Like the “add two numbers” or “subtract two numbers” program, the “multiply two numbers” program is another JavaScript example worth discussing. It is an essential building block for more complex programs and is frequently used in various applications.
The importance of multiplying two numbers in a Javascript program cannot be overstated. Multiplication is one of the most critical mathematical operations, and it is used in a wide variety of applications, such as:
- Computing the total cost of an order – If you have the quantity of a product and its price, you can multiply these two numbers to get the total cost of the order.
- Scaling images or dimensions – If you want to resize an image or scale a set of dimensions, you can use multiplication.
- Calculating percentages – Percentages are often used in finance and business. You can use multiplication to calculate percentages, such as the percentage of a discount or the percentage of a tax.
- Performing mathematical calculations – Multiplication is a key operation in many mathematical calculations, such as finding the area of a rectangle, the volume of a cylinder, or the rate of change of a function.
You can multiply two numbers using the following methods:
1. Using JavaScript Multiplication (*) Operator
The most straightforward way to multiply two numbers in JavaScript is to use the multiplication (*)
operator as shown.
let a = 5; let b = 10; let product = a * b; console.log(product);
In this example, we create two variables, a and b, assign them the values 5 and 10, respectively, and multiply them using the *
operator. The result is stored in a third variable product, then printed to the console.
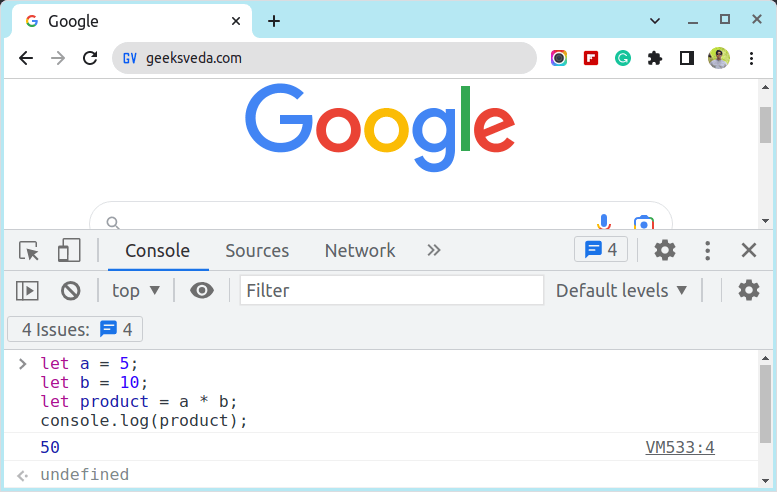
2. Using JavaScript Variables (*) Operator
The following example begins by defining two variables (num1 and num2) and assigning them the values of 10 and 5, respectively.
We then use the "*"
operator to multiply num1 by num2 and store the result in a product variable. Finally, we log the product’s value to the console, which should output 50.
const num1 = 10; const num2 = 5; const product = num1 * num2; console.log(product);
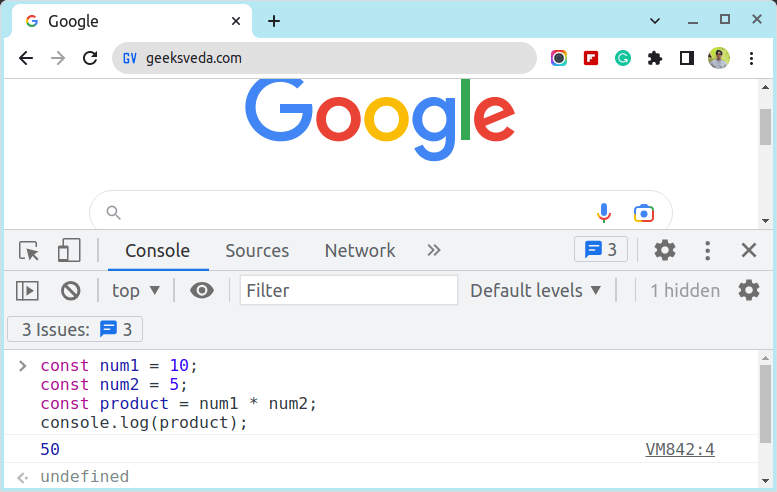
3. Multiplying Number Using JavaScript Number() Function
Another effective method of multiplying two numbers in JavaScript is by using the Number()
function as shown.
const num1 = "10"; const num2 = "5"; const product = Number(num1) * Number(num2); console.log(product);
This method multiplies two numbers by first converting the strings into numbers before using the “*
” operator to multiply the numbers.
