While programming in JavaScript, you may encounter the situation to perform the shuffling arrays operation. It is a common task that is done to rearrange or shuffle the array in random order.
Whether you are developing a game, a data visualization tool, or any music player, shuffling arrays can add an X-factor of surprise and unpredictability.
Today’s guide will explore different ways to shuffle arrays in JavaScript, from the classic Fisher-Yates Shuffle Algorithm to modern techniques using built-in array methods.
Moreover, we will also give practical examples along with the relevant limitations.
1. Understanding Fisher-Yates Shuffle Algorithm
For shuffling arrays in JavaScript, you can utilize the Fisher-Yates algorithm, which works by starting the array ending and then swapping each element with a random element that is placed before it.
The stated process is repeated for each array element until the whole array has been randomized or shuffled.
Shuffle JavaScript Array Using Fisher-Yates Algorithm
In this example, we will define a function named “shuffle()
” that accepts “arr
” as an argument and outputs the shuffled version of the array. This can be done by iterating over the original array from end to start.
More specifically, at each iteration, a random number or index “j
” is generated between “0
” and “i
” using the Math.floor()
in combination with the Math.random()
method. Note that the current index “i
” is inclusive.
After that, the element at the index “i
” gets swapped with the index at the “j
” index. This process keeps repeating until all of the elements have been swapped for once at least.
function shuffle(arr) { for (let i = arr.length - 1; i > 0; i--) { const j = Math.floor(Math.random() * (i + 1)); [arr[i], arr[j]] = [arr[j], arr[i]]; } return arr; } const array1 = [3, 5, 6, 2, 1, 9]; console.log('Shuffled Version', shuffle(array1));
As a result, a fully shuffled array version will be displayed on the console.
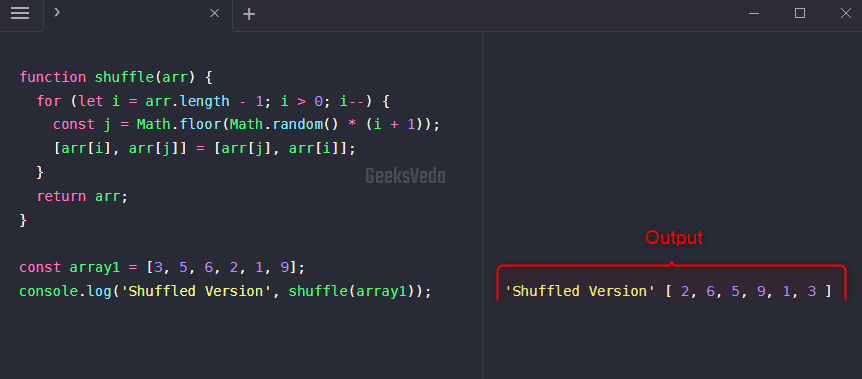
Benefits of Using Fisher-Yates Algorithm
Here, we have provided a list of some of the fantastic benefits of utilizing the Fisher-Yates algorithm.
- This algorithm produces a truly random and unbiased shuffled array by making sure that each array element has the same chance of being shuffled.
- It does not need any additional memory allocation as it shuffles or randomizes the original array.
- There is no preference for any particular shuffling.
- This algorithm works quickly for large arrays as well.
2. Understanding sort() Method in JavaScript
The JavaScript sort()
method is primarily utilized for sorting array elements in order as per requirements. This order can be ascending or descending.
However, you can also use this method for shuffling or randomizing the array elements.
Shuffle JavaScript Array Using sort() Method
In this particular example, we will check out the approach for using the “sort()
” method for shuffling the array elements.
For the corresponding purpose, we have passed a comparison function to the sort method as “() => Math.random() - 0.5
“. This function will generate a random number between -0.5 to 0.5.
More specifically, this function outputs a negative number having the probability of “50%
“. As a result, the sort()
method will tend to randomly shuffle the array in either descending or ascending array.
function shuffle(arr) { return arr.sort(() => Math.random() - 0.5); } const array1 = [3, 5, 6, 2, 1, 9]; console.log('Shuffled Version', shuffle(array1));
It can be observed, the shuffled array has been displayed.
