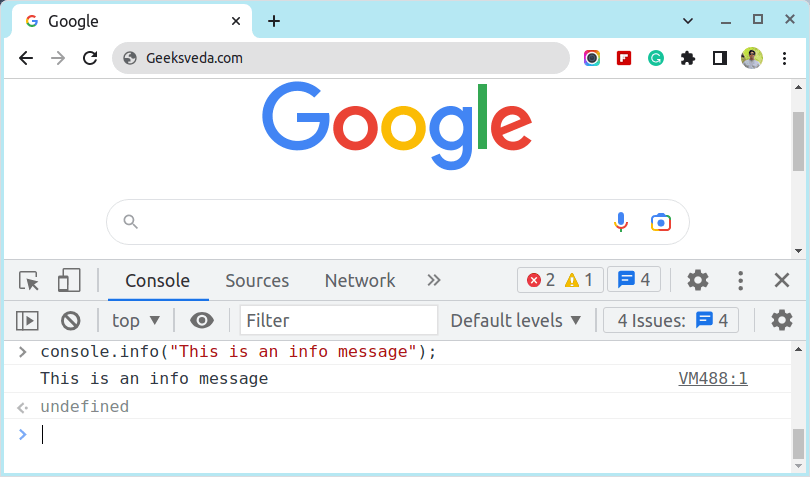
5. Using JavaScript console.table() Method
The console.table()
method outputs a table of data to the console, which can be useful for visualizing arrays or objects.
Here’s an example of console.table()
method:
const data = [ {name: "Winnie", age: 25}, {name: "Highline", age: 30}, {name: "Juliet", age: 20} ]; console.table(data);
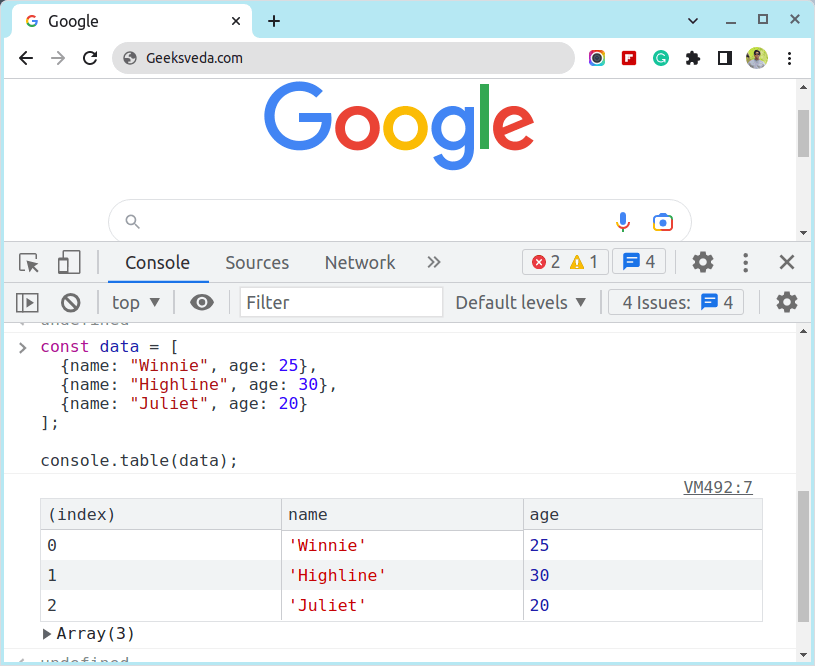
When you run the code for a console.table()
, you will see a formatted data table in the console, making it easier to read and analyze.
6 Using JavaScript innerHTML Method
The innerHTML property allows you to set the HTML content of an element on the page.
Here’s an example of innerHTML
method:
let message = "Welcome Home!"; document.getElementById("myDivOne").innerHTML = message;
In this code, we’re setting the innerHTML property of an element with the ID “myDivOne” to the message “Welcome!“. Executing the above utility will display the message “Welcome Home!” on the page in the element with the specified ID.
Conclusion
Printing a custom message in JavaScript is a fundamental programming task that is useful in many contexts. Whether you’re building a website, a web application, or just experimenting with JavaScript, knowing how to print custom messages is essential.
By using the different methods we’ve explored in this article, you can make your JavaScript code more dynamic, interactive, and engaging for your users.