Printing without a newline or space in Python is a valuable approach that permits you to control the formatting of your output. So, whether you want to improve data readability, create visually appealing displays, or design interactive interfaces, mastering this technique is crucial.
Today’s guide will demonstrate multiple methods and code examples to help you print without a new line or space. Moreover, we will also discuss some essential troubleshooting tips and tricks.
Ways to Print Without a Newline or Space in Python
Python supports different techniques for printing without a new line or space. Some of these are.
end
parametersep
parameterwrite()
methodsys
module
The following subsections will thoroughly discuss each of the mentioned approaches.
Python end parameter in print()
In the print()function, the end
parameter is mostly utilized for specifying single or multiple characters that should be displayed at the end of the output.
By default, its value is set to “/n
“, which signifies that a new line will be printed out. But no worries! You can pass the empty string ""
for printing without a new line.
For instance, in the following example, the messages added in both of the print()
statements will be printed out on the same line without space.
This functionality is achieved by defining an empty string as the end parameter value.
print("Hello GeeksVeda User!", end="") print("How's it going?")
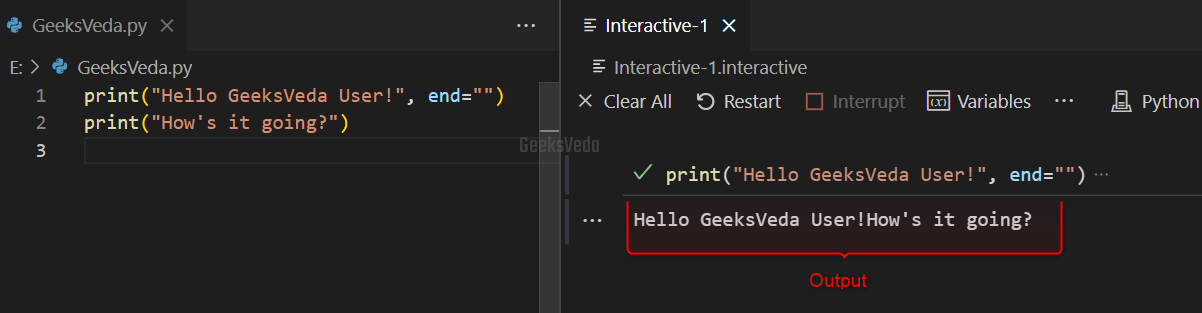
Python sep parameter in print()
Another useful parameter that Python supports is “sep
“. This parameter is added to the print()
function to specify the single or multiple characters that should be shown between the other passed arguments.
More specifically, the default value of the sep
parameter is set to single space " "
which is printed between the passed argument.
But, you can pass the empty string ""
for printing without a space.
Now, we will call only one print()
function, pass two arguments, and set the value of the sep
parameter as an empty string.
print("Hello,", "GeeksVeda User!", sep="")
Resultantly, the passed arguments will be printed on the same line without any space between them.
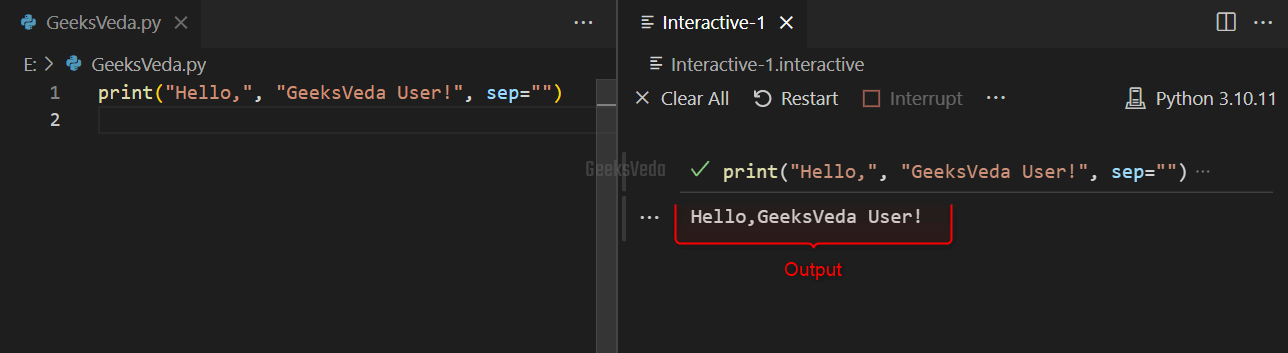
Python File write() Method
The “write()
” method of the file object permits you to write the data to the file. However, you can also utilize this method for writing data to the console (as standard output).
