While programming in Python, you may come across a scenario where it is required to verify if a number is even or odd. This validation is particularly useful for optimizing the algorithms for enhancing the user experience as well.
This guide will discuss several techniques for odd or even number checking in Python. From the Modulo
operator, bitwise AND
operator, division
, and remainder
, to conditional Ternary operator, and Bit Manipulation, and Shift, all of these approaches will be explained briefly!
Why is it Important to Know Odd or Even Numbers in Python
Understanding whether a number is odd or even is considered a fundamental building block of different applications and algorithms.
Whether you are implementing the iterative loops, optimizing computations, making sure that the data has been parsed accurately, and verifying if a number is odd or even plays an important role.
Here are some of the relevant use cases:
- Loop Control – Odd-even checks can guide the behavior of loops, helping you perform specific actions on odd or even iterations.
- Data Segregation – Segregating data based on parity facilitates targeted processing and analysis of subsets.
- Optimizing Algorithms – Odd-even numbers influence the efficiency of algorithms, affecting branching and logical decisions.
- Cryptography – In certain cryptographic algorithms, odd and even numbers play a role in creating secure keys and functions.
- Game Mechanics – In game development, determining whether a player’s score is odd or even can trigger special in-game events.
How to Check If a Number is Odd or Even in Python
In order to verify if a number is odd or even in Python, you can use:
- Modulo Operator
- Bitwise AND Operator
- Division and Remainder
- Conditional Ternary Operator
- Bit Manipulation and Shift
Let’s practically implement each of the listed methods!
1. Using Modulo Operator
With the help of the Modulo
operator “%
“, you can easily determine if a number is odd or even. This operator calculates the remainder when dividing a number by 2.
Resultantly, it permits you to distinguish between numbers that leave a remainder and those that do not.
For instance, in the following program, the “check_odd_even()
” function utilizes the modulo operator “%
” for checking if a number is even (when the reminder is 0) or odd (when the remainder is 1).
def check_odd_even(number): if number % 2 == 0: return "Even" else: return "Odd" input_number = int(input("Enter a number: ")) result = check_odd_even(input_number) print(f"The number is {result}.")
Here, we will input “22“. As you can see the number has been evaluated as even and the relevant message printed out.
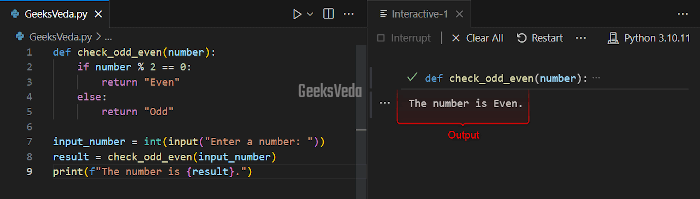
2. Using Bitwise AND Operator
Bitwise operations offer an unconventional way of checking the parity of a number. With the help of the bitwise AND operator “&
” with “1“, you can examine the least significant bit for determining whether a number is odd or even.
Here, the “check_odd_even()
” function uses the bitwise AND operator for directly checking the least significant bit. More specifically, if the least significant bit is 1, the number is odd, otherwise, it will be considered even.
def check_odd_even(number): if number & 1: return "Odd" else: return "Even" input_number = int(input("Enter a number: ")) result = check_odd_even(input_number) print(f"The number is {result}.")
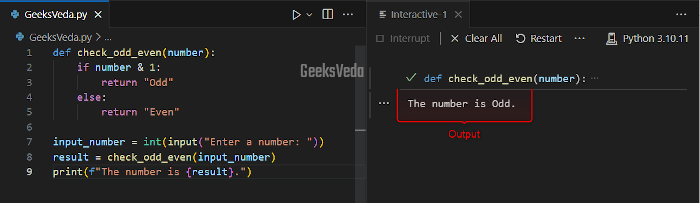
3. Using Division and Remainder
Dividing a number by 2 and evaluating the remainder is a classic method for distinguishing odd and even numbers.
According to the given program, the “check_odd_even()
” function will use division by 2 followed by 2 by multiplication to check if the number remains unchanged. If it does, the number will be evaluated as even. In the other case, it will be odd.
def check_odd_even(number): if number // 2 * 2 == number: return "Even" else: return "Odd" input_number = int(input("Enter a number: ")) result = check_odd_even(input_number) print(f"The number is {result}.")
