For any web developer, formatting dates is considered an essential task. You may need to perform this operation in use cases such as when you are working on a web app, blog, or on e-commerce website.
More specifically, displaying dates in a readable and clear format is crucial for offering a good user experience. JavaScript permits you to format dates according to your project requirements.
In today’s guide, we will explore multiple approaches for formatting dates which will mainly include built-in methods like toDateString()
, toLocaleDateString()
, and custom methods, such as Intl.DateTimeFormat()
method.
Lastly, we will also compare these approaches based on their functionalities.
1. Date Object in JavaScript
JavaScript Date Object refers to a single moment in time, which permits you to work with date and time while coding in JavaScript. Moreover, using it, you can perform several operations on them.
For instance, setting or getting, second, minute, hour, day, month, or year.
How to Create a New Date Object in JavaScript
In order to create a New Date Object in JavaScript, utilize the “new
” keyword that has to be followed by the Date()
constructor.
const date1 = new Date();
The given code will create a new Date object named date1
having the current date and time.
Alternatively, you can pass the date and time manually to the Date()
constructor.
const specificDate = new Date('May 9, 2023 12:00:00');
The Date()
constructor also accepts the milliseconds’ value as an argument.
const millisecondsDate = new Date(1652140800000);
JavaScript Get Date Methods
Date object support different methods that can be utilized for working with dates and times. Some of these methods are given in the following table with the description:
Method | Description |
getFullYear() | Outputs the year of the given date (in 4-digit format). |
getMonth() | Outputs the month of the given date (in 0-11 format). |
getDate() | Outputs the day of the month of the given date (in 1-31 format). |
getHours() | Outputs the hours of the given date in (0-23 format). |
getMinutes() | Outputs the minutes of the given date in (0-59 format). |
getSeconds() | Outputs the seconds of the given date in (0-59 format). |
getTime() | Outputs the number of milliseconds since January 1, 1970. |
setFullYear() | Sets the year value of the given date (in 4-digit format). |
setMonth() | Sets the month value of the given date (in 0-11 format). |
setDate() | Sets the day value of the month of the given date (in 1-31 format). |
setHours() | Sets the hour value of the given date (in 0-23 format). |
setMinutes() | Sets the minutes value of the given date (in 0-59 format). |
setSeconds() | Sets the seconds value of the given date (in 0-59 format). |
Now, we will utilize some of the above-given methods in the following program.
const date1 = new Date(); const year = date1.getFullYear(); const month = date1.getMonth(); const day = date1.getDate(); const hours = date1.getHours(); const minutes = date1.getMinutes(); const seconds = date1.getSeconds(); console.log(`Current date and time is: ${year}-${month}-${day} ${hours}:${minutes}:${seconds}`);
As a result, the current date and time will be displayed on the console in “YYYY-MM-DD HH:MM:SS
” format.
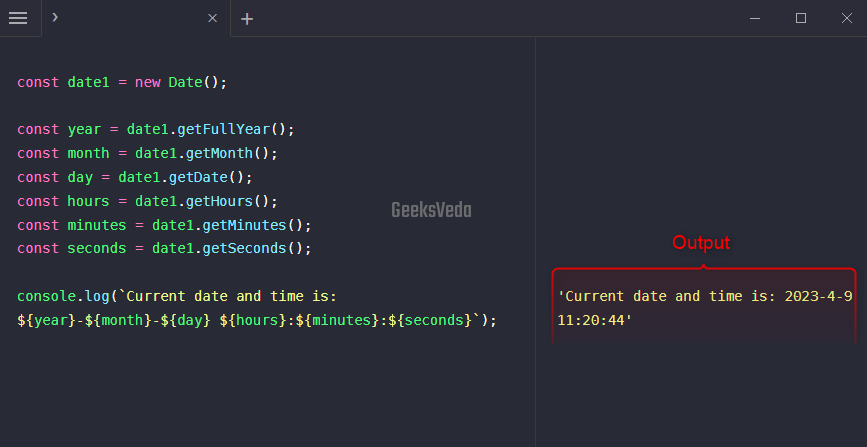
2. Built-in Date Formatting Methods in JavaScript
JavaScript also provides some built-in formatting methods for formatting date into strings.
These methods include “toDateString()
“, “toLocaleDateString()
“, and “toISOString()
” methods. The usage of all of these methods will be demonstrated in the upcoming sub-sections.
How to Use JavaScript Date toDateString() Method
The toDateString() method outputs a human-readable string representation of the date without the time values. It utilizes the format “Weekday Month Day Year
“, where:
- Weekday is the weekday’s full name.
- Month represents the month’s full name.
- Day refers to the month day.
- Year represents the year in four-digit format.
