Measuring the performance of code is an important aspect of optimizing algorithms and functions for faster and more efficient execution. It is particularly crucial when working on data analysis, machine learning, or other computational tasks in R.
Accurately assessing the execution time of your code is essential to achieving optimal results. This article aims to help simplify this process by exploring various methods for measuring the execution time of a function in R.
Before moving towards, you must install R on your system.
There are multiple methods to measure the execution time of a function in R, which are as follows:
Let’s discuss these methods in detail in the following section.
1. Using system.time() Function
The system.time()
is a built-in function in R that allows users to measure the execution time of functions. When called on a specific expression or function, it returns an object of class “proc_time“, which provides important information about the user CPU time, system CPU time, elapsed real time, and other system-specific values.
Using system.time()
is easy; you just need to put the expression or function you want to time inside the system.time()
function. As the expression or function runs, system.time()
records the CPU and elapsed time, and returns a “proc_time” object containing information, which can be particularly useful in identifying performance bottlenecks and optimizing your code to achieve better results.
The following example shows the implementation of the system.time() function in R.
# Define a loop to measure execution time n <- 1000 sum <- 0 system.time({ for (i in 1:n) { sum <- sum + i } })
The above code defines a loop that iterates from 1 to 1000 and calculates the sum of the first 1000 positive integers. It uses the system.time()
to measure the execution time of the loop and outputs the CPU and elapsed time taken to execute the loop.
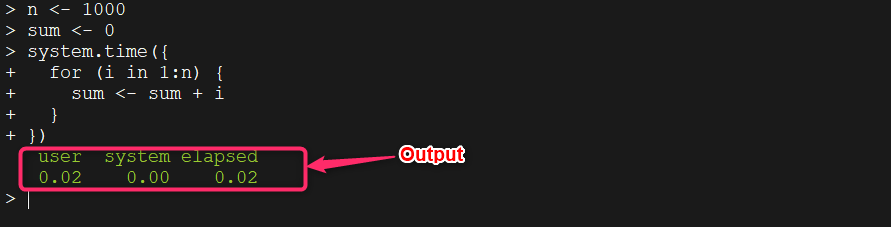
2. Using proc.time() Function
The proc.time()
is another built-in function in R that allows you to measure the execution time of the function. When you use proc.time()
, it returns a vector containing information about the amount of CPU and system time used by the R process.
To use this function, you must first record the time at the beginning of your code block, and then record the time at the end of the code block. After that, you can subtract the start time from the end time to get the total time taken for the code block to run.
Here is a simple example that shows the use of proc.time()
function in R.
# Define a loop to measure execution time n <- 100 factorial <- 1 start_time <- proc.time() # record start time for (i in 1:n) { factorial <- factorial * i } end_time <- proc.time() # record end time total_time <- end_time - start_time # calculate total execution time print(paste0("The factorial of ", n, " is ", factorial)) print(paste0("Total execution time: ", total_time["elapsed"], " seconds"))
The above program uses a loop to calculate the factorial of a number and measures the execution time using the proc.time()
function. The start and end times of the loop are recorded, and the difference between them is used to calculate the total execution time, which is printed to the console along with the calculated factorial value.
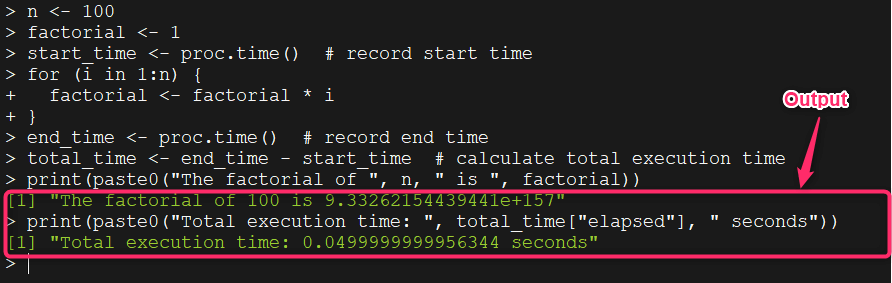
3. Using microbenchmark Package
The microbenchmark package in R provides a simple and accurate way to measure the execution time of functions. With this package, you can easily compare the performance of different functions or code snippets, and obtain information on the minimum, median, and maximum execution times, along with other statistical measures.