By default, the write()
method does not specify a space or new line. Therefore, it can be used for printing strings without a new line.
Here, we have called the write()
method two times, and both strings will be printed on the same line without any space.
import sys sys.stdout.write("Hello GeeksVeda User!") sys.stdout.write("How's it going?")
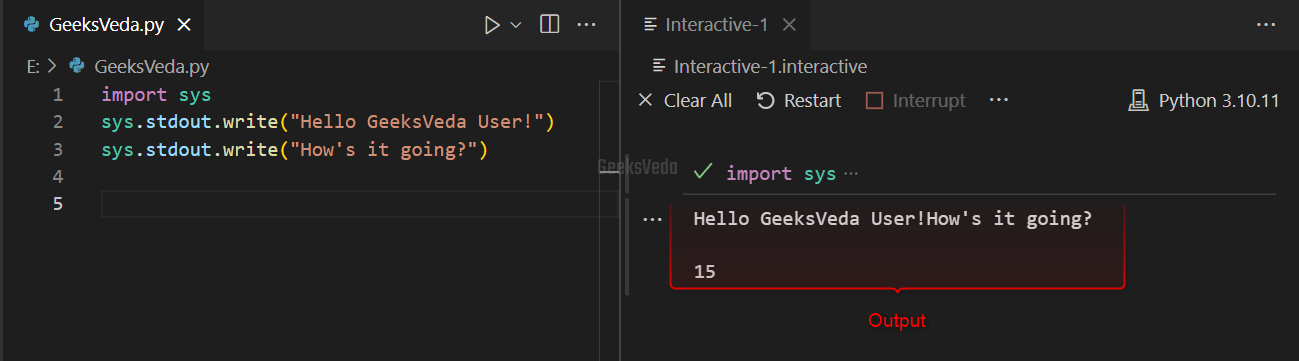
Python sys Module
Python’s built-in “sys
” module offers access to some variables maintained or utilized by the interpreter. However, it can be also used for manipulating the sys.stdout
or output stream and displaying it without the new line.
To use the sys
module, import it first into your Python project. Then, we will input the user name by utilizing the input()
function.
After that, the sys.stdout.write()
method will be called two times for showing the welcome message along with the user name on the same line.
import sys name = input("Enter you name ") sys.stdout.write("Hello " + name + "!") sys.stdout.write("Welcome to GeeksVeda")
Here, we have passed “Sharqa
” as the user name.
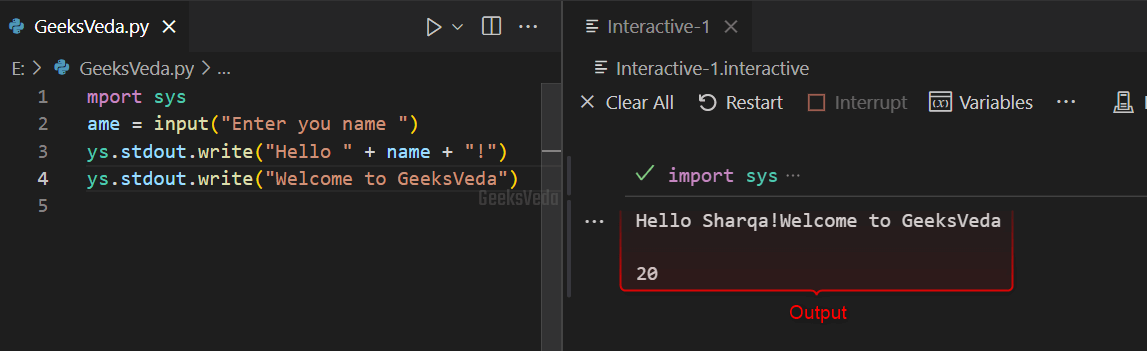
It can be observed that the username and the welcome message have been printed without a new line or space.