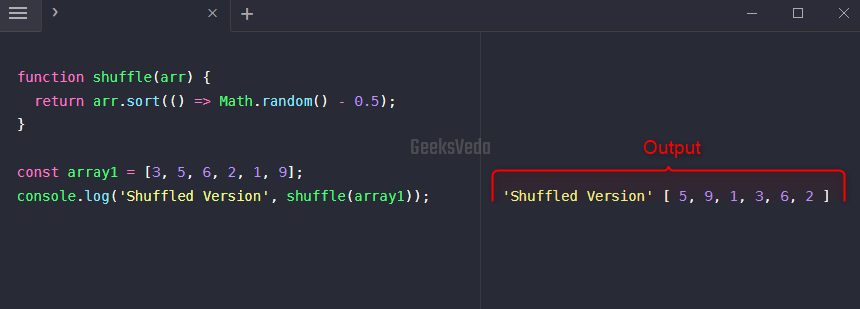
Limitations of sort() Method
There also exist some limitations to using the sort()
method for the array shuffling or randomizing operation.
- This method needs a comparison function which ultimately makes it more complex than other approaches.
- As the algorithm used by the
sort()
method was not specifically designed for randomizing or shuffling, therefore it can cause biased shuffling. - The
sort()
method does not work efficiently for shuffling arrays with large elements. - This method does not have the capability to save the original order of the elements. So, it can not be used for generating multiple shuffling or randomization of the same array.
3. Understanding Math.random() Method in JavaScript
JavaScript Math.random()
is a built-in method that assists in generating a random number between one and zero. However, this method can be utilized for randomly choosing array elements and then creating a shuffle array version.
Shuffle JavaScript Array Using Math.random() Method
In the provided example, the Math.random()
method is used in combination with the Fisher-Yates algorithm for the discussed purpose.
The algorithm starts working for initializing the variable “currentIndex
” with respect to the array length. After that, it then chooses a random index between 0 and the currentIndex
with the help of the Math.random()
number.
After finding it, the algorithm swaps the elements with the element present at the current index.
This whole operation will be repeated for all array elements, moving from the last to the first index, until the given array is completely randomized or shuffled.
function shuffle(arr) { let currentIndex = arr.length; let tempValue, randomIndex; while (currentIndex !== 0) { randomIndex = Math.floor(Math.random() * currentIndex); currentIndex--; tempValue = arr[currentIndex]; arr[currentIndex] = arr[randomIndex]; arr[randomIndex] = tempValue; } return arr; } const array1 = [3, 5, 6, 2, 1, 9]; console.log('Shuffled Version', shuffle(array1));
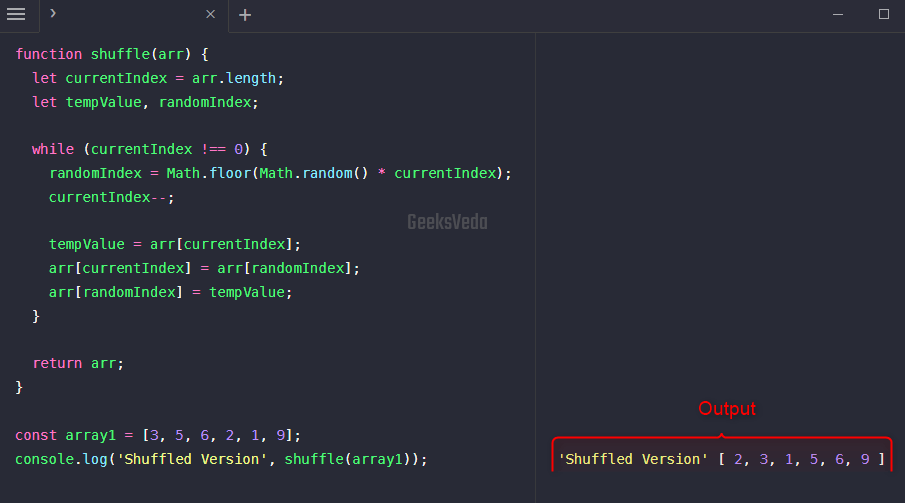
Limitations of Using Math.random() Method
Check out the enlisted limitations of using the Math.random()
method for array shuffling.
