When we work with objects in programming, it’s important to remember that if an object has a value of None, we cannot call it a function and the same rule applies to Python as well.
If we mistakenly try to call a None object, we encounter a specific error known as “TypeError: ‘NoneType’ object is not callable“.
This article will discuss some of the code examples to explain and fix this error in more detail.
What is TypeError: NoneType Object Is Not Callable in Python?
In Python, ‘None‘ is a special built-in value that represents the lack of an object or the absence of a value, which is commonly used to indicate that a variable has not been assigned a value or that a function does not have a valid return value.
When we try to encounter the “NoneType” error, it means that we are trying to do a callable operation, such as invoking a method or function, or calling an object that has the value of ‘None‘.
There are several reasons why we get the ‘TypeError: NoneType Object Is Not Callable‘ error while coding in Python as discussed below.
- Assignment of None – We might have accidentally assigned None to a variable that should have held a callable object, such as a function or method.
- Missing Return Statement – If a function does not have a return statement or if the return statement is missing for a specific condition, it will default to returning None. Consequently, trying to call that function and expecting a callable object will result in an error.
- Incorrect Function or Method Name – It’s possible that you misspelled the function or method name, or you provided an incorrect reference, leading to a None value instead of a valid callable.
- Overwriting a Function with None – If you assign None to a variable that previously held a function, trying to call that variable as a function will raise this error.
- Incorrect Variable Assignment – You might have mistakenly assigned None to a variable that should have held a callable object.
How We Can Avoid These Mistakes?
- Check Variable Assignment – Ensure that you haven’t accidentally assigned None to a variable that should hold a callable object.
- Include a Return Statement – Make sure all functions have a return statement or return a value for all possible code paths.
- Verify Function or Method Name – Double-check the spelling and correctness of the function or method name being called.
- Avoid Overwriting Functions – Avoid assigning None to a variable that previously held a function.
- Confirm Proper Function Invocation – Ensure you are correctly using parentheses
()
when calling a function, as omitting them will result in trying to call the function object itself.
There are some other techniques and things that we should also consider while coding in Python, which are discussed ahead in this article.
1. Assign a Variable in Python
In the first example, a variable will be assigned a “None” value and then it will be called to see if the error occurs or not.
My_value = None My_value()

As the assigned value is None, the output will have the TypeError as None Type because our object is not callable here.
2. Python Function Return Value
In this example, let’s take a function and return a value to see what it is going to return.
def calculate_result(): if True: return None else: return 42 result = calculate_result() result()
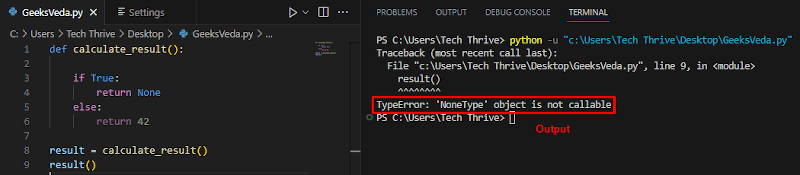
In this code example, there is a function calculate_result()
that returns None based on a condition. The function is called, and its return value is assigned to the variable result.
However, since the result is None, trying to call it as a function raises a “TypeError” because None is not callable.
To resolve the error, ensure that the value assigned to the result is a callable object, such as a function.
def calculate_result(): if True: return lambda: None else: return lambda: 42 result = calculate_result() result()
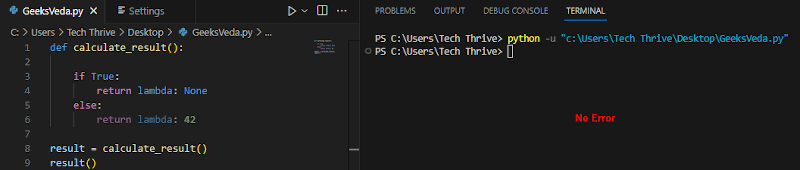
Now, the code is modified as the calculate_result()
function is returning a callable object instead of None.
3. Debug a Variable Value in Python
In this code example, we will have a function, that will return None.
def get_object(): return None my_object = get_object() print(my_object) my_object()

As it is clear from the output, printing the value of my_object
, we can see that it is indeed None. When we try to call my_object as a function, the error is raised.
To solve the error where we need to ensure that my_object
is a callable object before invoking it.
def get_object(): return None my_object = get_object() if callable(my_object): my_object() else: print("The object is not callable.")
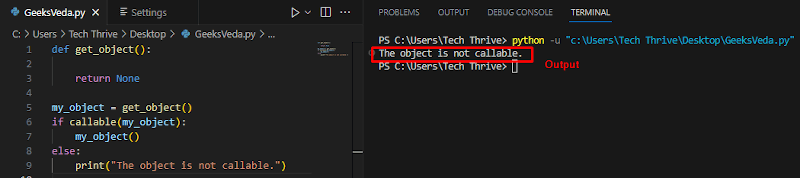
As you see, the error is no more as our object is callable this time.
4. Assign a Function to a Variable in Python
In this example, we will assign a function to a variable, which has a value in it as None. Eventually, the variable will also have the value of None.
def get_value(): return None value = get_value() value()

As the function “get_value()
” has a value of None, it will raise the ‘TypeError: NoneType object is not callable” error. When attempting to call value as a function.
To solve this error in this code snippet, we need to ensure that the value is a callable object before invoking it.
def get_value(): return None value = get_value() if callable(value): value() else: print("The value is not callable.")
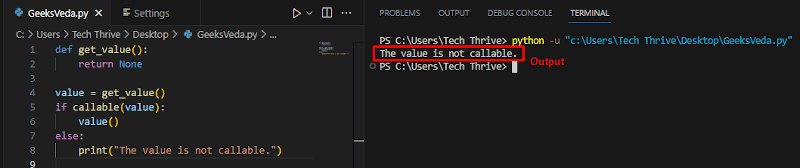
So here we do not have the TypeError anymore.
How to Address TypeError: NoneType Error in Python
Here are some strategies to address this error:
- Review the logic of the function or variable that is returning None. Ensure that it returns a valid callable object instead, such as a function or a method.
- Check if the object is callable before invoking it using the
callable()
function, which allows you to handle non-callable objects appropriately and prevent errors from occurring. - Implement proper error handling techniques such as try-except blocks to catch and handle the “TypeError” gracefully, providing meaningful error messages to the user.
By understanding the causes of this error and applying the appropriate solutions, you can effectively resolve the “TypeError: ‘NoneType’ object is not callable” error in your Python code, ensuring the smooth execution of your programs.
Conclusion
In conclusion, the “TypeError: ‘NoneType’ object is not callable” error in Python occurs when you try to invoke a non-callable object of type NoneType. This error often arises when a function or variable that should return a callable object mistakenly returns None.
To resolve this error, it is important to carefully examine the code and identify where the non-callable object is being used as if it were a function or method.