In the world of Python programming, metaclasses play a significant role in customizing class creation and extending the language’s capabilities. By understanding the concepts of OOP, Python classes and objects, you can explore the power of metaclasses.
Today’s guide will briefly discuss Python metaclasses, the method to create custom metaclasses, and their difference from simple classes. Moreover, we will also discuss the process of creating singleton classes and the procedure to perform class-level validation and attribute management in Python.
What are Python Classes
Object-Oriented Programming (OOP) is a programming paradigm that is utilized for organizing the code into objects, which are known as instances of classes.
More specifically, classes are the blueprint for creating objects in OOP. They are used for defining the behavior and structure of objects by defining the methods and their relevant attributes. These objects can hold unique data and have the ability to define methods.
What are Metaclasses in Python
In Python, Metaclasses are defined as the “classes of classes
” as they define the classes’ behavior. These types of classes permit the customization of class creation by modifying and intercepting the class creation process. Moreover, they also enable you to control several aspects of class creation, such as adding methods, modifying attributes, or enforcing particular behaviors.
Moreover, metaclasses empower developers for implementing advanced frameworks and patterns that go beyond the capabilities of standard class creation.
Difference Between Simple Class and Metaclass in Python
Check out the following table comprising the differences between simple class and metaclass in Python.
Simple Class | Metaclass | |
Definition | Defines the behavior and structure of objects | Defines the behavior of classes |
Inherits From | Object class | Type class or another metaclass |
Instance Creation | Creates instances of the class | Creates classes (which can then create instances) |
Special Syntax | No special syntax | Utilizes metaclasses attribute in the class definition |
Modifications | Modifies methods and attributes of instances | Modifies behavior and attributes of classes |
Customization | Limited customization options | Permits extensive customization of class creation |
Application | Used for creating objects and defining behaviors | Used for customizing class creation and behavior |
How to Create Custom Metaclasses in Python
In Python, the “type
” metaclass is the default metaclass. It can be utilized for creating custom metaclasses. To do so, follow the provided steps.
- First of all, define a class that has a “
type
” subclass or another metaclass. - Then, override the required methods like “
__init__
” or “__new__
” for customizing the class creation process. - Lastly, use the custom metaclass by specifying the created metaclass as the argument in the class definition.
For instance, we have defined the “MyMeta
” metaclass by subclassing “type
“. Moreover, the “__new__
” method is overridden for customizing the class creation operation.
class MyMeta(type): def __new__(cls, name, bases, attrs): return super().__new__(cls, name, bases, attrs) class MyClass(metaclass=MyMeta): pass obj = MyClass()
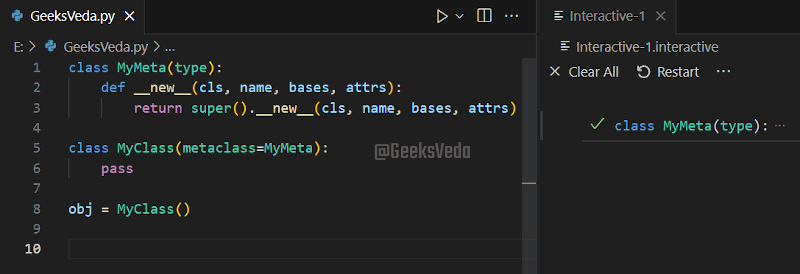
How to Create a Singleton Class in Python
Python Singleton classes permit only one instance to be created throughout the execution of the program. These classes are primarily utilized for making sure that there is a single point of access to a globally available object or shared resource. Moreover, you can also use it when it is needed to restrict the class instantiation to a single object.
Metaclasses also assist in creating singleton classes from it. Like, here, in the given example, we will create a singleton class from a custom metaclass. The “Metaclass
” will keep records of the instance which are going to be created from the associated class.