For instance, in the given code, we have first created a date object that stores the current date and time. After that, the toDateString()
method is invoked with the created date object to return the formatted string.
const date1= new Date(); const dateString = date1.toDateString(); console.log(`The Formatted date becomes: ${dateString}`);
It can be observed that the current date “Tue May 09 2023
” has been displayed by following the Weekday Month Day Year format.
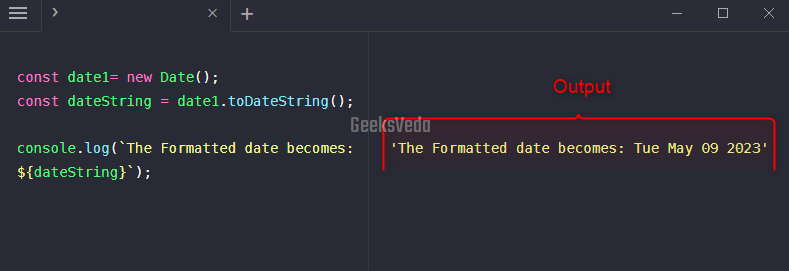
How to Use JavaScript Date toLocaleDateString() Method
The toLocaleDateString()
method also returns a human-readable string value of the date in the local format of the user. This method also neglects the time portion.
Moreover, the toLocaleDateString()
method accepts options arguments for defining the formatting and locale options.
Here is an example regarding the usage of the toLocateDateString()
Method in JavaScript.
const date1 = new Date(); const options = { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric' }; const dateString = date1.toLocaleDateString('en-US', options); console.log(`The Formatted date becomes: ${dateString}`);
Here, firstly we have created a date1
Date object. After that, we defined the formatting options and their relevant types.
In the next step, we have passed en-US as local, and the options object to the toLocaleDateString()
method.
Resultantly, the code will output the current date Tuesday, May 9, 2023.
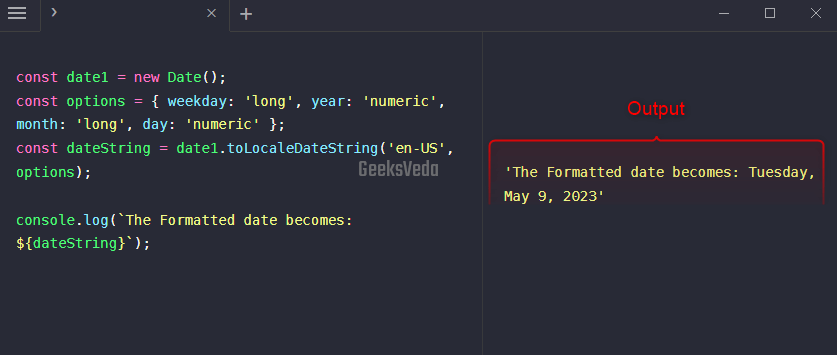
How to Use JavaScript Date toISOString() Method
The toISOString() JavaScript method is mostly utilized in the web APIs for returning a string representation of the date in ISO format, which is “YYYY-MM-DDTHH:mm:ss:sssZ
“.
You can simply call the toISOString()
method with the newly created Date object without passing any arguments as follows.
const date1 = new Date(); const isoString = date1.toISOString(); console.log(`The ISO date becomes: ${isoString}`);
The given program will display the current date in the mentioned ISO format.
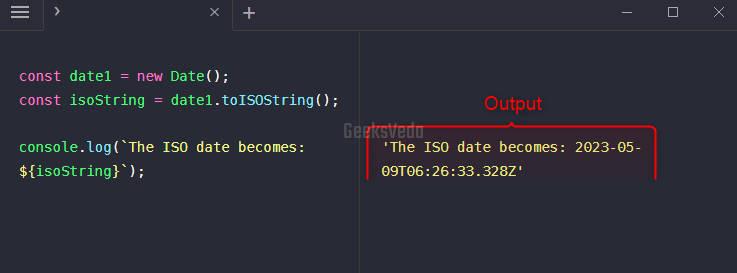
3. Custom Date Formatting in JavaScript
In JavaScript, you can utilize the “Intl.DateTimeFormat()
” method for creating customized date and time formats according to your requirements. Or, we can say that it enables you to customize the date and time formatting options.
How to Create a New DateTimeFormat Object
A new DateTimeFormat can be created with the Intl.DateTimeFormat()
method. The created object can be then used for formatting date and time format with respect to the defined locale and other options.
For instance, in the provided example, we will create a new DateTimeFormat object with the given “options
” and “en-US
” as the locale.
const options = { year: 'numeric', month: 'long', day: 'numeric' }; const formatter = new Intl.DateTimeFormat('en-US', options);
Different Format Options With Their Explanation
Some of the options that the Intl.DateTimeFormat()
method supports are enlisted below with the relevant formats.