It can be particularly useful when working with small code segments and can assist you in optimizing your code for faster performance. However, to use this package, you must first install it on your R compiler with the following code.
install.packages("microbenchmark")
The following example shows the use of the microbenchmark package in R.
library(microbenchmark) # Define a function to benchmark my_function <- function(x, y) { z <- x + y return(z) } # Generate some input data x <- runif(1000) y <- runif(1000) # Run the benchmark results <- microbenchmark(my_function(x, y)) # Print the results print(results)
The above code uses the benchmark library to measure the performance execution time of my_function
using randomly generated input data. The results variable stores the benchmark results and the print function displays them on the terminal.
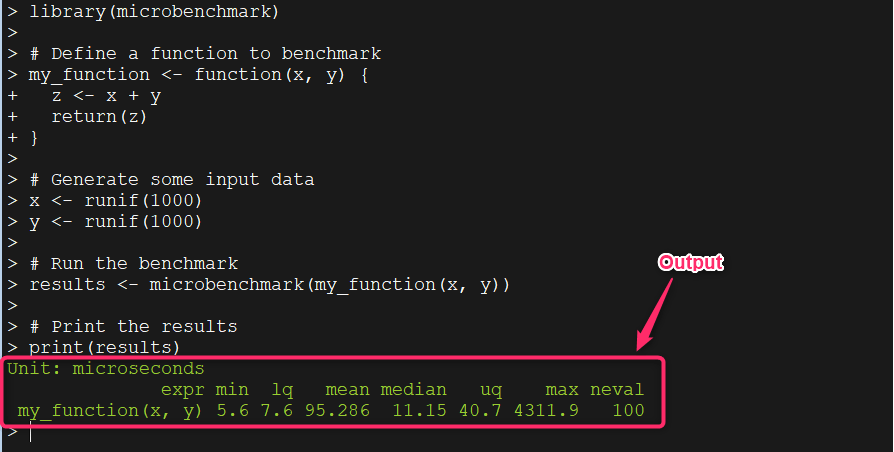
4. Using tictoc Package
The tictoc is a package used in most programming languages for measuring execution time. By using this package in R, the users will be able to measure the execution time of a function.
You must install the tictoc package on R before using it in your code. The following command will install the tictoc package on your R compiler.
install.packages("tictoc")
The below-given example shows the implementation of the tictoc package in R.
library(tictoc) tic() for (i in 1:1000) { x <- runif(1) } toc()
The above code uses the tic()
function to start a timer, followed by a for loop that iterates 1000 times, generating a random number in each iteration. Finally, it uses the toc()
function to stop the timer and display the elapsed time.
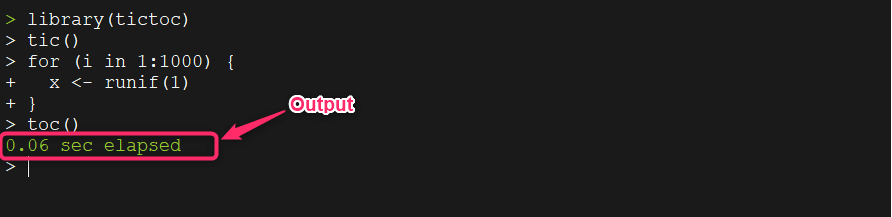
Conclusion
This article discusses four methods for measuring the execution time of a function in R, namely system.time()
, proc.time()
, microbenchmark, and tictoc package.
The system.time()
and proc.time()
functions are built-in functions in R that enable users to measure the CPU and elapsed time of their code.
The microbenchmark package is particularly helpful when working with small code segments, and provides statistical measures of execution time. The tictoc package is a commonly used function that can measure the execution time of loops and sections of code.
Do you have any other methods to measure the execution time of a function in R? you would want us to include in this article? Please share it in the comment box below.