Advantages of Printing Without a Newline or Space
Check out the provided list of the advantages of printing without printing a new line or space in Python.
- Saves screen space.
- Enables dynamic and interactive user experiences.
- Offers more control over output formatting.
- Assist in improving the organization and reliability of the printed information.
- Useful for the custom console interfaces and progress indicators.
Common Errors in Python and How to Fix Them
This section will demonstrate some of the common errors that occur while printing a newline or space and their respective fixes.
SyntaxError: unterminated string literal in Python
Missing a double quotation after or before defining the string in the print()
function can throw the unterminated string literal error.
print("Hello GeeksVeda User! ", end="") print("How's it going?")
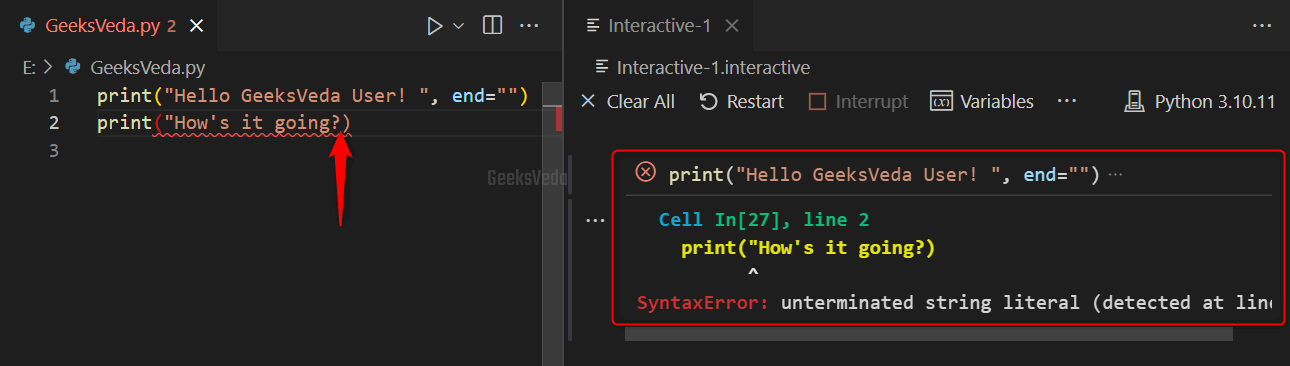
To resolve it, add the opening or closing double quotes which are missing in the print()
statement.
print("Hello GeeksVeda User! ", end="") print("How's it going?")
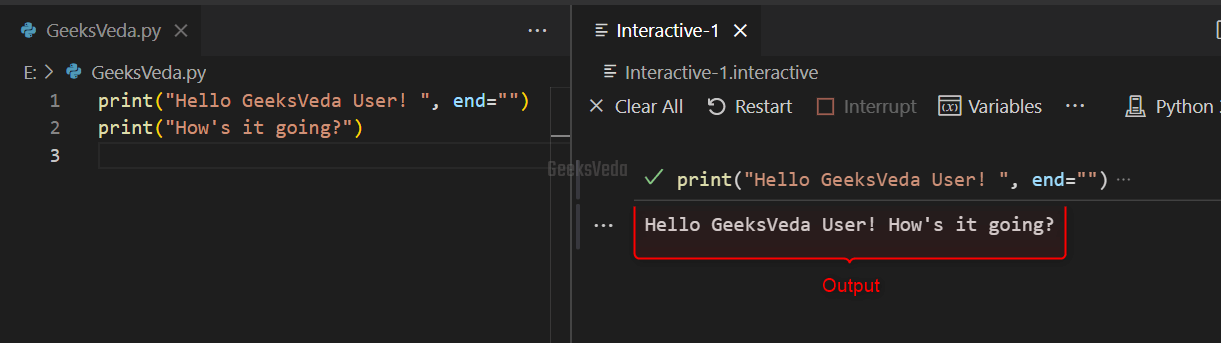
SyntaxError: incomplete input in Python
Another similar case is missing the opening or closing parentheses in the print()
statement. In such a scenario, the incomplete input syntax error will be displayed on the console.
