Learn the approaches to comparing two dates in JavaScript with accuracy and precision in this comprehensive guide.
From understanding the essential Date object to handling time zones and addressing precision challenges, this blog will offer the most suitable approaches and best practices.
So, explore the power of date comparison in JavaScript to improve your web development skill and ensure seamless functionality for date-related operations.
Understanding Date Objects in JavaScript
In JavaScript, the Date object is mainly utilized for working with dates and times. It signifies a particular moment in time and offers multiple properties and methods for manipulating the date components.
More specifically, using the Date object, you can perform several operations like fetching the current date and time, creating new dates, and comparing dates.
Common Use Cases When Comparing Dates in JavaScript
In section, we will discuss the following use cases and their relevant challenges.
- Date Comparison Based on Equality.
- Date Comparison Based on Chronological Order
- Date Comparison Based on Time Consideration
1. Compare Two Dates Based on Equality
The “getTime()
” method of the Date object can be used for comparing two dates for equality in JavaScript. This method calculates the time value in milliseconds for the specified date.
After getting both times values, the strict equality operator “===
” is utilized for comparison.
For instance, in the provided example, firstly, we created two Date objects named d1
and d2
. After that, we added an “if
” statement that compares the value of both dates that are retrieved using the getTime()
method with each object.
Resultantly, the added message will be shown on the console.
const d1 = new Date('2023-05-14'); const d2 = new Date('2023-05-14'); if (d1.getTime() === d2.getTime()) { console.log('Dates are equal'); } else { console.log('Dates are not equal'); }
As both dates were the same or equal, the added message “Dates are equal” has been shown on the console.
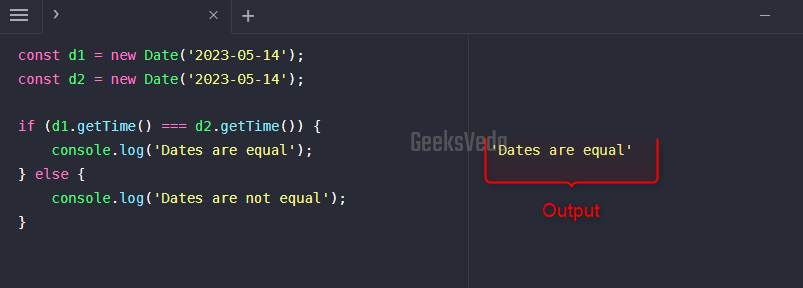
2. Compare Two Dates with Timezone in JavaScript
While comparing dates based on equality, some potential issues may occur related to precision and timezone differences. Particularly, the timezone difference can majorly affect the results of the date comparison, specifically if the defined date has originated from different timezones.
Here, in the given program, d1
represents the given date in UTC. Whereas, the d2
Date object refers to the same data but in GMT +3 time zone.
Observed that both Date objects are representing the same moment. However, the getTime()
method will consider both as different dates because of the added time zone.
const d1 = new Date('2023-05-14T12:00:00'); const d2 = new Date('2023-05-14T15:00:00'); if (d1.getTime() === d2.getTime()) { console.log('Dates are equal'); } else { console.log('Dates are not equal'); }
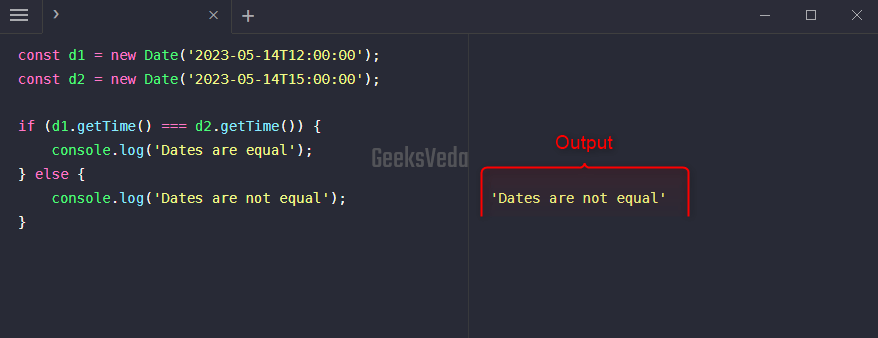
To resolve the mentioned issue, convert both dates or utilize the methods, such as getUTCHours()
or getUTCDate()
for the comparison.
