Like the “add two numbers” program, subtracting two numbers is a fundamental concept in programming and is frequently used in various applications.
You will find it useful in web development, game development, data analysis, and virtualization. The importance of this program lies in its simplicity and usefulness as a building block for more complex programs.
Subtraction is a fundamental mathematical operation, and being able to perform it in a program is a basic programming skill. Many more complex programs rely on the ability to perform simple arithmetic operations like subtraction, so understanding how to write a program to subtract two numbers is an important foundational skill for any programmer.
Additionally, this program can be easily modified to perform other arithmetic operations, such as addition, multiplication, or division, by simply changing the operator used in the “subtract” function.
This article outlines some methods you can use to subtract two numbers in JavaScript.
1. Using JavaScript Subtraction – Operator
The simplest method of subtracting two numbers in JavaScript is by using subtraction ("-"
) operator.
let result = 10 - 3; console.log(result);
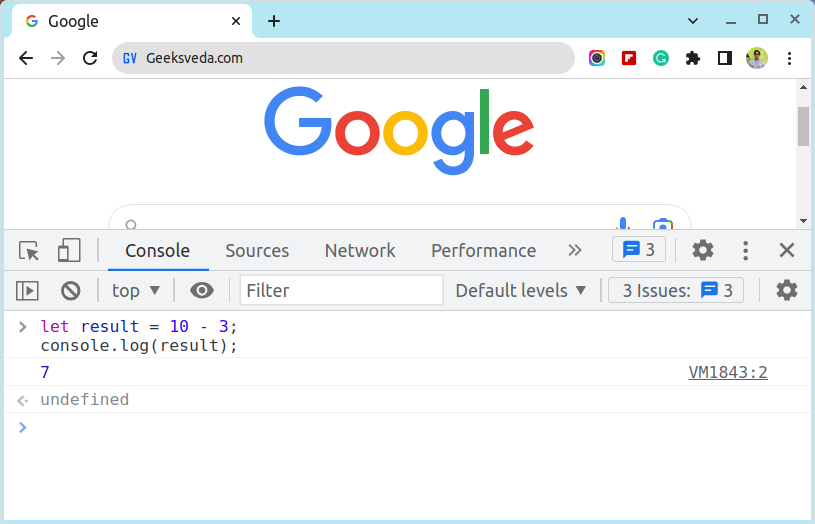
2. Using JavaScript Variables and – Operator
Instead of using literal values as in the above method, you can also use variables alongside the "-"
operator, as you can see in the code snippets below.
const num1 = 10; const num2 = 5; const difference = num1 - num2; console.log(difference);
In this example, we’re defining two variables (num1 and num2) and assigning them the values of 10 and 5, respectively.
We then use the "-"
operator to subtract num2 from num1 and store the result in a variable named difference. Finally, we log the value of the difference to the console, which should output 5.
The following code also demonstrates how you can use variables.
let num1 = 10; let num2 = 3; let result = num1 - num2; console.log(result);
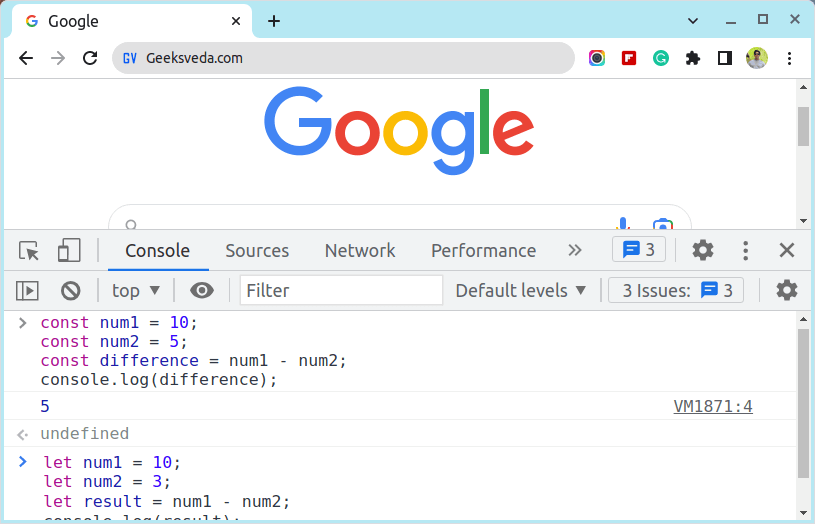
3. Subtract Number Using JavaScript Number() Function
You can also use the Number()
function to subtract two numbers, as shown below.
const num1 = "10"; const num2 = "5"; const difference = Number(num1) - Number(num2); console.log(difference);
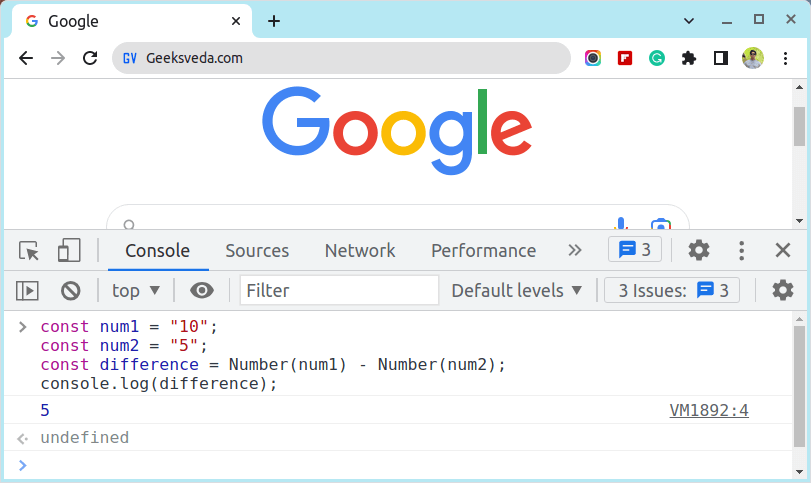
4. Subtract Number Using JavaScript parseFloat() Function
You can also use the parseFloat()
function to subtract two numbers.
