In this modern technological world, working with JSON data is essential in several applications. More specifically, Python offers a robust library for handling JSON files effortlessly.
So, let’s learn the approaches to reading files and parsing JSON files through today’s guide. Additionally, we will also discuss the step-by-step procedure for opening, reading, and fetching the specific information from the JSON files.
What is JSON Module in Python
In Python, the “json
” module provides the functionalities for handling the JSON data. It permits you to read, parse, and handle the JSON data without any hassle.
With the JSON module, you can easily work with JSON arrays and objects, fetch nested data structures, and perform operations, such as deserialization and serialization.
Here, the provided “test.json
” file represents the typical JSON structure with the key-value pairs, nested objects, and arrays.
{ "name": "Sharqa", "age": 25, "city": "New York", "interests": ["Coding", "Painting", "Article Writing"], "languages": { "english": "fluent", "french": "intermediate" } }
How to Read and Parse a JSON File in Python
To read and parse a JSON file, you can utilize the “json
” module, and use its “load()
” and “loads()
” methods. For the corresponding purpose, check out the given sub-sections.
Using json.load() Method
“json.load()
” is a method supported by the “json
” module in Python which permits you to load JSON data from the mentioned file. It reads the content of the JSON file and then parses it to a Python object.
This method is primarily utilized for reading JSON configuration files, building data pipelines, processing large JSON datasets, integrating with web APIs, and automated testing.
In our scenario, we will use the”json.load()
” to read the parse the given “test.json
” file. To do so, first, we imported the “json
” module.
Then, the open()
function is invoked for opening the JSON file and assigning it to the “file variable
“.
After that, the “load()
” method is called and we have passed a file as an argument to it. Resultantly, the JSON content will be parsed into a Python object and assigned to the “data
” variable.
Lastly, we have fetched specific information from the parsed JSON data by utilizing the keys as the dictionary indices.
import json # Opening the JSON file with open('test.json') as file: # Loading the JSON data data = json.load(file) # Accessing and printing specific information from the parsed JSON data name = data['name'] age = data['age'] city = data['city'] interests = data['interests'] print(f"Name: {name}") print(f"Age: {age}") print(f"City: {city}") print(f"Interests: {interests}")
Here, we have retrieved the values for the “name
“, “age
“, “city
“, and “interests
” keys.
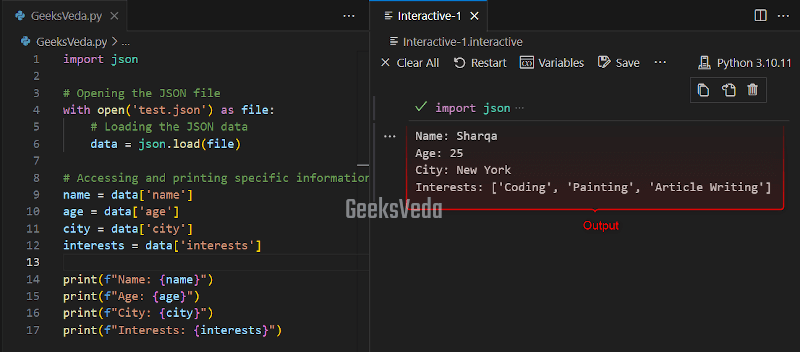
Using json.loads() Method
The “json.loads()
” method parses the JSON string and converts it into another Python object. Additionally, it can be used for parsing JSON data from an API response, handling JSON data in memory, and transforming and manipulating data.
Now, in the following code, the “test.json
” file is opened with the “open()
” function in read mode. Then, its contents are read as a string by invoking the “read()
” method.
After that, the “loads()
” method is called for parsing the JSON string and converting it to a Python object.
As a result, it will be stored in the “data
” variable, which is then used for accessing and printing the JSON data, including “name
“, “age
“, “city
“, and “interests
“.
import json # Reading the JSON file as a string with open('.json') as file: json_str = file.read() # Parsing the JSON string data = json.loads(json_str) # Accessing and printing specific information from the parsed JSON data name = data['name'] age = data['age'] city = data['city'] interests = data['interests'] print(f"Name: {name}") print(f"Age: {age}") print(f"City: {city}") print(f"Interests: {interests}")test
As you can see, we have successfully read and parsed the “test.json
” file.
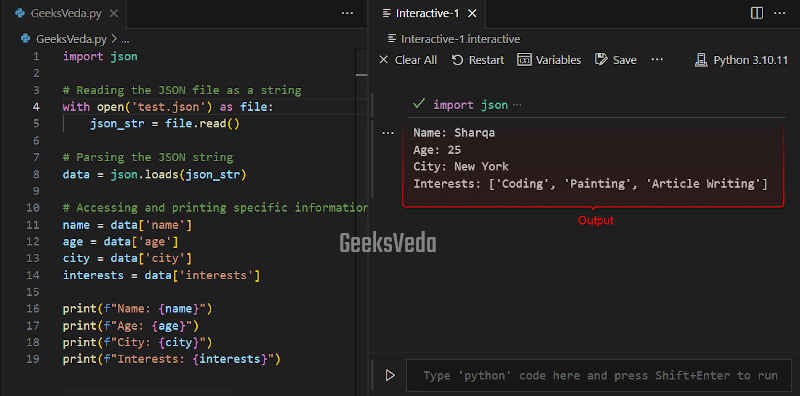
Differences Between JSON load() and JSON loads() Methods
Have a look at the given table stating the key differences between the load()
and loads()
methods of the JSON module.
load() | loads() | |
Purpose | Reads and parses JSON data from a file. | Parses JSON data from a string. |
Input | File object | JSON string |
Usage/Syntax | “json.load(file) “ |
“json.loads(json_str) “ |
Output | Python object | Python object |
String interaction | Not applicable | JSON string must be provided |
File interaction | Reads the JSON data from the file directly | The file must be opened and its content read as a string |
Error handling | Display “JSONDecodeError ” if JSON is not well-formed |
Shows “JSONDecodeError ” if JSON is not well-formed |
How to Convert a JSON File to Python Objects
In case your application receives JSON data from any external source, you can invoke the “json.load()
” method for converting it into a Python object. Moreover, it will make it easy for you to access particular attributes or perform any specific calculations.