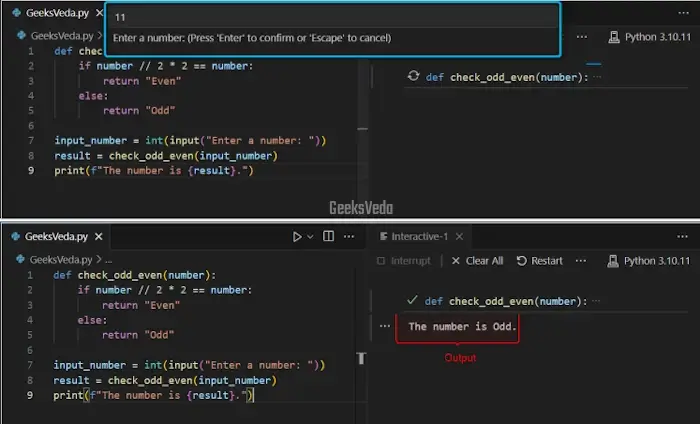
4. Using Conditional Ternary Operator
Python’s conditional ternary operator offers a concise approach to determining whether a number is odd or even. It minimizes the if-else structure into a single line, making it ideal for straightforward parity checks.
Here, the “check_odd_even()
” function utilizes the ternary operator for determining the parity of the number in a more concise form.
def check_odd_even(number): return "Even" if number % 2 == 0 else "Odd" input_number = int(input("Enter a number: ")) result = check_odd_even(input_number) print(f"The number is {result}.")
Here, we will input “44“. As a result, the number has been evaluated as even and the relevant message printed out.
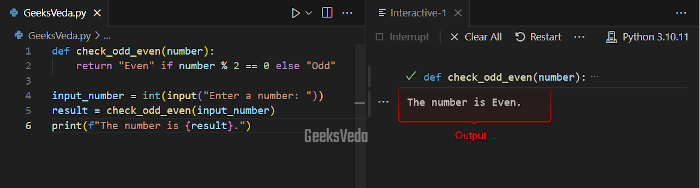
5. Using Bit Manipulation and Shift
You can also apply the bit manipulation technique to determine the parity. By utilizing the bitwise shifts and masking, you can isolate the least significant bit and thereby determine whether the number is odd or even.
For instance, in the following program, the “check_odd_even()
” function uses the bitwise shift “>>
” and AND operation “&
” for isolating the least significant bit and checking its value.
def check_odd_even(number): if (number >> 0) & 1: return "Odd" else: return "Even" input_number = int(input("Enter a number: ")) result = check_odd_even(input_number) print(f"The number is {result}.")
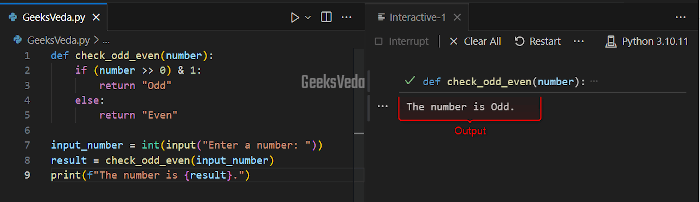
Choose the Best Method to Check Odd or Even Number
Let’s compare each of the mentioned methods for choosing the right approach:
Method | Advantages | Disadvantages | Use Cases |
Modulo Operator |
|
|
General-purpose odd-even checks in clear and concise code. |
Bitwise AND Operator |
|
|
Situations where memory and speed are crucial, like embedded systems. |
Division and Remainder |
|
|
Basic parity checks in scenarios involving positive integers. |
Conditional Ternary Operator |
|
|
Compact odd-even checks in concise code segments. |
Bit Manipulation and Shift |
|
|
Situations where understanding bit manipulation is desired or for large numbers. |
This brought us to the end of our guide related to checking even or odd numbers in Python.
Conclusion
Whether you want to go for a traditional modulo operator, bitwise operations, or ternary operator, the choice of a technique for checking even or odd numbers depends on your project requirements.
Each approach has its own advantages and disadvantages, and use cases, which can handle the diverse preferences and scenarios in Python programming.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!