- This method generates pseudorandom numbers, which signifies that the numbers appear to be random but are generated by a formula or algorithm and thus can be predicted. This leads to biased shuffling or randomizing.
- There exists a chance that some values may be more likely to occur as compared to others.
- Its implementation is complex compared to other approaches.
- This method works slowly for large arrays.
4. Combining All Methods to Shuffle Arrays
You can also utilize the previously discussed three approaches for creating a more randomized shuffle which guarantees that each element will have an equal chance of being selected.
To do so, first, apply the Fisher-Yates algorithm for shuffling the array.
After that, call the sort()
method for shuffling the equal elements. Then, invoke the Math.random()
method for randomly shuffling the elements within an indices range.
function shuffle(arr) { let currentIndex = arr.length; let tempValue, randomIndex; // Using the Fisher-Yates Shuffle Algorithm while (currentIndex !== 0) { randomIndex = Math.floor(Math.random() * currentIndex); currentIndex--; tempValue = arr[currentIndex]; arr[currentIndex] = arr[randomIndex]; arr[randomIndex] = tempValue; } // Shuffling any equal elements with the .sort() method arr.sort(() => Math.random() - 0.5); // Randomly shuffling elements within a range of indices with the Math.random() method for (let i = 0; i < arr.length; i++) { const randomIndex = Math.floor(Math.random() * (arr.length - i) + i); const temp = arr[i]; arr[i] = arr[randomIndex]; arr[randomIndex] = temp; } return arr; } const array1 = [3, 5, 6, 2, 1, 9]; console.log('Shuffled Version', shuffle(array1));
Resultantly, we will get a highly randomized shuffled array that makes sure that each array element has an equal chance of being selected.
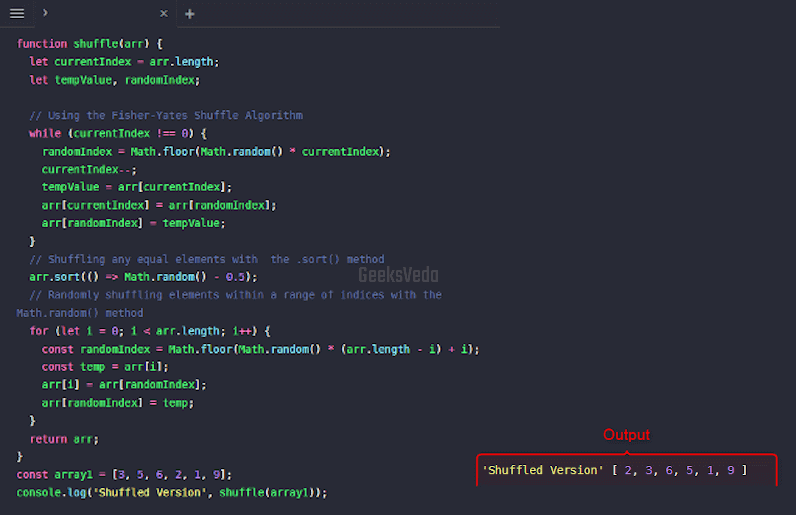
That’s all essential information regarding randomizing or shuffling a JavaScript array.
Conclusion
Although the operation of shuffling arrays may seem like a simple task. However, it can significantly affect the code.
There are several approaches to shuffle arrays in JavaScript, including the Fisher-Yates Shuffle Algorithm, the sort()
method, and the Math.random()
method.
Choose any of the mentioned approaches while keeping in mind their relevant limitations.