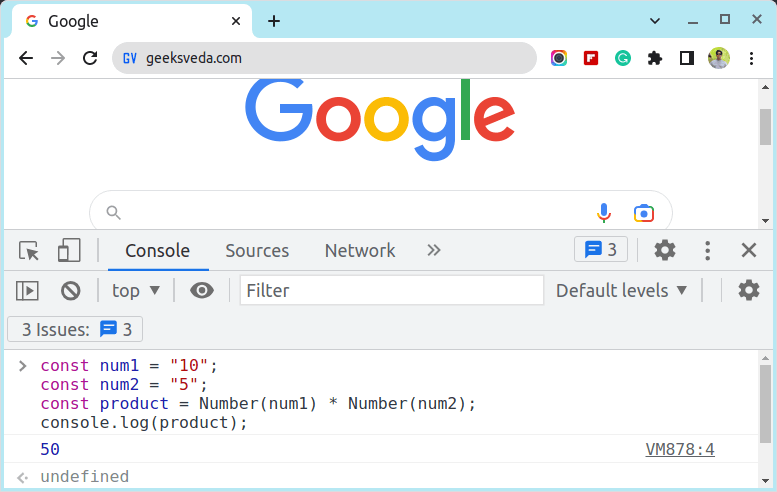
4. Multiplying Number Using JavaScript parseFloat() Function
Another method used in executing the multiply two numbers in JavaScript is the parseFloat()
function as shown below.
const num1 = "10"; const num2 = "5"; const product = parseFloat(num1) * parseFloat(num2); console.log(product);
This method also works by first converting the strings into numbers before multiplying them with each other.
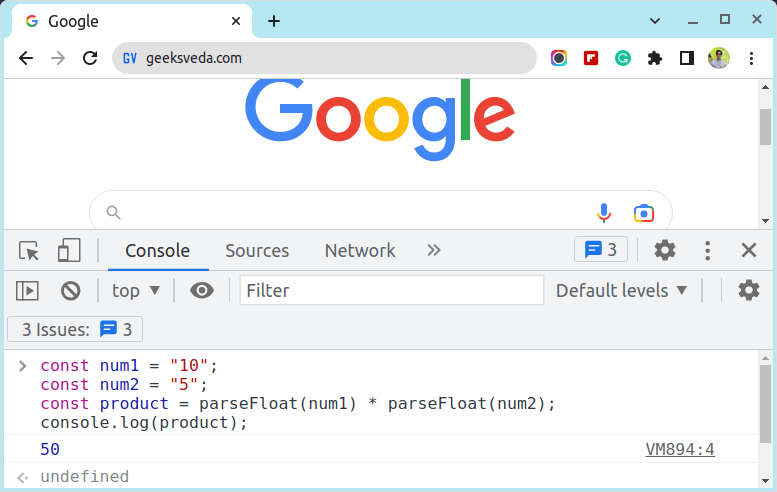
5. Using JavaScript multiply() Method
JavaScript’s Math object provides a multiply()
method that can be used to multiply two numbers.
let a = 5; let b = 10; let product = Math.multiply(a, b); console.log(product);
In this example, we use the multiply()
method of the Math object to multiply the variables a and b. The result is stored in the product variable, then printed to the console.
Note that the multiply()
method is not part of the standard JavaScript specification, so it may not be available in all environments.
Conclusion
These methods achieve the same result of multiplying two numbers together, but they have different use cases depending on the situation.
For example, using variables and the "*"
operator is the simplest and most straightforward method while using Number()
or parseFloat()
functions are useful when working with user input or when the numbers are stored as strings.