In Object-oriented programming (OOP), inheritance is considered a crucial concept for promoting the reuse of the code. It also assists in structuring classes.
However, as your project grows in complexity, you may encounter situations where the parent class is not sufficient. This is the point where multiple inheritance can help you out!
In today’s guide, we will discuss Python multiple inheritance and its relevant concepts, such as the diamond problem and the method resolution order. Let’s start this journey!
What is Inheritance in Python
Inheritance refers to the concept in OOP where a subclass or child class inherits the behaviors and properties from the super or parent class. More specifically, the subclass can extend and specialize the functionalities of the parent class.
This concept prompts code reusability and also reduces redundant code.
Additionally, by implementing inheritance, you are permitted to create specialized classes and maintain a clear structure as well.
1. Class Hierarchy and Parent-Child Relationships
Inheritance creates a hierarchical structure between classes. This concept is also called the parent-child relationship.
In such a scenario, the parent class offers a blueprint for common methods and attributes. On the other hand, child classes can add new features or override the existing ones.
2. How to Implement Inheritance
In the following example, we will implement inheritance using two classes “Animal
” and “Cat
“. The “Animal
” class is the base class having the “speak()
” method.
This method is initialized as an empty method using the pass statement (for the purpose of overriding by the subclass).
“Cat
” is the subclass of “Animal
“. This class has its own “speak()
” method which outputs the “Meow
” string.
After defining both classes, we created instances of both classes and invoked the “speak()
” method on each.
class Animal: def speak(self): pass class Cat(Animal): def speak(self): return "Meow" # Create instances of the classes animal = Animal() cat = Cat() # Call the speak method on instances animal_sound = animal.speak() cat_sound = cat.speak() # Print the results print("Animal Sound:", animal_sound) print("Cat Sound:", cat_sound)
As you can see, the “speak()
” method for the base class has displayed “None
“, whereas, for the subclass, it shows “Meow
“.
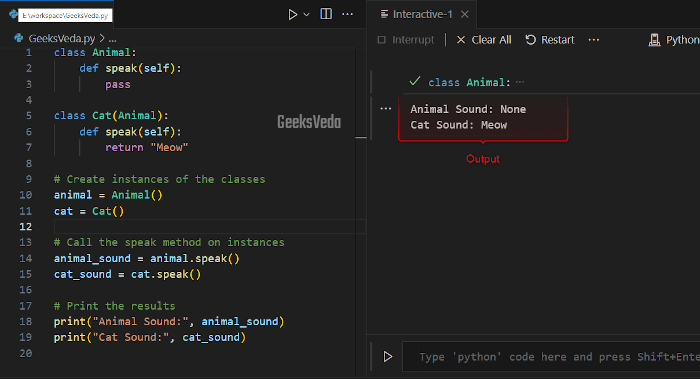
What is Multiple Inheritance in Python
Python multiple inheritance is all about inheriting the methods and attributes from more than one parent or base class. It permits the child classes to combine the features from multiple sources and utilize them effectively.
How to Implement Multiple Inheritance
According to the given code, we have three classes, “Person
“, “Scholar
“, and “Student
“, where:
- The “
Person
” class initializes a person’s name. - The “
Scholar
” class initializes a scholarship amount. - The “
Person
” class inherits both the “Person
” and “Scholar
” classes.
More specifically, the “Person
” utilized the constructors of the other two classes to initialize its attributes. This enables the “Student
” object to access both “name
” and “scholarship
” attributes.
class Person: def __init__(self, name): self.name = name class Scholar: def __init__(self, scholarship): self.scholarship = scholarship class Student(Person, Scholar): def __init__(self, name, scholarship): Person.__init__(self, name) Scholar.__init__(self, scholarship) # Creating instances of the Student class student1 = Student("Hanaf", 5000) student2 = Student("Zoha", 3000) # Accessing attributes of the Student instances print(f"{student1.name} has a scholarship of ${student1.scholarship}") print(f"{student2.name} has a scholarship of ${student2.scholarship}")
It can be observed that the attributes of the created instances have been successfully accessed and displayed with the print() function.
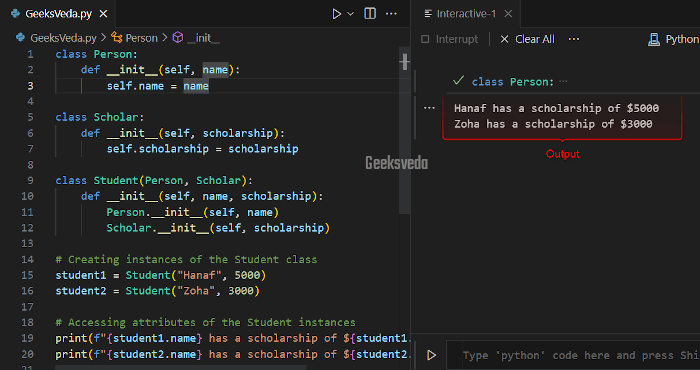
What is Diamond Problem in Multiple Inheritance
In Multiple Inheritance, the Diamond problem is a common issue that arises when a class inherits from two or more classes that already have a common base class. This specifically created a diamond-shaped inheritance hierarchy.
