Whether you are manipulating data, updating formatting, or correcting typos, string replacement is a common operation that you may need to perform in your JavaScript code.
While the JavaScript replace()
method is the default approach for replacing a single instance of a string, what if you need to replace all instances of a string? It can be done by utilizing the regular expressions. However, there also exist some other techniques for it.
In today’s blog, we will explore several techniques to replace all occurrences of a string in JavaScript, including regular expression. Moreover, the relevant limitations and the points related to overall performance consideration will also be a part of this guide.
1. Using split() and join() in JavaScript
While thinking about replacing all occurrences in JavaScript, the first approach that comes to one’s mind could be the usage of the split()
and join()
methods.
Using this approach, you can split the original string into an array based on the defined string. This can be done using the split()
method.
Then, the next step is to join the array elements with the required replacement string by utilizing the join()
method.
For instance, we will now use split()
and join()
methods for replacing all occurrences of the word Coding with Designing.
let str = "I love Coding and Coding is my life"; let newArray = str.split("Coding"); let replacedString = newArray.join("Designing"); console.log(replacedString);
It can be observed that we have successfully replaced all occurrences of the word Coding with Designing.
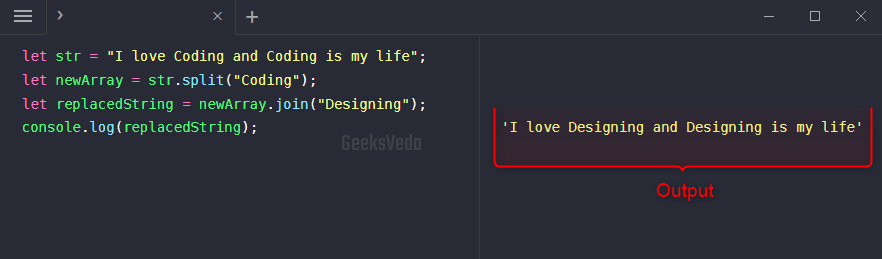
Limitations of split() and join() Methods
This approach works perfectly for replacing all occurrences of the defined string. However, it also has some limitations that are enlisted below.
- The
split()
andjoin()
method approach is preferable when it is required to replace the exact match of the string. It does not offer support for regular expressions or more advanced pattern matching. - This approach does not provide more options for manipulation of the replacement string.
- As the
split()
andjoin()
methods split the original string into an array first and then join the array element according to the defined pattern or string. Resultantly, this causes performance issues and unnecessary memory consumption. So, in such scenarios, the replace() method offers better performance.
2. Using JavaScript String replace()
In JavaScript, the replace()
built-in method is utilized for replacing a particular string occurrence of a substring in a string.
To use the mentioned method, follow the provided syntax.
str1.replace("old_str", "new_str")
This method accepts two arguments.
- The first argument represents the old string “
old_str
” that needs to be replaced. - The second argument refers to the new string “
new_str
” that will replace only the first occurrence of the givenold_str
in the original string.
Now, let’s check out the usage of the replace()
method in a JavaScript program.
let str1 = "I love Coding"; let str2 = str1.replace("Coding", "Designing"); console.log(str2);
Here, the original string “str1
“, the first occurrence of the string Coding will be replaced with Designing.
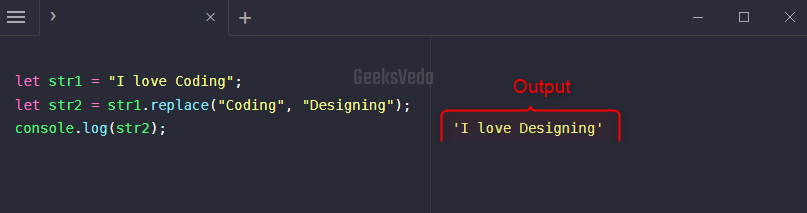
Limitation of the replace() Method
As observed in the previous example, the replace()
method has the capability to only replace the first occurrence of the specified string. Therefore, you cannot utilize this method solely for replacing all occurrences at once.