For instance, in the provided program, the “open()
” function opens the “test.json
” file and the “json.load()
” method reads and parses its data, converting it into a Python object.
The resultant object will be stored in the “data
” variable and then displayed on the terminal with the “print()
” function.
import json # Converting JSON file to Python object with open('test.json') as file: data = json.load(file) print(data)
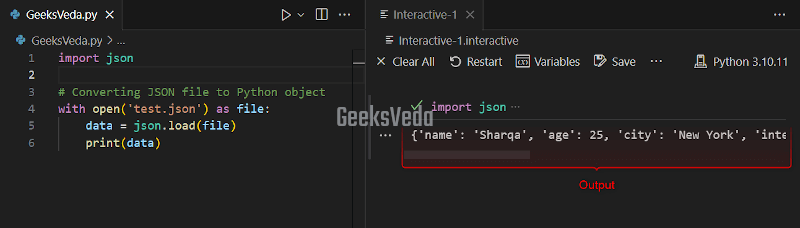
How to Convert Python Objects to a JSON File
The JSON module provided another method named “dump()
” that can be used for the purpose of converting Python objects to JSON. This method accepts a Python object as an argument and writes it as JSON data to the specified file. You can utilize the “json.dump()
” method for storing Python object data as JSON or to interact with JSON-based APIs.
Here, a Python dictionary “data” is created having key-value pairs representing an object. Then, the “open()” function is involved to open the file in the write mode “w
“.
Next, the “json.dump()
” method writes a serialized JSON representation of the “data
” object to the “test.json
” file.
import json # Converting Python object to JSON file data = {"name": "Alexa", "age": 35} with open('test.json', 'w') as file: json.dump(data, file)
It can be observed that the Python object attributes with their values have been stored in the json file.
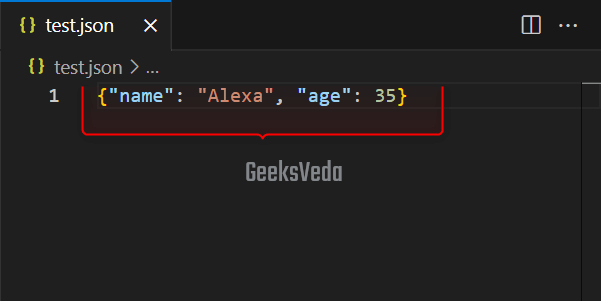
How to Convert Custom Python Objects to a JSON File
You can also utilize the “json.dump()
” method for converting custom Python objects to a JSON file along with the “__dict__
” attribute of the object. This approach is implemented in the scenario where it is required to serialize the custom objects or to exchange data between different systems.
In the given program, we have defined a custom class named “Author
“‘ with the “name
” and “age
” attributes. Then, we created an instance “author
” of this class.
After that, we converted this object to JSON by accessing the “__dict__
” attribute of the author and specifying the corresponding file as a parameter.
Resultantly, the JSON data will be written to the “test.json
” file.
import json # Custom Python object class Author: def __init__(self, name, age): self.name = name self.age = age # Creating an instance of the custom object author = Author("Sharqa", 25) # Converting the custom object to JSON and writing to a file with open('test.json', 'w') as file: json.dump(author.__dict__, file)
As you can see, the custom object has been successfully converted into JSON data.
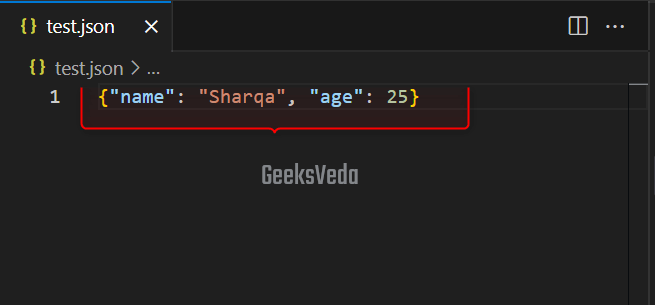
Best Practices for Reading and Parsing a JSON File Using Python
Have a look at the given best practices for reading a JSON file in Python.
- Utilize the “
json
” module to work with JSON data. - Open the file and select the most suitable method, like “
json.load()
” for file-based JSON reading and parsing or “json.loads()
” for reading and parsing string-based JSON. - Verify the access and structure of the data using the parsed JSON.
- Close the file after performing the required operations.
- Maintain code readability and follow coding conventions.
- Thoroughly test the JSON parsing with different scenarios and files.
- Handle errors and implement error handling.
That’s all from today’s guide related to reading and parsing a JSON file in Python.
Conclusion
Mastering the skill of reading and parsing JSON files will allow you to manipulate the data as per your requirements. By utilizing the “json
” module, you can effortlessly convert the JSON data to Python objects, navigate, and fetch the specified information from the JSON structures.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!