The Divide Two Numbers JavaScript program is a basic program that takes two numbers as input and returns the quotient of their division. It is a fundamental mathematical operation and an essential component of programming.
The importance of the program lies in the fact that division is one of the four basic arithmetic operations, along with addition, subtraction, and multiplication. It is a critical operation in programming used in many applications, including calculating averages, finding percentages, and determining proportions.
To execute a program that divides two numbers in JavaScript, you need to have a basic understanding of the following skills:
- Variables – You should know how to declare and initialize variables in JavaScript. In the case of a division program, you’ll need to create two variables to hold the numbers you want to divide.
- Functions – You should understand how to define and call functions in JavaScript. The division program will likely be implemented as a function that takes two parameters and returns their quotient.
- Arithmetic Operators – You should be familiar with arithmetic operators such as / used for division in JavaScript.
- Conditional Statements – You should know how to write if statements in JavaScript to check if the second parameter is zero and handle this scenario appropriately.
- Console Logging – You should be able to use console.log() to output the result of the division operation to the console.
A basic understanding of these JavaScript concepts should be sufficient to execute a program that divides two numbers. However, the complexity of the program may require additional knowledge and skills.
You can use several methods if you intend to divide two numbers in JavaScript as shown.
1. Using JavaScript Division (/) Operator
You can use the division operator as indicated in the code sample.
function divideUsingOperator(a = 30, b = 6) { return a / b; } console.log(divideUsingOperator());
This method uses the division operator (/)
to divide the first number by the second number. For example, you can provide default values for parameters a and b.
Therefore, calling the function with no arguments implies that the utility will use the default values as earlier defined. Note that the command console.log(divideUsingOperator()); that we call here has no arguments.
The above code uses the default values to divide 30 by 6 to produce 5 as the answer. Alternatively, you can call the same function but with new arguments.
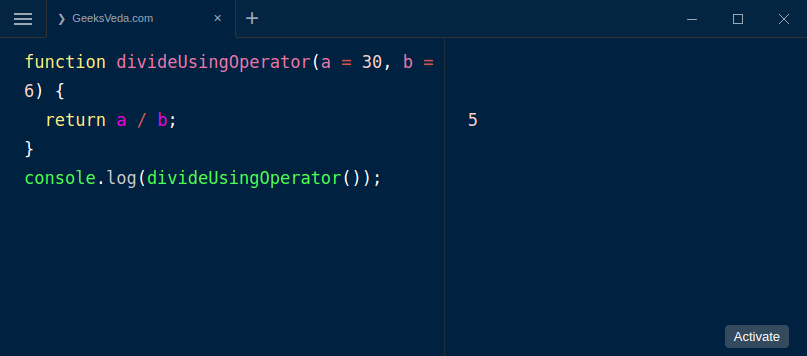
2. Using JavaScript divide() Function
Another way to divide two numbers is to create a function that takes two parameters and returns their quotient.
function divide(a, b) { return a / b; } let result = divide(10, 2); console.log(result);
In this example, we begin by defining a function called divide()
that takes two parameters, a and b, and returns their quotient using the division operator /
.
We then call the function with the two numbers we want to divide, store the result in a variable called result, and output it to the console using console.log()
.
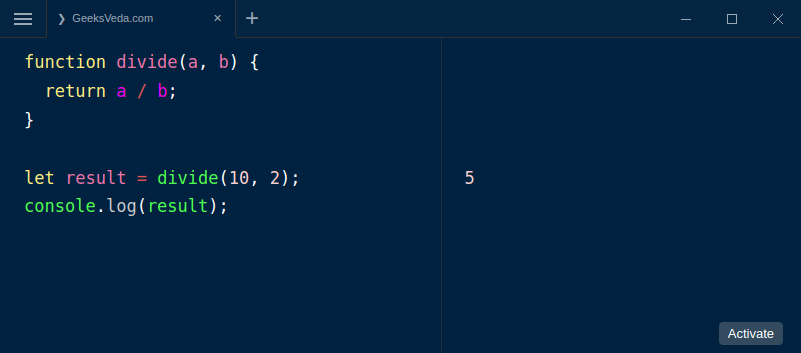
3. Using JavaScript Math.floor() Method
Another method is to use the Math.floor()
method, as shown in the example code below.
function divideUsingMathFloor(a, b) { return Math.floor(a / b); } console.log(divideUsingMathFloor(30,6));
This method uses the Math.floor()
method to round down the quotient by dividing the first number by the second number. This method can be useful if you want to ensure that the result is an integer.
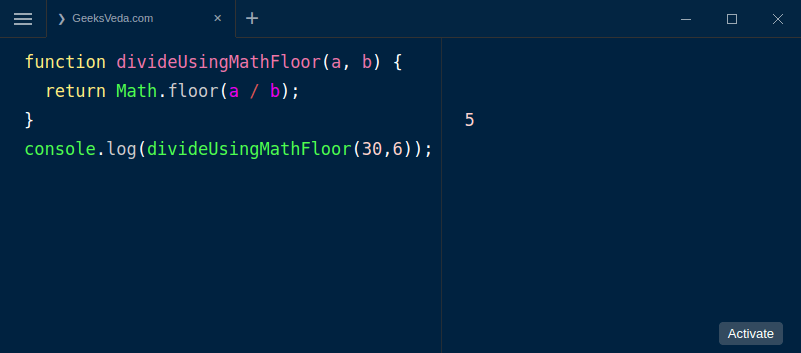
In this example, the divideUsingMathFloor
function takes two parameters a and b, and returns the result of dividing a by b, rounded down to the nearest integer using the Math.floor method.
The console.log
statement at the bottom is an example of how you could use the function and output the result to the console. When you run this code, it should output 5, which is the result of dividing 30 by 6 and rounding down to the nearest integer using Math.floor.
4. Using JavaScript Recursion
Finally, you can use recursion to divide two numbers in JavaScript, which subtracts the second number from the first number repeatedly until the first number is less than the second number.
The number of times the second number is subtracted is the quotient. This method can be useful if you want to perform division without using the division operator.
function divideUsingRecursion(a, b) { if (a < b) { return 0; } else { return 1 + divideUsingRecursion(a - b, b); } }
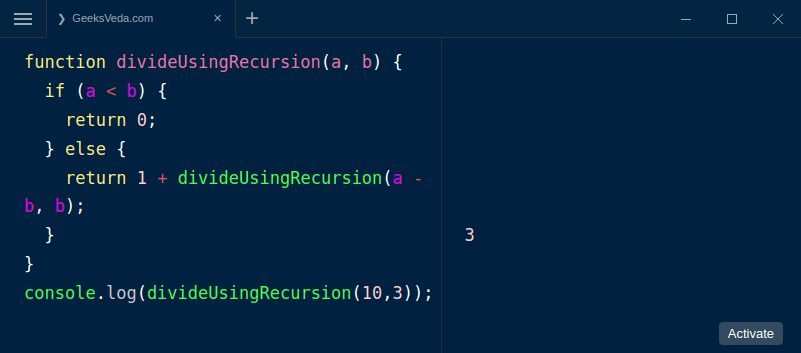
Conclusion
These methods should help you handle most JavaScript-building tasks. Of course, your choice will depend on your skills, personal preference, and the application you intend to build.
Do you have any other methods you would want us to include in this program? Please share it in the comment box below.