The “add two numbers” program is a fundamental concept in programming, including JavaScript. It’s a basic operation frequently used in many applications and an essential building block for more complex programs.
JavaScript is widely used to develop web applications, games, and server-side applications. In each of these areas, there are many different use cases for adding two numbers, such as calculating the dimensions of an image or calculating the sum of values in a data set.
The JavaScript program to add two numbers is essential because it is one of the fundamental programs every beginner comes across when learning JavaScript.
The following methods can help you add two numbers in JavaScript.
1. Using JavaScript Addition + Operator
The first, and arguably the most common method is to use the addition (+
) operator as shown below.
let num1 = 5; let num2 = 10; let sum = num1 + num2; console.log("The sum of " + num1 + " and " + num2 + " is " + sum);
The +
operator directly adds the numbers to provide their sum.
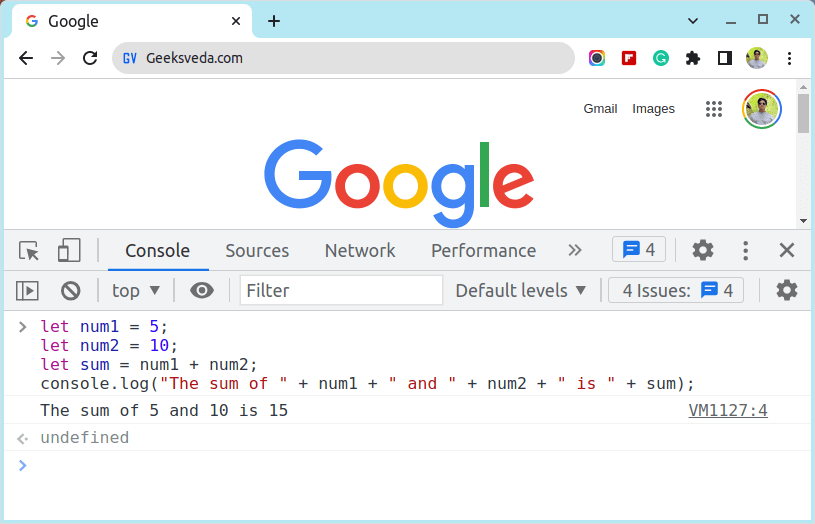
2. Using JavaScript Variables and + Operator
In the below example, we begin by defining two variables (num1 and num2) and assigning them the values of 7 and 10, respectively.
We then use the "+"
operator to add num1 and num2 and store the result in a variable named sum. Finally, we log the value of the sum to the console, which should output 17.
const num1 = 7; const num2 = 10; const sum = num1 + num2; console.log(sum);
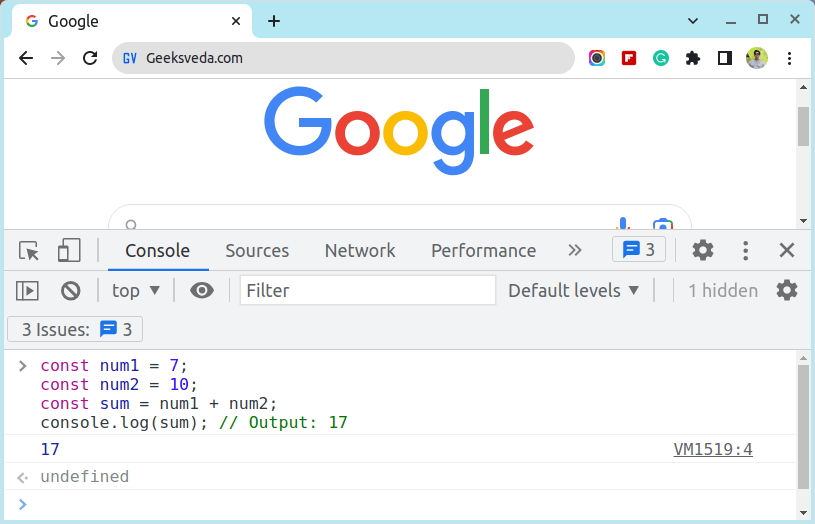
3. Using JavaScript Number() Function
In the below example, we first convert the strings “7” and “10” to numbers using either the Number()
function.