Note that the “__call__
” method is overridden for making sure that only one instance is created. The “SimpleClass
” is a singleton class that utilizes the MetaClass. Therefore, creating the objects of the SimpleClass will output the same instance.
class MetaClass(type): _instances = {} def __call__(cls, *args, **kwargs): if cls not in cls._instances: cls._instances[cls] = super().__call__(*args, **kwargs) return cls._instances[cls] class SimpleClass(metaclass=MetaClass): pass obj1 = SimpleClass() obj2 = SimpleClass() print(obj1 is obj2)
As a result, the given condition “obj1 is obj2
” prints True.
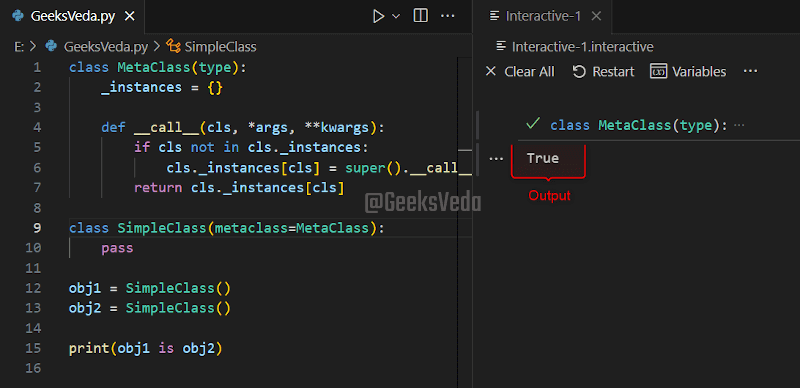
Implement Class-Level Validation and Attribute Management in Python
Class-level validation and attribute management are essential for ensuring consistency and data integrity within classes. They assist in enforcing particular requirements or rules on attributes and provide a mechanism for validating input values or performing additional checks at the class level. This results in more maintainable and reliable code.
The given program implements a class-level validation with the help of utilizing a custom metaclass named “ValidationMeta
“. It overrides the “__new__
” method for checking if the “name
” attribute exists in the class definition.
If the attribute is missing, an “AttributeError
” will be raised.
class ValidationMeta(type): def __new__(cls, name, bases, attrs): if 'name' not in attrs: raise AttributeError(f"'{name}' class must have a 'name' attribute.") return super().__new__(cls, name, bases, attrs) class MyClass(metaclass=ValidationMeta): name = 'My Class' class InvalidClass(metaclass=ValidationMeta): pass
When we create an instance of “MyClass
“, and define a “name
” attribute, it will be created successfully. However, creating an instance of “InvalidClass
” without the mentioned attribute will throw an “AttributeError
” during the class creation.
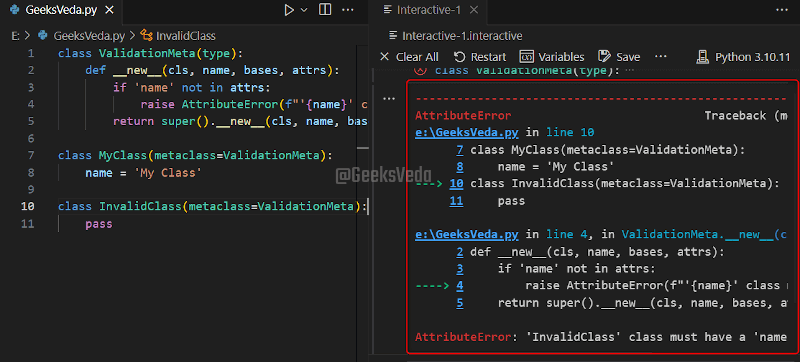
Best Practices for Creating Metaclasses in Python
Here are some of the best practices for creating Metaclasses in Python.
- Give meaningful names to metaclasses and document their purpose.
- Utilize metaclasses sparingly and only when required.
- Keep metaclasses implementation straightforward and avoid unnecessary complexity.
- Test metaclasses thoroughly to make sure that they work correctly.
- Consider alternative approaches like composition when possible.
Conclusion
In Python, metaclasses offer extensibility and flexibility for customizing class creation. By utilizing them and following the enlisted best practices, developers can create robust frameworks and enforce coding conventions.
However, keep in mind the impact of metaclasses on your code and use them sparingly. If these classes are handled well, they can be proved as a valuable asset in Python development.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!