Welcome to the second article of the Python Exception Handling series! As you code in Python, different errors can pop up unexpectedly, but no worries! There exist different approaches for handling them.
In this article, we will talk about different exception-handling approaches, such as “try-except
“, “try-except-else
“, and “try-except-finally
“, and nested handling. These techniques will assist in managing errors and also make sure that your program runs smoothly.
How to Raise Exceptions in Python
In Python, exceptions can be raised when an error occurs during the program execution. More specifically, you are also permitted to intentionally raise exceptions for handling particular situations.
For the purpose of raising an exception, define the “raise
” statement followed by the exception type and the optional customized error message.
For instance, in the following example, the “divide()
” function raises a “ZeroDivisionError
“, if the divisor “y
” is zero.
When the “divide(10, 0)
” function has been invoked with the “try
” block, the exception will be raised. This exception will be caught by the “except
” block and the defined message will be shown using the print() function.
def divide(x, y): if y == 0: raise ZeroDivisionError("Division by zero is not allowed") return x / y try: result = divide(10, 0) except ZeroDivisionError as e: print(e)
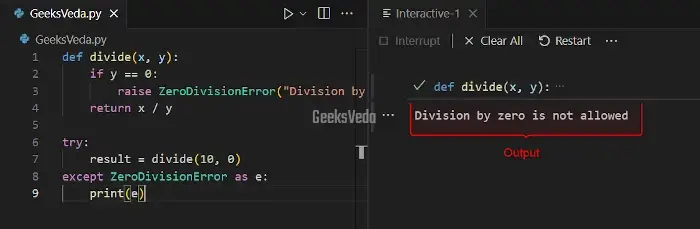
What is a “try-except” Block in Python
The “try-except
” block is specifically used for creating and handling exceptions in Python programs. It enables you to write code that can raise exceptions and handle them without crashing the program.
Additionally, you are permitted to implement different strategies, such as attempting recovery actions or defining default values when exceptions occur.
According to the given code, firstly, you have to enter a number. If the typed number is valid, the division operation will be performed. In the other case, if a “ZeroDivisoonError
” or “ValueError
” occurs, the corresponding error messages will be displayed on the console.
However, if no exception occurs, the “else
” block prints the result.
try: num = int(input("Enter a number: ")) result = 10 / num except ZeroDivisionError: print("Error: Division by zero") except ValueError: print("Error: Invalid input") else: print("Result:", result)
In the first case, we entered “0
” and try to perform the division operation. As a result, the first except block will catch the exception and display the division error on the console.
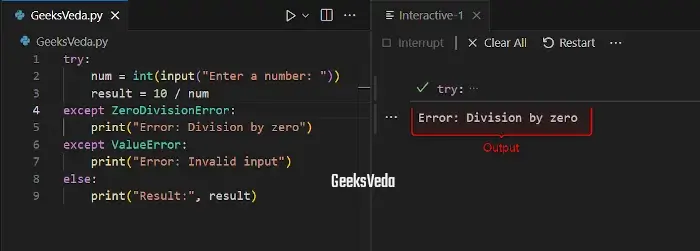
Next, we will give some invalid input to the program. In this case, the second “except” block will run and show the invalid input message.
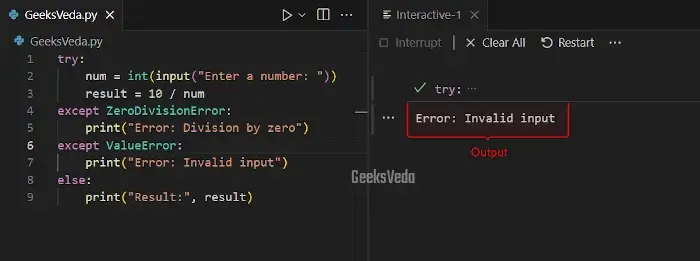
However, when a valid number has been entered, for instance, 5, the program will show “2.0
” as the resultant quotient value.
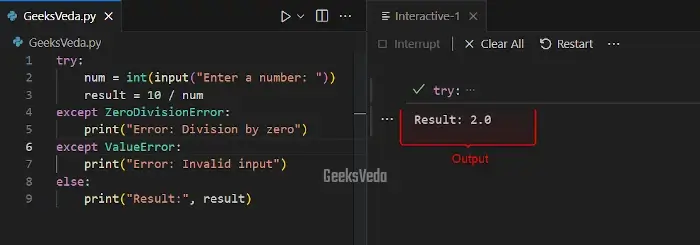
What is “try-except-else” Block in Python
In Python, the “try-except-else
” block is utilized for specifying the code that needs to run if no exception occurs inside the “try
” block.