We then use the "+"
operator to add the two numbers and store the result in a variable named sum. Finally, we log the value of the sum to the console, which should output 17.
const num1 = "7"; const num2 = "10"; const sum = Number(num1) + Number(num2); console.log(sum);
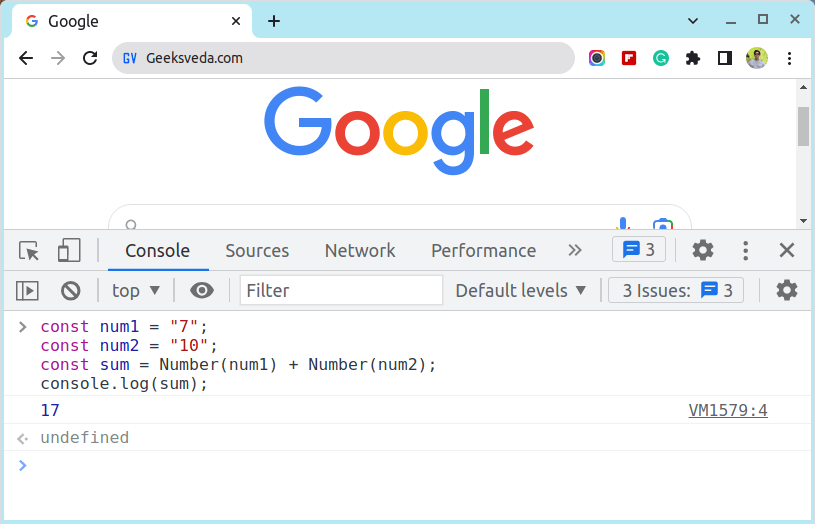
4. Using JavaScript parseFloat() Function
Finally, we can use the parseFloat()
function to add two numbers, as shown below.
const num1 = "7"; const num2 = "10"; const sum = parseFloat(num1) + parseFloat(num2); console.log(sum);
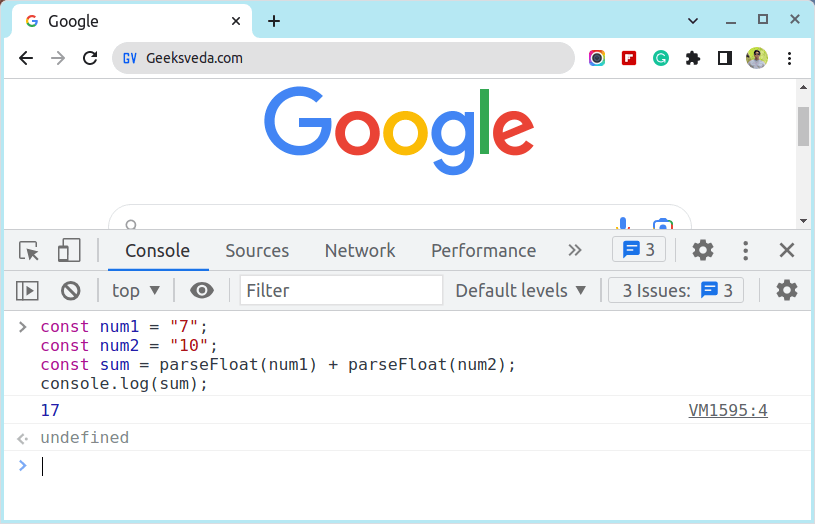
Just like with the Number()
function, we’re converting the strings “7” and “10” to numbers using the parseFloat()
function. We then use the "+"
operator to add the two numbers together and store the result in a variable named sum. Finally, we log the value of the sum to the console, which should output 17.
Note that we can only add two numbers already in numeric format. If you’re adding numbers that are stored as strings (e.g., “7” and “10”), you’ll need to convert them to numbers first before adding them together.
Conclusion
The JavaScript program is important because it teaches the basics of data types, such as the Number data type, which represents numeric data. Additionally, the program demonstrates the use of various JavaScript programming topics, such as variables, constants, and operators.
The program can be implemented in different ways, such as using HTML input fields to get user input, using the prompt()
function to get user input, or use predefined values to add two numbers.
Please tell us in the comment box if you have any methods we can explore.