print("Hello,", "GeeksVeda User!", sep=""
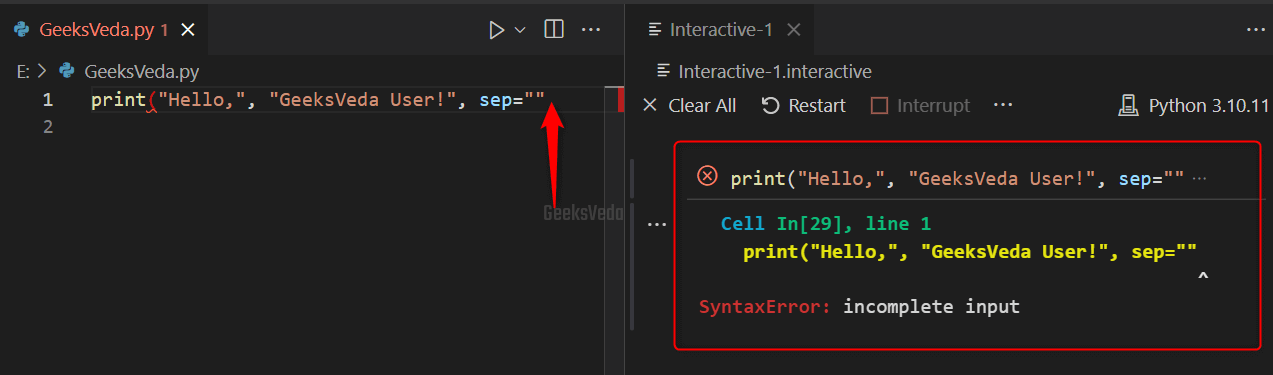
To resolve the mentioned error, add the required parentheses in the print()
function.
print("Hello,", "GeeksVeda User!", sep="")
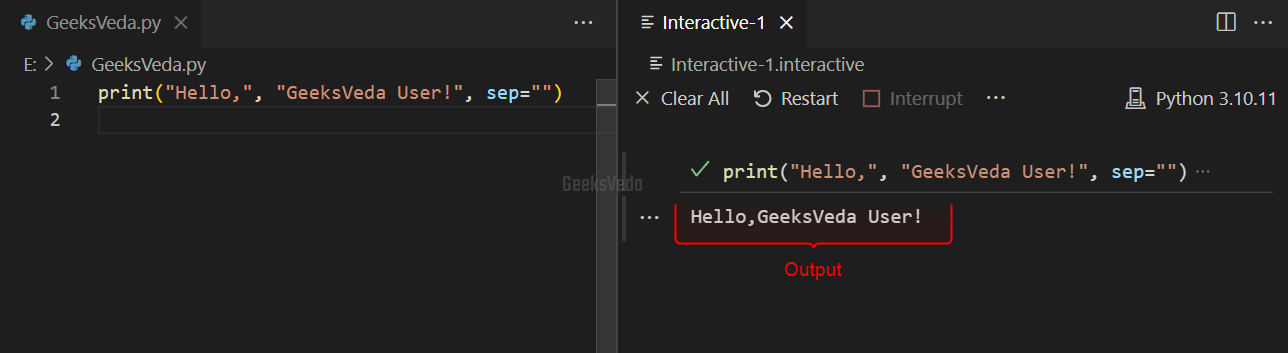
IndentationError: unexpected indent in Python
This error occurs when you have specified unnecessary indentation in your code. For instance, an unexpected indentation can be seen at the start of the third line of the code.
import sys sys.stdout.write("Hello GeeksVeda User!") sys.stdout.write("How's it going?")