This approach particularly simplifies the code flow by separating the exceptional and non-exceptional cases. Moreover, it resultantly improves the readability of the program.
For instance, the given code tries to open the file named “example.txt
” in the read mode as “r
“. In case, if the file has not been found, the “FileNotFoundError
” exception will be caught by the except block and the defined error message will be displayed.
However, if the file is found and successfully opened, its content will be read, displayed, and the file will be then closed in the “else
” block.
try: file = open("example.txt", "r") except FileNotFoundError: print("Error: File not found") else: content = file.read() file.close() print("Content:", content)
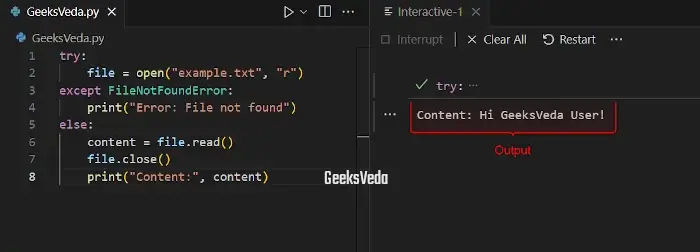
What is “try-except-finally” Block in Python
The “try-except-finally
” block is another approach for exception handling. In this approach, the “try
” block encapsulates code that can raise an exception. On the other hand, the “except
” block handles those exceptions with particular instructions.
However, the “finally
” block makes sure that the cleanup operations, such as closing files or releasing resources have been carried out, regardless of whether the exception has been raised or not.
You can use the “try-except-finally
” block, when it is required to guarantee the execution of a particular code, such as closing the database connection, no matter what would be the output of the preceding code.
In the ongoing example, we have added the “finally
” block for closing the file. N
try: file = open("data.txt", "r") content = file.read() except FileNotFoundError: print("File not found") finally: file.close()
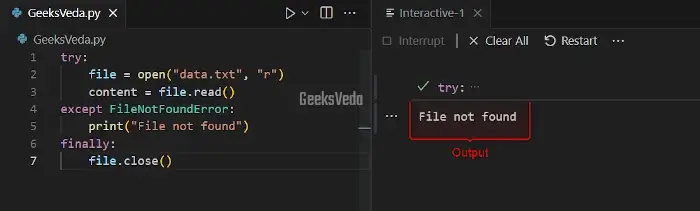
What is Nested Exception Handling in Python
Nested exception handling is all about placing one “try-except
” block within another. This permits the handling of multiple exceptions at several levels of code execution. Additionally, it also prevents unexpected terminations of the program.
You can utilize nested handling when dealing with complex code structures, such as function calls or loops. More specifically, where exceptions can occur at multiple places.
In the below-given program, we have used the nested “try
” block. The inner block attempts to divide a number by zero, caught by “ZeroDivisionError
“.
However, the outer block captures the other exceptions and displays a general error message along with the particular exception.
try: try: x = 10 / 0 except ZeroDivisionError: print("Division by zero") except Exception as e: print(f"An error occurred: {e}")
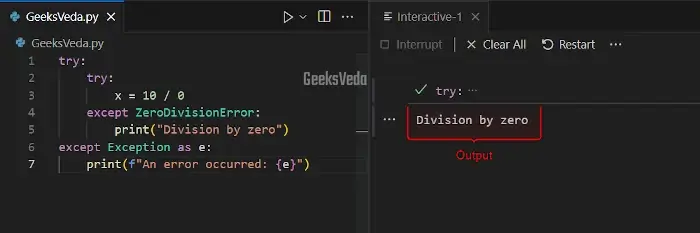
That’s all about different approaches for handling exceptions in your Python program.
Conclusion
Knowing different approaches for Python exception handling is key to writing robust and reliable code. These approaches, such as “try-except
“, “try-except-else
“, and “try-except-finally
“, and nested handling can assist you to manage errors.
Moreover, these techniques also make sure that the program stays stable. Resultantly, you can present your Python skills in an efficient manner.
In the next article of this Python Exception Handling series, we will discuss custom or user-defined exceptions.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!