In the context of timekeeping, leap years are those years that contain an extra day and maintain the balance between the calendars and the Earth’s orbit around the sun.
As a programmer, it is important for you to understand that checking leap years in Python is not just about verifying February’s length, it is also related to aligning financial computations, precise date calculations, and creating dependable algorithms.
From the traditional if-else statement to the built-in modules such as “datetime
” and “calendar
“, this guide will cover all major techniques for checking leap years in a program.
Why You Should Check Leap Year in Python
In programming, leap years act as critical markers in timekeeping. It makes sure that our calendars remain accurate and aligned with the rotation of the Earth around the run.
More specifically, verifying whether a year is a leap or not is only about adjusting February’s day.
Here are some of the use cases:
- Date Handling – Accurate handling of dates requires leap-year awareness to ensure precise calculations.
- Scheduling and Reminders – Leap year consideration is essential for scheduling events, especially when recurring annually.
- Financial Applications – In finance, leap years affect interest calculations, loan schedules, and fiscal year planning.
- Scientific Simulations – Accurate temporal simulations require leap-year accounting for realistic outcomes.
- Data Analysis – When analyzing data spanning multiple years, accounting for leap years avoids discrepancies.
How to Check Leap Year in Python
In order to check a leap year in Python, you can use:
- Modulo Operator
- Conditional Statements
- Date Class
- Calendar Module
- List Comprehension
1. Using Modulo Operator
For identifying leap years in Python, the Modulo operator “%
” is considered the most efficient and straightforward approach. In this approach, we will check if a year is evenly divisible by 4 and not divisible by 100 (except when divisible by 400), you can accurately determine the leap years.
For instance, in the provided program, “is_leap_year()
” is a function that uses the modulo operator to check if the year entered by the user satisfies the leap year conditions.
def is_leap_year(year): if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0): return True else: return False input_year = int(input("Enter a year: ")) if is_leap_year(input_year): print(f"{input_year} is a leap year.") else: print(f"{input_year} is not a leap year.")
In this case, we will enter “2020“. Resultantly, “2020” will be identified as a leap year:
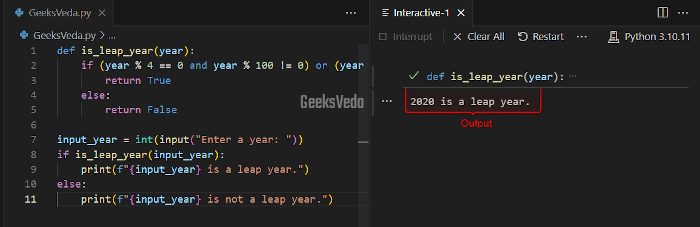
2. Using Conditional Statements
Conditional statements such as if-else provide a direct and readable approach for checking if a year is a leap or not. Using this approach, you can add clear conditions that match leap criteria that can effectively validate whether a year is a leap or not.
Now, in the same function, we have added nested conditional statements for determining the leap year according to the given criteria.
def is_leap_year(year): if year % 4 == 0: if year % 100 != 0 or year % 400 == 0: return True return False input_year = int(input("Enter a year: ")) if is_leap_year(input_year): print(f"{input_year} is a leap year.") else: print(f"{input_year} is not a leap year.")
Entering “2020” will show a message that it is a leap year.
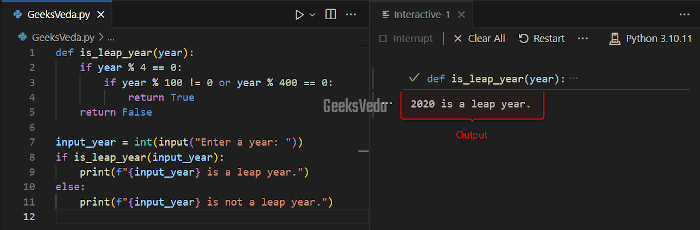
3. Using Date Class
Python’s built-in module offers a “date” class which simplifies the process of leap year checking. With the help of this class, you can effortlessly validate whether a year is a leap or not.
According to the following program, firstly, we will import the “date” class from the datetime module to directly verify if the February of the given year exists. If so happens, the year is considered a leap, otherwise not.
from datetime import date def is_leap_year(year): return date(year, 2, 29).year == year input_year = int(input("Enter a year: ")) if is_leap_year(input_year): print(f"{input_year} is a leap year.") else: print(f"{input_year} is not a leap year.")
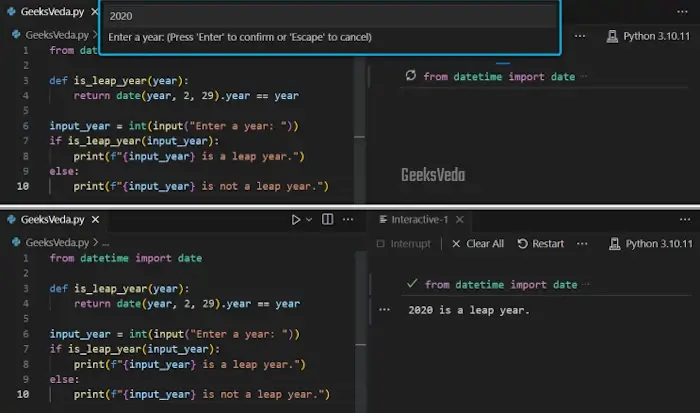
4. Using Calendar Module
The “isleap()
” function of the Python calendar module can be invoked to simplify the leap year determination. This function offers a straightforward approach for checking whether the specified year is a leap or not.