const d1 = new Date('2023-05-14T12:00:00'); const d2 = new Date('2023-05-14T15:00:00'); if (d1.getUTCDate() === d2.getUTCDate()) { console.log('Dates are equal'); } else { console.log('Dates are not equal'); }
It can be observed that by using the getUTCDate()
method both dates are evaluated as equal.
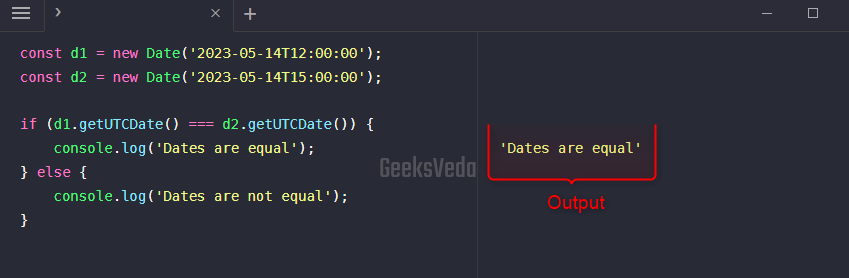
3. Compare Dates Based on Chronological Order in JavaScript
For comparing two dates based on their chronological order, utilize the “getTime()
” method for accessing the corresponding time values in milliseconds or the comparison operators ( >, <, >=, <=)
.
In this approach, you can find out which dates come after or before the other based on chronological order.
In the below example, the comparison operators “(< and >)
” are used in the if-else if statement. Based on the evaluated comparison result, the added message will be displayed on the console.
const d1 = new Date('2023-05-14'); const d2 = new Date('2023-05-15'); if (d1 < d2) { console.log('d1 comes before d2'); } else if (d1 > d2) { console.log('d1 comes after d2'); } else { console.log('d1 and d2 are the same'); }
As d1
represents 14th May 2023 and d2
refers to 15 May 2023, therefore the output signifies that d1 comes before d2
.
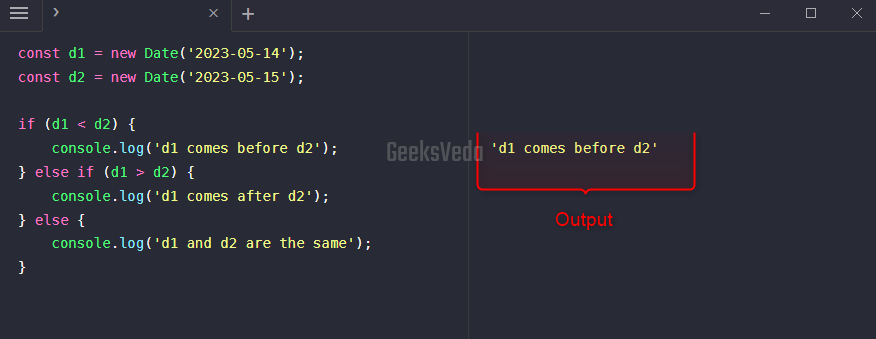
4. Different Date Formats in JavaScript
Different date formats also cause issues regarding accuracy and consistency. So, it is required to parse the dates into the Date object with the help of suitable techniques, like utilizing the “Date.parse()
” method.
It is recommended to normalize the dates to a certain timezone or convert them before performing the comparison.
For instance, we will now have two equal dates but in different formats. So, first, it is required to parse the dates by utilizing the Date.parse()
method and then pass this value to the Date()
constructor.