In case, if you want to do so, it is required to use either the regular expressions or the global flag.
For a clear understanding, we will discuss both in the upcoming sections.
3 Using Regular Expressions in JavaScript
A pattern or expression that is defined to match specific characters set in a string is called a Regular Expression. In a program, you can create the JavaScript regular expression with the help of the “RegExp
” object.
These expressions are primarily utilized for finding and replacing the given character’s pattern in the string.
In this example, the “/Coding/g
” regular expression is added as the first argument of the replace()
method.
let str1 = "I love Coding and Coding is my life"; let str2= str1.replace(/Coding/g, "Designing"); console.log(str2);
As a result, the replace()
method will match the specific expression with all of the “Coding
” string occurrences and replaces them with “Designing
” as follows.
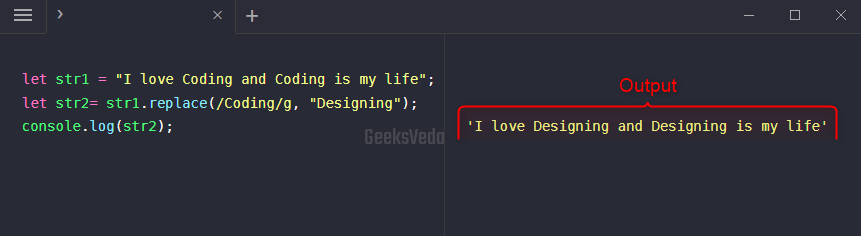
Different Flags With Their Explanation
Regular expression supports different flags that can be added to perform the required functionality. For instance.
- The “
g
” flag represents the global search. - The “
m
” flag indicates the multi-line search. - The “
i
” flag refers to the case-insensitive search. - The “
u
” flag represents the Unicode support.
Creating Regular Expression Using RegExp
You can also create a regular expression by utilizing the RegExp()
constructor. It enables you to define a dynamic regex or matching pattern as per your requirements.
For instance, continuing the same example, we will now replace the word Coding with Designing in the created string. More specifically, the regular expression will be created with RegExp
as follows.
let str = "I love Coding"; let regexPattern = new RegExp("Coding"); let replacedStr = str.replace(regexPattern, "Designing"); console.log(replacedStr);
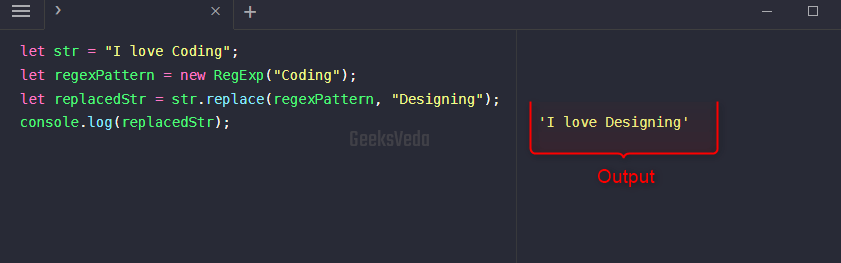
Using Global Regular Expressions in JavaScript
As discussed earlier, the global flag “g
” is added in the regular expression for performing the global search and replace operation. This flag signifies that the replace()
method will now have to replace the string for all occurrences.
In this example, we have added “/the/g
” as the regular expression. This expression will search for all of the “the
” string occurrences because of the added global flag “g
” and then replaces them with “a
“.
let str1 = "the robber hid the gun in the planter nearest the left corner"; let str2= str1.replace(/the/g, "a"); console.log(str2);
It can be observed that all four occurrences of “the
” have been replaced with “a
“.
