The check if a number is even or odd JavaScript program is a simple program that takes a number as input and returns whether the number is even or odd. It is an essential program in programming and mathematics.
The importance of a program lies in the fact that determining whether a number is even or odd is a fundamental mathematical concept used in many areas of programming. It is also helpful for solving problems that involve working with numbers, such as calculating averages or finding prime numbers.
One should have a prior understanding of basic JavaScript syntax, arithmetic operations, and conditional statements to learn how to write a JavaScript program that finds if a number is even or odd.
It is also helpful to know the modulo operator, which gives the remainder of a division, and the bitwise AND
operator, which can be used as a faster technique to determine if a number is even or odd. However, the bitwise AND
operator is optional for beginners to know.
There are various JavaScript methods that you can use to check whether a number is even or odd.
1. Using JavaScript Modulo (%) Operator
You can use the modulo operator to check if a number is odd or even.
function checkEvenOrOddUsingModulo(number) { if (number % 2 === 0) { return "Even"; } else { return "Odd"; } } checkEvenOrOddUsingModulo(11); checkEvenOrOddUsingModulo(18);
This method uses the modulo operator (%)
to find the remainder when the number is divided by 2. If the remainder is 0, the number is even. If the remainder is 1, the number is odd.
For example, let us call the checkEvenOrOddUsingModulo(number)
with different arguments and see the results.
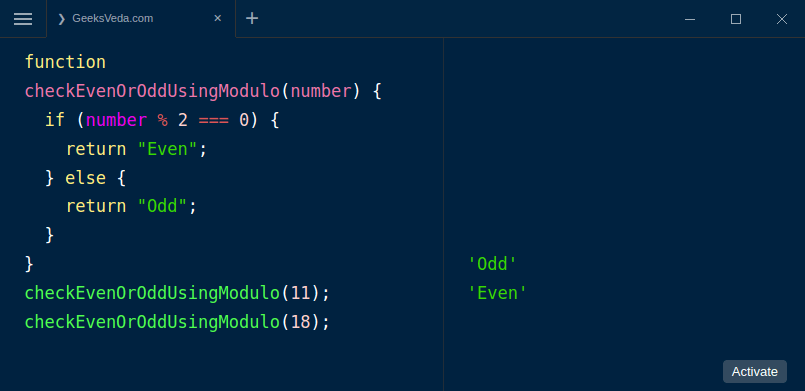
As you can see, 11 is odd while 18 is even.
2. Using JavaScript Bitwise AND (&) Operator
You can also use the bitwise AND
operator (&)
to determine if a number is even or odd, as shown below.
function checkEvenOrOddUsingBitwise(number) { if (number & 1) { return "Odd"; } else { return "Even"; } } checkEvenOrOddUsingBitwise(1); checkEvenOrOddUsingBitwise(0);
This method uses the bitwise AND
operator (&)
with 1. If the number is odd, your system will output 1 as the result. On the contrary, if the number is even, the result will be 0.
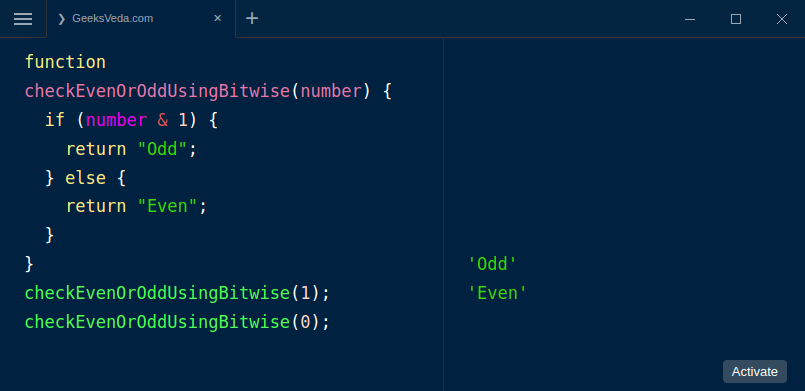
3. Using JavaScript Conditional Operator
This is the last method that you can use to find if a number is even or odd as shown.
function checkEvenOrOddUsingConditional(number) { return number % 2 === 0 ? "Even" : "Odd"; }
This method uses a conditional operator (? :)
to check whether the number is even or odd. If the number is even, it returns “Even“. Otherwise, it returns “Odd“.
Conclusion
For several reasons, learning how to write a JavaScript program to find if a number is even or odd can be beneficial. You can apply it to various programming problems, such as finding all even or odd numbers in a given range or checking if a given number is a multiple of another.
Do you have any other methods to check if a number is even or odd? you would want us to include in this article? Please share it in the comment box below.
Goodly written article.
Which method can I use for manipulating financial data with numbers with decimals? also, help me with a link for the JS program for counting the number of characters in a string.
Cannot see it here.
@Maria,
You can count the number of characters in a string using Javascript regular expressions as shown.