- year – 2-digits, numeric, long.
- month – 2-digits, numeric, long, short.
- day – 2-digit, numeric.
- hour – 2-digit, numeric.
- minute – 2-digit, numeric.
- second – 2-digit, numeric.
- timeZoneName – long, short.
Now, let’s look at some of the examples of creating custom date formats.
Create Custom Time hh:mm:ss Format in JavaScript
The given program first creates a DateTimeFormat object with options representing the year, month, and day format.
Then the current date is formatted by utilizing the formatter object. The resultant value is stored in dateString1
. Lastly, the formatted date will be printed on the console.
const opts = { year: 'numeric', month: 'long', day: 'numeric' }; const formatter = new Intl.DateTimeFormat('en-US', opts); const date1 = new Date(); const dateString1 = formatter.format(date1); console.log(`The Formatted date becomes: ${dateString1}`);
It can be observed that we have successfully created a formatted string “May 9, 2023
” representing the current date in the given format.
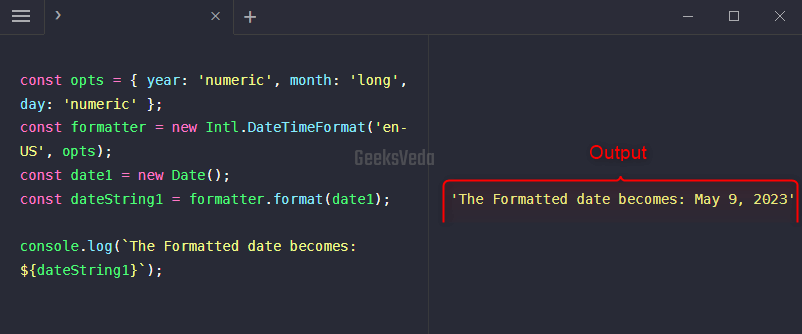
Create a Date as YYYY-MM-DD hh:mm:ss in JavaScript
The only difference in this example is that we have changed the format by defining the year, month, date, hour, minute, and second as the new format.
ll of the other code functionality will remain the same.
const opts = { year: 'numeric', month: '2-digit', day: '2-digit', hour: 'numeric', minute: 'numeric', second: 'numeric' }; const formatter = new Intl.DateTimeFormat('en-US', opts); const date1 = new Date(); const dateTimeString1 = formatter.format(date1); console.log(`The Formatted date and time becomes: ${dateTimeString1}`);
Resultantly, the current date and time will be displayed in the MM/DD/YYYY
, HH:MM:SS
format as follows.
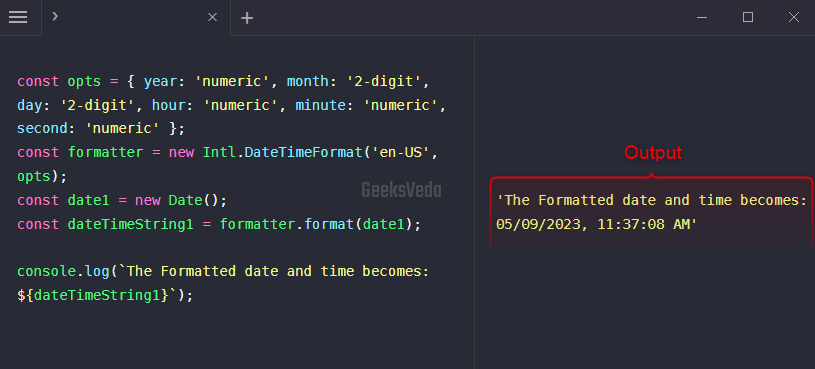
4. Comparison of Built-in and Custom Formatting Methods
Have a look at the given table in which we have compared built-in and custom formatting methods based on their features.
Features | Built-in Formatting Methods | Custom Formatting Methods |
Functionality | Offers limited formatting options | Customizable and flexible |
Learning curve | Easy | Needs understanding of options |
Format control | Limited | Allows full control over the style and format |
Dependency | None | Depends on custom functions or external library |
Localization support | Basic | Provides advanced locale options |
Browser support | Widely supported | Depends on the customized approach |
Customizability | Limited | Highly customizable |
Ease of use | Straightforward | Needs more configuration |
That’s all information regarding formatting date in JavaScript.
Conclusion
In JavaScript, the task of formatting dates can seem challenging at first. However, with the built-in and custom formatting methods, you can easily format dates according to your requirements.
Built-in methods are fast and easy to use, while custom methods provide more customizability and flexibility over the output format.
Moreover, with the examples and tips given in today’s post, you can start formatting dates like a pro!