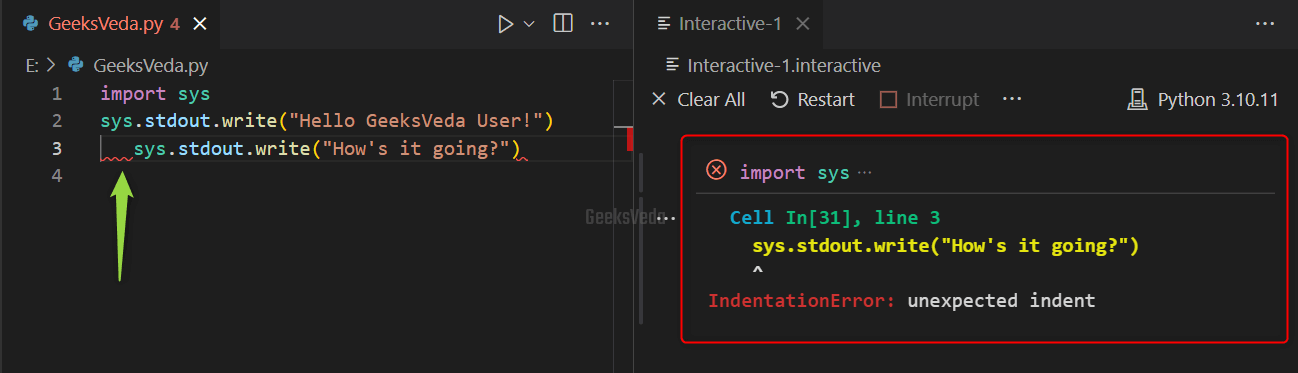
As a solution for this IndentationError, remove the extra indentation as follows.
import sys sys.stdout.write("Hello GeeksVeda User!") sys.stdout.write("How's it going?")
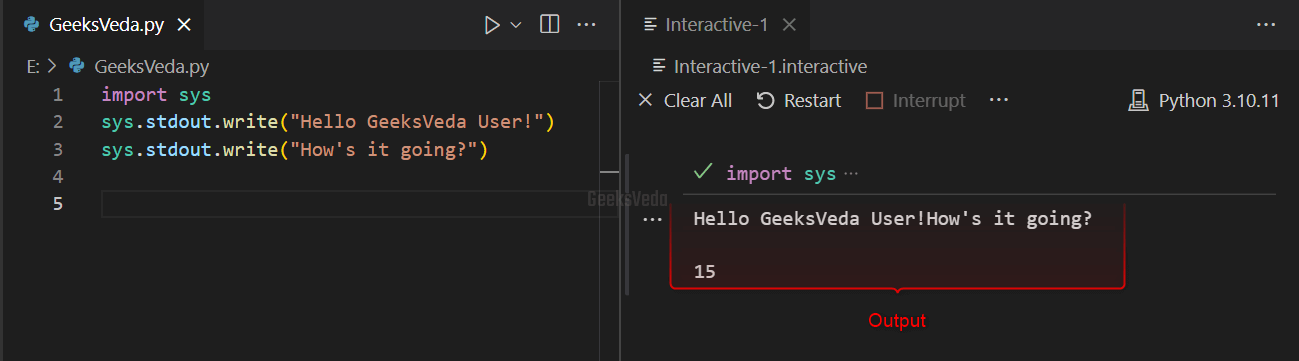
NameError: name ‘sys’ is not defined in Python
This error is a type of warning that states that the sys module is not imported in the Python code. For the demonstration, check the given program.
sys.stdout.write("Hello GeeksVeda User!") sys.stdout.write("How's it going?")
