In Python programming, there come situations where it is required to filter through data for extracting particular elements. This can be done with the “filter()
” function.
In today’s guide, we will explore the fundamental aspects of the Python filter()
function, its usage, and its applications such as filtering with lambda function, regular functions, and combining filter()
with other functions. Moreover, we will also compare the filter()
method with other alternative approaches.
What is filter() Function in Python
Python’s “filter()
” function is a robust tool that can be utilized for selectively extracting elements from iterable objects based on the specified condition. It behaves as a data filter and enables you to focus on particular elements that meet your criteria.
This ultimately improves the logic and efficiency of the data processing.
What is Filtering in Python
Filtering or filtration is a fundamental operation in Python that is based on the extraction of the elements that satisfy the defined condition.
This functionality can be achieved with the help of the “filter()
” function. This function accepts a filtering function and an iterable as an input and outputs an iterator comprising the elements that passed the criteria.
How Do You Use filter() Function in Python
In order to use the “filter()
” function, pass two arguments that can be a filtering function and an iterable. The filtering function will then return “True” or “False” according to the filtered result.
After that, the “filter()
” function iterates through the iterable and applies the filtering function to each element, and outputs an iterator that will contain the filtered elements.
For instance, in the provided code, the “is_even()
” function defines the filtering condition for identifying even numbers. Then, the “filter()
” function applies the “is_even()
” function to each element of the “numbers” list and retains only those that satisfy the mentioned condition.
def is_even(num): return num % 2 == 0 numbers = [11, 22, 33, 44, 55, 66, 77, 88, 99, 110] even_numbers = list(filter(is_even, numbers)) print(even_numbers)
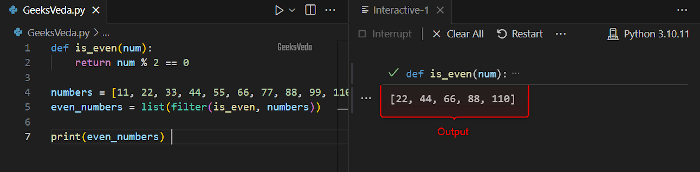
Filtering with Lambda Functions
Lambda functions offer a concise way of creating small, inline functions that can be used for filtering data.
More specifically, combining the “filter()
” with lambda enables you to define filtering conditions within the function call of the filter()
function. Moreover, you are not required to add separate function definitions.
According to the provided code, we have used a lambda function within the “filter()
” function call for filtering the even numbers from the “numbers” list.
x % 2 == 0
“.numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) print(even_numbers)
It can be observed that the filtered even numbers have been displayed on the console.
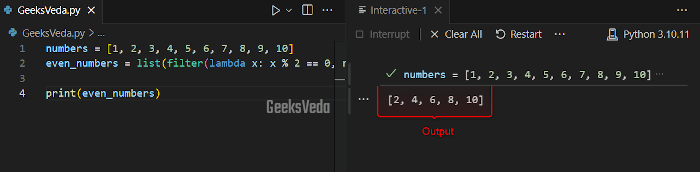
Filtering with Regular Functions
Python regular functions provide a more versatile approach for filtering data. This methodology enables you to encapsulate complex filtering logic. Moreover, by defining a separate function and passing it to the “filter()
” function, you can easily handle the filtering condition.
Here, the “is_divisible_by_three()
” function is defined for filtering numbers that are divisible by three. Then, the “filter()
” function applies the mentioned function to the “numbers” list elements and only retains those who meet the filtering criterion.
def is_divisible_by_three(num): return num % 3 == 0 numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] divisible_by_three = list(filter(is_divisible_by_three, numbers)) print(divisible_by_three)
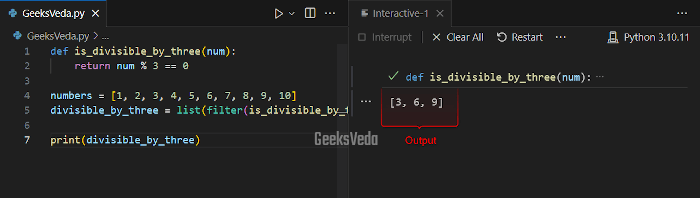
This is how regular functions can be employed for handling complex filtering requirements with the “filter()
” function.