The remaining code will work the same as it previously.
const d1 = new Date(Date.parse('2023-05-15')); const d2 = new Date(Date.parse('15/05/2023')); if (d1 < d2) { console.log('d1 comes before d2'); } else if (d1 > d2) { ole.log('d1 comes after d2'); } else { console.log('d1 and d2 are the same'); }
Besides having different formats, both date values will be evaluated as equal.
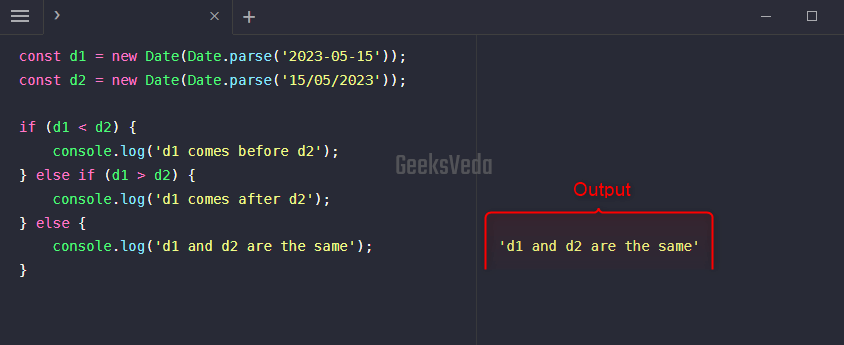
5. Compare Two Dates With Time Consideration
While comparing event timings, appointment schedules, or time-sensitive data, comparing dates based on time components becomes crucial. Thus, the time component must be considered for determining the chronological order, and neglecting it can lead to inaccurate sorting or incorrect results.
So, in order to compare two dates, you can utilize different methods such as getSeconds()
, getMinutes()
, and getHours()
for obtaining the particular time components.
Moreover, it is essential to ensure consistency and handle time zones appropriately.
const d1 = new Date('2023-05-14T12:00:00'); const d2 = new Date('2023-05-14T15:00:00'); if (d1.getHours() < d2.getHours()) { console.log('d1 comes before d2'); } else if (d1.getHours() > d2.getHours()) { console.log('d1 comes after d2'); } else { console.log('d1 and d2 have the same hour'); }
The given code compares d1
and d2
based on their hours with the getHours()
method. By comparing the hour components, we will determine the chronological order in accordance with time.
Based on the evaluation, it can be observed that d1
comes before d2
.
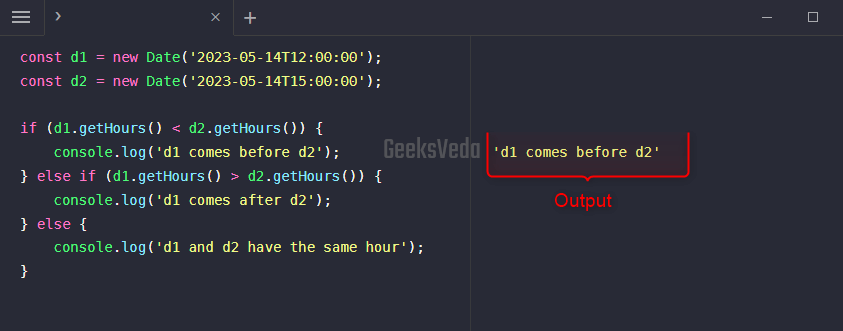
Best Practices for Comparing Two Dates in JavaScript
Check out the provided list of best practices for comparing two dates in JavaScript.
- Utilize the “
Date
” object for working with dates in JavaScript code. - Ensure to use a consistent date format for accurate comparison.
- Be aware of the precision issues.
- Consider time zone differences and normalize dates if required.
- Handle edge cases like daylight and leap years.
- Use comparison methods or Get Date methods for comparison.
That’s all from this informative guide related to comparing two dates in JavaScript.
Conclusion
Being a JavaScript programmer, it is essential for you to master the approach of comparing dates for performing accurate date-related operations in web development.
You can compare dates based on equality, chronological order, and time consideration using Date objects, their relevant methods, and comparison operations.
By following the best practices provided in this guide, you can ensure reliable and accurate date comparisons in your code.