Here, we will first import the “calendar” module and then call its “isleap()
” function for directly checking whether a given year is a leap year.
import calendar def is_leap_year(year): return calendar.isleap(year) input_year = int(input("Enter a year: ")) if is_leap_year(input_year): print(f"{input_year} is a leap year.") else: print(f"{input_year} is not a leap year.")
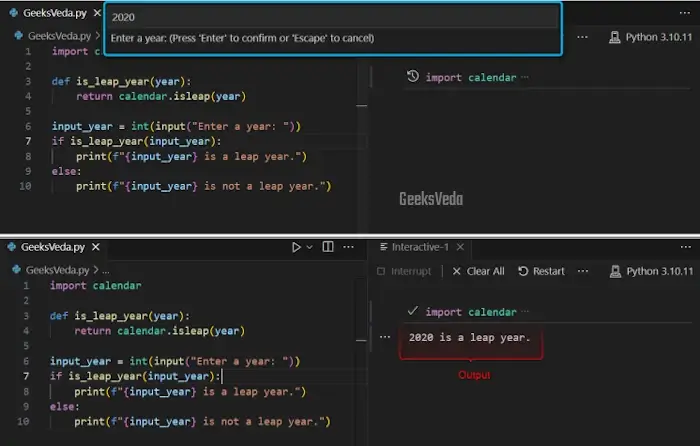
5. Using List Comprehension
List comprehension is a unique approach for generating a list of leap years. With the help of a concise list comprehension expression, you can easily create a list of leap years by specifying the range (start year and end year), as follows.
def is_leap_year(year): if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0): return True else: return False def leap_years_in_range(start, end): return [year for year in range(start, end+1) if is_leap_year(year)] start_year = int(input("Enter start year: ")) end_year = int(input("Enter end year: ")) leap_years = leap_years_in_range(start_year, end_year) print(f"Leap years between {start_year} and {end_year}: {leap_years}")
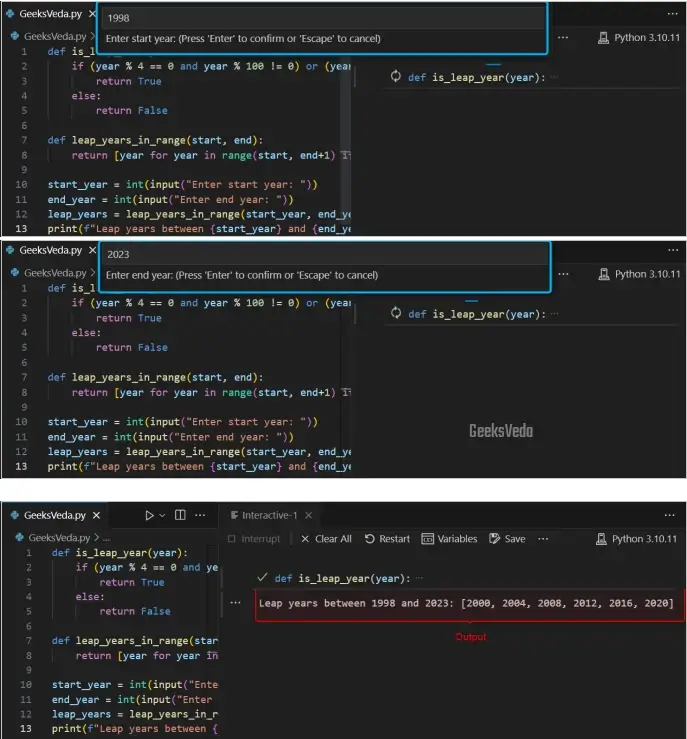
Compare Methods to Check Leap Year in Python
Here is the comparison between the discussed approaches for checking leap year in Python:
Method | Advantages | Disadvantages | Use Cases |
Modulo Operator |
|
|
General-purpose leap year validation with a focus on code simplicity. |
Conditional Statements |
|
|
Accurate leap year validation for any year range, ideal for understanding the leap year rule. |
Using Date Class |
|
|
Handling leap year checks in scenarios involving date manipulation and calculations. |
Using Calendar Module |
|
|
Efficiently handling leap year validation with a focus on using Python’s built-in modules. |
Using List Comprehension |
|
|
Generating lists of leap years within specific year ranges for different programming scenarios. |
That brought us to the end of today’s guide related to checking leap years in Python.
Conclusion
The ability to check whether a year is a leap or not is essential in calculations related to calendar-based Python projects. In today’s guide, we have explored several techniques for checking leap years.
Now, whether you go for the traditional conditional statement, modulo operator, or the built-in modules, select the right approach as per your requirements.
Want to explore and learn more related to Python, Do check out our dedicated Python Tutorial Series!