const num1 = "10"; const num2 = "5"; const difference = parseFloat(num1) - parseFloat(num2); console.log(difference);
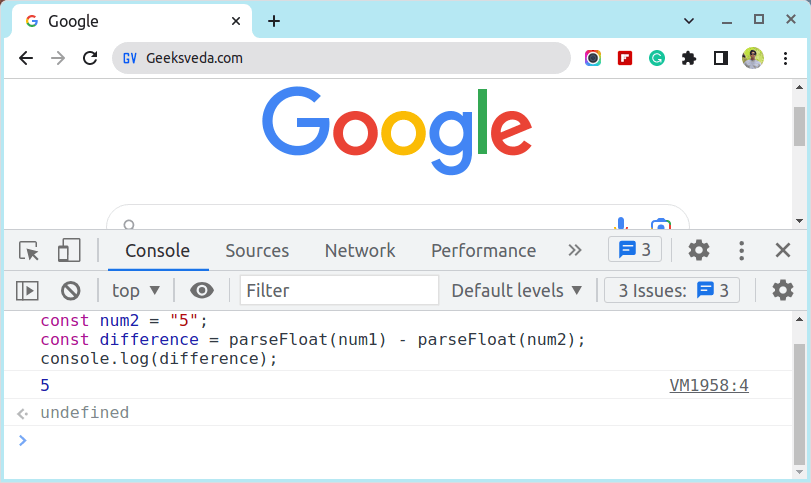
5. Subtract Number Using JavaScript Template Literal
A template literal is a way of embedding expressions inside a string literal in JavaScript.
let num1 = 10; let num2 = 3; let result = `${num1 - num2}`; console.log(result);
Note that we wrap the subtraction expression inside '${}'
in the template literal to evaluate it and include the result in the string.
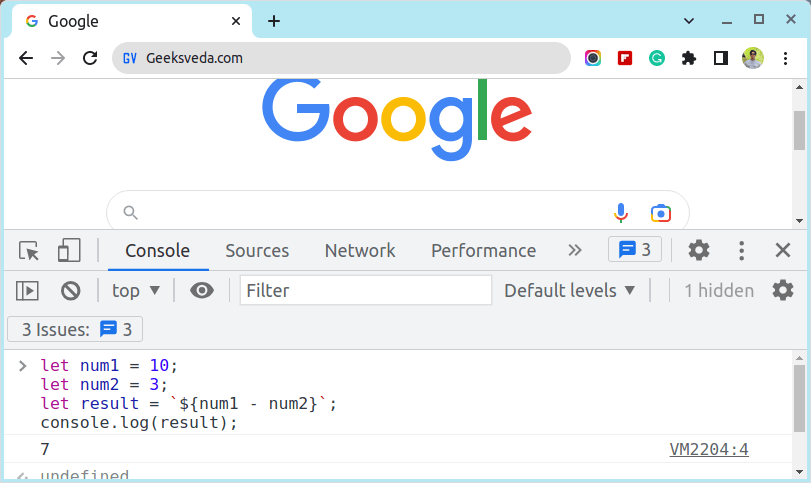
6. Using JavaScript reduce() Method
The reduce()
method is a built-in JavaScript method that can be used to perform a variety of operations on arrays.
let numbers = [10, 3]; let result = numbers.reduce((acc, curr) => acc - curr); console.log(result);
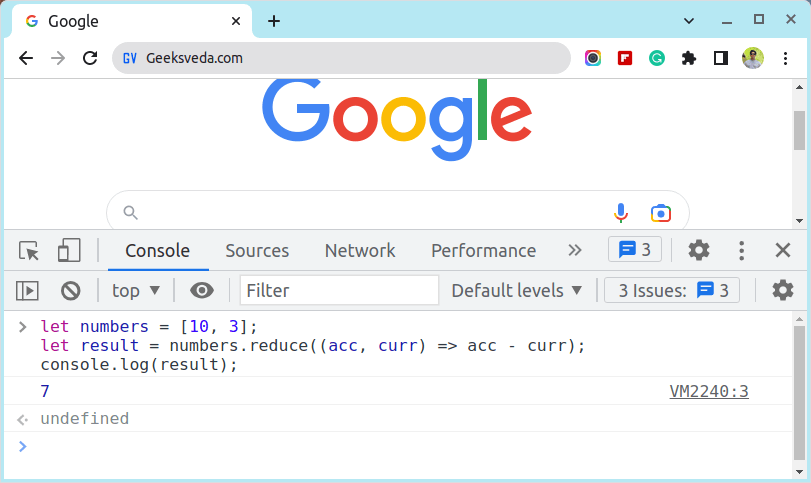
Here, we create an array of the two numbers we want to subtract, then call reduce()
on that array. The reduce()
method works successfully by taking a callback function that you will need to call for each element in the array.
The callback function takes two arguments: the accumulator (initially set to the first element in the array) and the current element. In this case, we subtract the current element from the accumulator and return the result. After reduce()
has iterated over all the elements in the array, it returns the final value of the accumulator, which is the result of the subtraction.
Conclusion
Each method accomplishes the same thing: subtracting two numbers in JavaScript. The choice of which method to use depends on the specific needs of your application and personal preference.
Do you have a different method worth sharing with us? Let us see it in the comment box below.