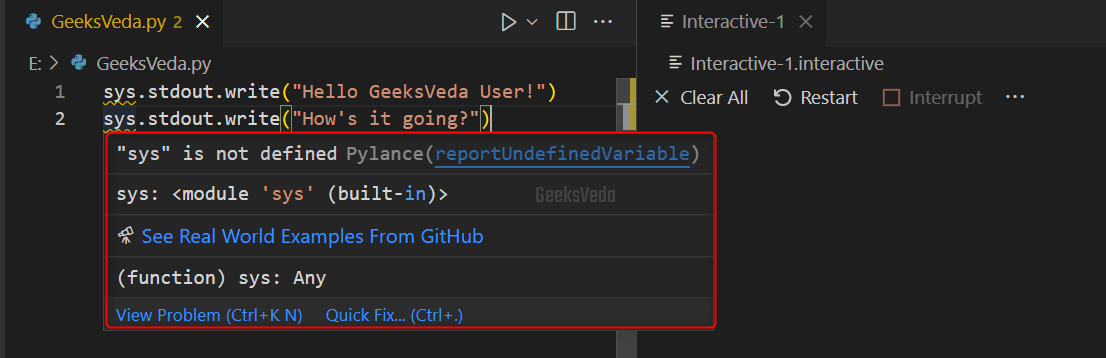
Import the sys module before using it as the solution.
import sys sys.stdout.write("Hello GeeksVeda User!") sys.stdout.write("How's it going?")
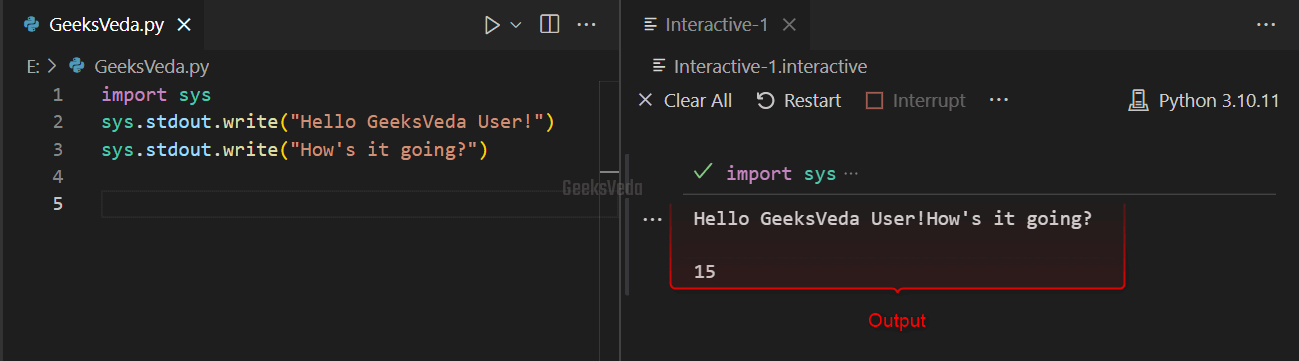
Python Troubleshooting Tips
- Validate that you have correctly spelled the method or function name that you are utilizing as it can lead to typos.
- Verify that you have imported the required modules.
- Check for extra or missing parentheses or opening or closing double quotation marks..
- Review the Python version you are using.
- Ensure that you pass the correct parameter values to the write() method or the print() function.
That was essential information regarding printing without a new line or space.
Conclusion
In Python, printing without a newline or space is a valuable technique that provides greater control over output formatting.
By utilizing the methods discussed in this article, such as end, sep parameters, write() method, or sys module, you can create more efficient and organized printed output.
Moreover, remember these troubleshooting tips to improve your Python skills and enhance the user experience in your projects.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!