The “Hello World” program is a basic introductory program often used as a starting point for learning a new programming language. In JavaScript, the “Hello World” program simply prints the text “Hello, World!” to your screen.
The “Hello World” program is necessary as it helps programmers learn the basic syntax of a language and how to write a simple program that can produce output. It helps to verify that the development environment is set up correctly. Besides, you can use it to check that the programming language is working as expected.
See example:
console.log("Hello, World!");
This program outputs “Hello, World!” to your JavaScript Developer Console, which can be accessed through the Chrome browser’s Developer Tools window.
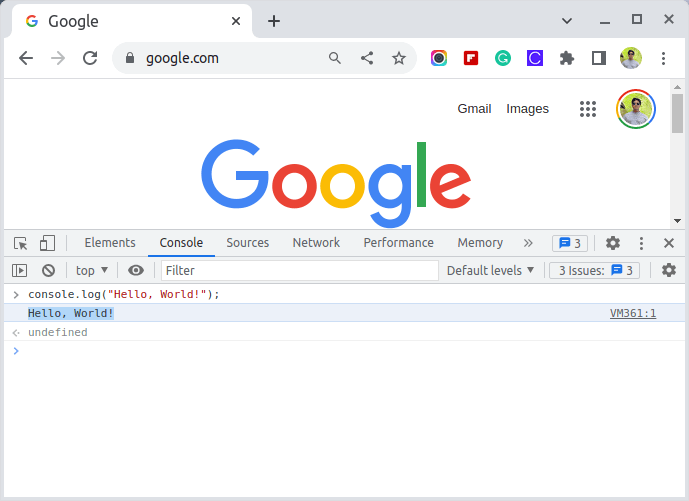
Alternatively, the command-line interface is an option through which you can execute a JavaScript program, but you must have Node.Js installed on the system.
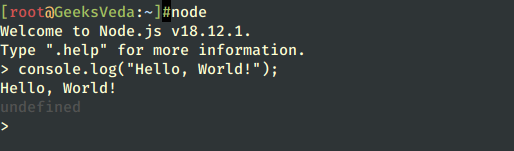
To write a “Hello World” program in JavaScript, you should understand the language’s syntax, variables, and data types. You should also be familiar with executing JavaScript code through a web browser or a command-line interface.
There are several ways to print “Hello, World!” in JavaScript.
1. Using console.log() Method
One way is to use the console.log()
method, as shown in the example above. The code will look like this;
console.log('Hello World');
The program uses the console.log()
method to print the “Hello, World!” message to your console. It is the most common way to print output in JavaScript.
This method can take one or more before logging them into the console. In this case, you will log the “Hello, World!” string to your console.
2. Using the alert() Method
Another way is to use the alert()
method to display a pop-up window with the message:
alert("Hello, World!");
The program uses the alert()
method to display a box containing “Hello, World!“. The method is ideal for displaying messages to the user through a pop-up box.
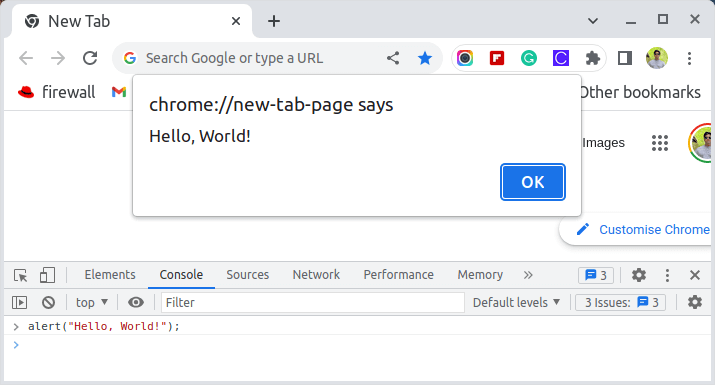
3. Using the document.write() Method
Alternatively, you can write the message directly to the HTML document using the document.write() method.
document.write("Hello, World!");
The program uses the document.write()
method to write the message “Hello, World!” to the HTML document. The document.write()
method adds dynamic content to a web page, which can be useful for creating simple interactive programs.
However, it is not recommended for production code as it can cause page layout and performance issues.
4. Using the document.getElementById() Method
Finally, you can use the document.getElementById()
method to print Hello World.
document.getElementById("output").innerHTML = "Hello, World!";
The above code uses the document.getElementById()
method to get the HTML element with the ID “output“, and then sets its innerHTML property to the message “Hello, World!“. This method comes in handy in adding dynamic content to a web page.
Conclusion
The “Hello World” program in JavaScript is a simple but important starting point for learning the language and developing more complex applications. Each of these methods has its own significance and use case.
The console.log()
is the most commonly used method for printing output to the console. alert()
is useful for displaying messages to the user in a pop-up box. document.write()
and document.getElementById().innerHTML
are both useful for adding dynamic content to a web page.
It’s important to choose the method that best fits the situation.