To find the maximum of two numbers in a JavaScript program is a fundamental mathematical operation and an essential component of programming, which takes two numbers as input and returns the maximum or greater of the two.
This introductory program comes in handy in several programming languages, including sorting algorithms, finding the most significant or smallest number in a dataset, and determining the winner of a game. It is crucial to understand how to find the maximum of two numbers in programming and to know various methods to achieve it efficiently.
To learn how to write a program, one must understand basic JavaScript syntax and programming concepts such as conditional statements and functions. Additionally, knowledge of basic mathematical operators (such as greater than, less than, and equals) would be helpful.
By developing these skills, you will quickly learn how to write a JavaScript program to get the maximum of two numbers using any of the several methods available.
1. Using JavaScript Math.max() Method
The first method is by using the Math.max()
as shown:
function findMaxUsingMathMax(a, b) { return Math.max(a, b); } // Generate output with any two numbers console.log(Math.max(a, b));
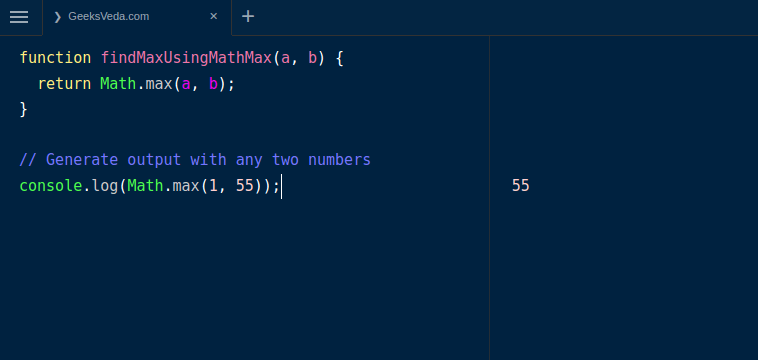
This method uses the Math.max()
method to find the maximum of the two numbers. It takes in two arguments and returns the larger of the two.
2. Using JavaScript Conditional Statement
An example of how to get the maximum of two numbers using a conditional statement is as shown:
function findMaxUsingConditional(a, b) { if (a > b) { return a; } else { return b; } } // Generate output with any two numbers console.log(findMaxUsingConditional(a, b));
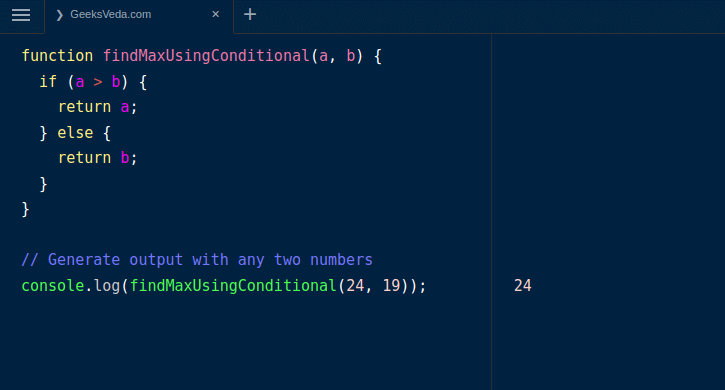
This method uses a conditional statement to check which of the two numbers is larger. If the first number has a greater numerical value than the second number, the output will be the first number. Otherwise, it returns the second number.
3. Using JavaScript Ternary Operator
Lastly, you can use the ternary operator method to find the largest of two numbers, as shown in the code example;
function findMaxUsingTernary(a, b) { return a > b ? a : b; } // Generate output with any two numbers console.log(findMaxUsingTernary(a, b));
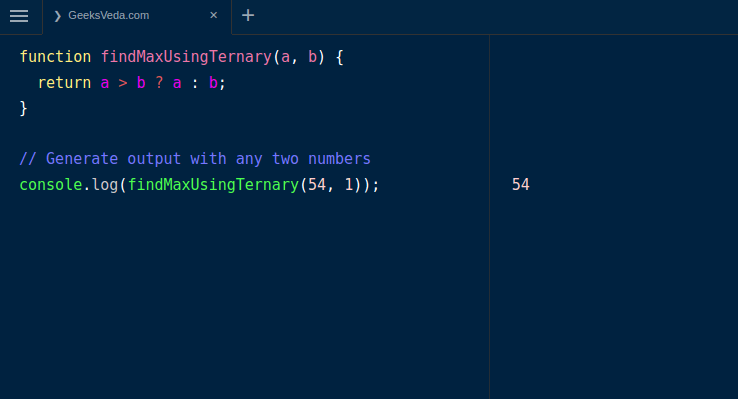
This method uses the ternary operator to check which of the two numbers is larger. If the first number is more significant numerically than the second number, it returns the first number. Otherwise, it returns the second number.
4. Using JavaScript Sort() Method
You can use the sort method as follows:
function findMax(num1, num2) { return [num1, num2].sort((a, b) => b - a)[0]; } // Generate output with any two numbers console.log(findMax(a, b));
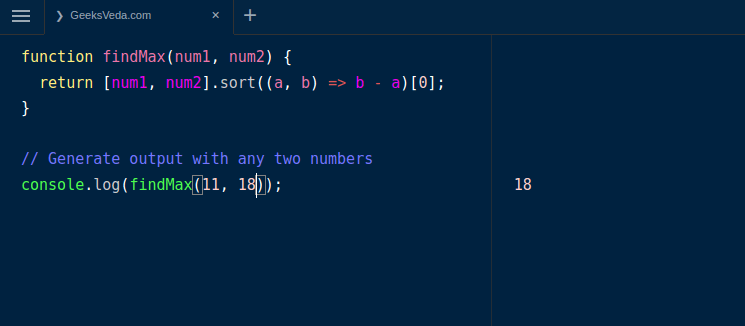
This code defines a function called findMax that takes two parameters, num1, and num2. The function creates an array containing these two numbers, then calls the sort()
method on the array.
The sort()
method sorts the numbers in descending order (i.e., from largest to smallest) using a comparison function that subtracts b from a. Finally, the function returns the first element of the sorted array, which is the largest number.
The sort()
method can be useful in certain situations where you must find the maximum of more than two numbers. You can simply pass an array of numbers to the function instead of just two numbers, and the function will return the largest number in the array.
However, the previous three methods are more efficient and easier to read and implement for just two numbers.
Conclusion
In this article, we explored four different methods: the Math.max()
function, a conditional statement, the ternary operator, and the sort()
method on an array.
While all of these methods will give the same result, each has its advantages and disadvantages depending on the context of the problem you are trying to solve. Which method would you choose and why?