So, in order to solve the diamond problem, you can utilize the Method Resolution Order (MRO). Additionally, using this strategy and properly designing the class hierarchy can prevent conflicts.
1. How to Solve Diamond Problem
In the following example, we have four classes “A
“, “B
“, “C
“, and “D
“, where “D
” is the subclass of “B
” and “C
“.
C
” class itself has been inherited from the “A
” class which initiates the diamond problem.Now, when the “show()
” method has been invoked with the class “D
“” instance, Python utilizes MRO for evaluating the method resolution sequence.
class A: def show(self): print("Class A") class B(A): def show(self): print("Class B") class C(A): def show(self): print("Class C") class D(B, C): pass # Creating an instance of class D obj = D() # Calling the show method obj.show()
MRO will make sure that the “show()
” method has been searched and executed in a particular order, which consequently, displays the output “Class B” because of the order of inheritance.
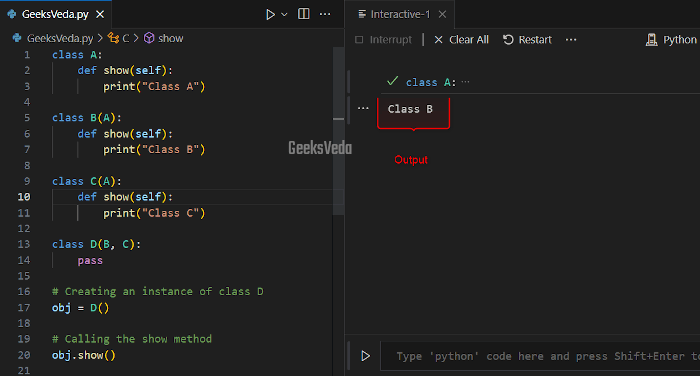
Method Resolution Order (MRO) in Python Inheritance
Method Resolution Order (MRO) is the sequence in which it looks for the methods in the class hierarchy while implementing multiple inheritance. It makes sure that methods are invoked in a predictable and consistent manner. Applying this methodology will also avoid ambiguities.
1. How Python Determines MRO?
Python utilizes the C3 linearization algorithm for evaluating the MRO. It follows the depth-first, left-to-right approach for prioritizing the methods from the parent classes.
Additionally, this algorithm creates a linear order of classes, which also resolves the ambiguity of the diamond problem.
2. Implement MRO in Multiple Inheritance
Here, we have three classes “X
“, “Y
“, and “Z
“, where “Z
” inherits from both “X
” and “Y
” classes.
So, when the “show()
” method has been invoked with the class “Z
” instance, Python will follow the MRP for determining the method resolution sequence.
MRO will make sure that the show()
method of the class “X
” runs first and displays the output “Class X
” when the show()
method has been called with an “obj
” instance.
class X: def show(self): print("Class X") class Y: def show(self): print("Class Y") class Z(X, Y): pass # Creating an instance of class Z obj = Z() # Calling the show method obj.show()
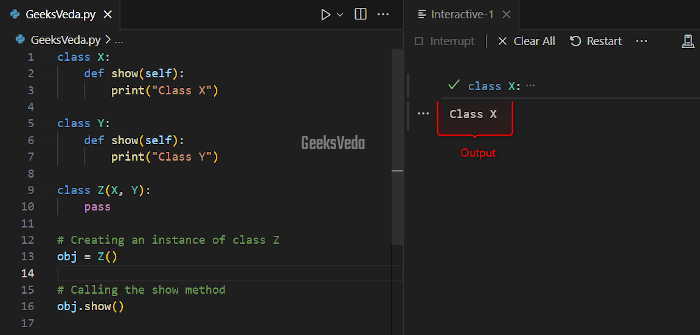
Single Inheritance vs Multiple Inheritance in Python
Here is the comparison of single and multiple inheritance based on the listed features:
Feature | Single Inheritance | Multiple Inheritance |
Number of Parents | Inherits from only one parent class. | Inherits from more than one parent class. |
Class Hierarchy | Linear hierarchy. | Hierarchical and complex class hierarchy. |
Code Reusability | Limited code reusability. | Greater code reusability through multiple sources. |
Method Conflicts | Minimal method conflict possibilities. | Potential method conflicts due to multiple sources. |
Simplicity | Simpler and easier to understand. | Complex to manage, especially with large hierarchies. |
Ambiguities | Minimal ambiguities and MRO straightforward. | May introduce ambiguities and require careful MRO. |
Implementation | Easier to implement and maintain. | Can be challenging to implement and troubleshoot. |
Flexibility | Less flexible in terms of combining behaviors. | More flexible for combining diverse behaviors. |
That’s all from this effective guide regarding applying multiple inheritance in Python.
Conclusion
Python multiple inheritance provides a robust way for building class structure. It also enables you to reuse the code effectively. Moreover, by understanding the Diamond problem, Method Resolution Order, and exploring alternative techniques, you can handle the challenges related to multiple inheritance.
Choose the approach as per your project requirement and create flexible class designs for your Python applications.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!