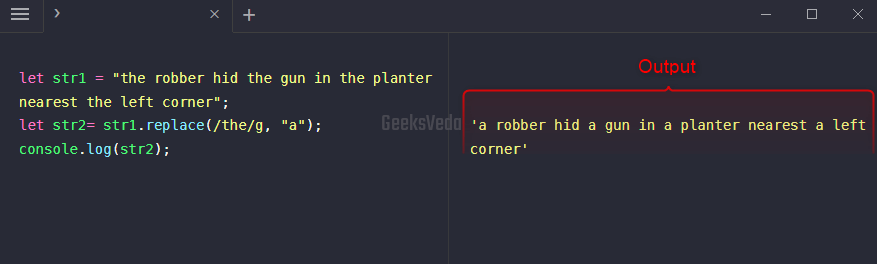
4 Using replaceAll() Method in JavaScript
To get rid of the hassle of defining a regular expression
and adding a flag
to it for the specified operation, ECMAScript 2021 or ES2021 introduced the replaceAll()
method.
This method can be utilized for the string replacement of all of the occurrences at once.
Here is the syntax followed to use it.
str1.replaceAll("old_str", "new_str")
According to the provided syntax.
- The first argument signifies the old string “
old_str
” that needs to be replaced. - The second argument represents the new string “
new_str
” that will replace all occurrences of the given old_str in the original or old string.
Now, we have increased the length of the str1 to check out the working of the replaceAll()
method more clearly. In the given str1, currently, we have six “the
” strings that we want to replace with “a
“.
For the corresponding purpose, we have invoked the replaceAll()
method and passed both strings, respectively.
let str1 = "the robber hid the pistol in the planter nearest the left corner of the road to the store"; let str2= str1.replaceAll("the", "a"); console.log(str2);
As you can see, all of the occurrences of the specified string have been replaced successfully.
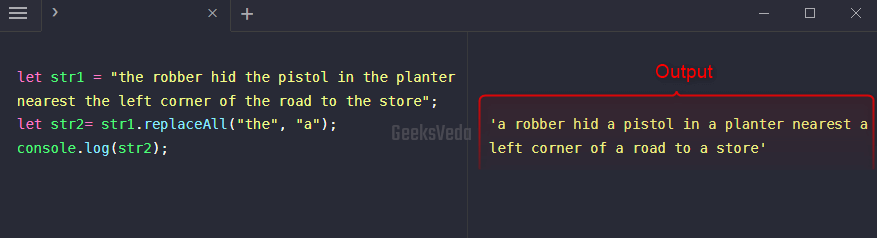
Difference Between replace() vs replaceAll() in JavaScript
Now, we will compare the replace()
and replaceAll()
methods based on their functionality and features.
Features | replace() Method | replaceAll() Method |
Introduced in ECMAScript | ECMAScript 1.0 (1997) | ECMAScript 2021 |
First Argument | Regular expression or String | Only String |
Only replaces the first occurrence | Yes (if a string is passed as the first argument) | No |
Replaces all occurrences | No (but can be done by using the global flag in the regular expression) | Yes |
Performance Consideration
Regular expressions are robust but can be proved as computationally expensive. For instance, utilizing regular expressions for string replacement operations can heavily impact the performance, specifically in cases where you have to deal with complex regular expressions or large strings.
Moreover, when the number of replacements is greater, it will take longer to execute.
Tips for Optimizing the String Replacement in JavaScript
Here, we have enlisted some of the tips that you can consider for optimizing string replacement in JavaScript.
- Use “
replace()
” for simple string replacement. - For replacing all occurrences, utilize the”
replaceAll()
” instead of regular expressions. This method is easier to read and more efficient as compared to regular expressions. - If you only want to use the
replace()
method, then add the global flag “g
” for replacing all strings at once, rather than one by one. - Optimize the regular expression pattern by avoiding complex patterns.
- Select the suitable method for string replacement based on the use case.
That’s all from this informative guide related to replacing all string occurrences.
Conclusion
One of the most frequent tasks in JavaScript development is replacing a string where it has been found.
Therefore, in today’s guides, we have explored different JavaScript replacement approaches, such as the replace() method, regular expressions, the global flag, and the replaceAll() method.
We have also covered the limitations of these techniques and the overall performance consideration. Now, you are all ready to choose the most appropriate approach for replacing all strings in the JavaScript code!