Combine filter() with Other Python Functions
The “filter()
” function becomes even more powerful when combined with other built-in Python functions like “map()” and “reduce()
“.
This combination permits you to create an expressive and efficient data transformation pipeline that manipulate, filter, and aggregate data easily.
1. Using map() with filter() Function
In the following example, the “filter()
” function is invoked for extracting even numbers from the “numbers” list. The resultant even numbers are then passed to the map()
function, which calculates the squares of each even number.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] filtered_squared_even = list(map(lambda x: x ** 2, filter(lambda x: x % 2 == 0, numbers))) print(filtered_squared_even)
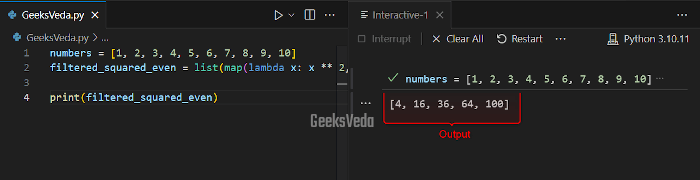
2. Using reduce() with filter() Function
Here, the “filter()
” function extracts the even numbers from the “numbers
” list. Then, the “reduce()
” function is applied for filtering even numbers and multiplying them together for calculating their product.
from functools import reduce numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] product_of_even = reduce(lambda x, y: x * y, filter(lambda x: x % 2 == 0, numbers)) print(product_of_even)
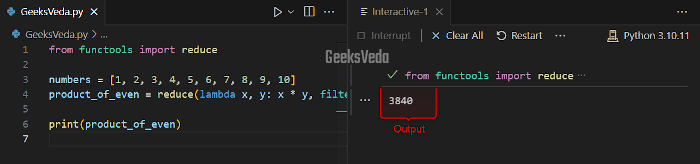
Filtering Data Types and Structures
The versatility of the “filter()” function extends to several data types and is structured in Python. This makes it a valuable tool for handling diverse datasets.
Whether working with lists, dictionaries, strings, or other iterable objects, the filter() function can filter and extract the element according to the defined criteria.
For instance, in the provided code, the “filter()
” function is invoked for extracting words from the lists named “words
“. The condition is specified as the words should have a length of six characters or more.
Then, the lambda function evaluates the length of each word with the “len()
” function, and the “filter()
” function retains words that satisfy the specified condition.
words = ['apple', 'banana', 'cherry', 'date', 'elderberry'] filtered_words = list(filter(lambda x: len(x) >= 6, words)) print(filtered_words)

The Python filter() Function VS Alternative Approaches
Here is the head-to-head comparison of the Python filter()
function with other alternative approaches such as list comprehension and loops:
Aspect | Python filter() Function | List Comprehension | Loop |
Code Readability | Offers concise and expressive syntax. | Provides compact syntax with comprehension. | Involves more code and nesting. |
Efficient Memory Usage | Filters elements one at a time, minimizing memory usage. | Creates a new list, consuming more memory. | Requires intermediate lists. |
Streamlined Filtering | Straightforward filtering using a single function. | Combines filtering and expression in one line. | Involves separate steps for filtering and expression. |
Dynamic Filtering Conditions | Adaptable with both lambda and regular functions. | Allows dynamic conditions within comprehension. | Requires explicit condition adjustment. |
Data Transformation | Limited to filtering elements from iterable. | Can include expression transformation within comprehension. | Requires separate steps for transformation. |
Performance Efficiency | Efficient for large data sets with minimal overhead. | Performs well but may create intermediate lists. | Performs well but may create intermediate lists. Performance impact with large data. |
Complexity | Simplifies filtering tasks, reducing complexity. | Keeps filtering and transformation in one step. | Involves separate filtering and transformation steps. |
Resource Optimization | Optimizes processing resources efficiently. | Efficient, but intermediate lists may impact performance. | May consume more memory and processing power. |
That brought us to the end of this effective guide related to the Python filter() function.
Conclusion
Python’s “filter()
” function is a versatile tool that can be considered for sorting data. It can be utilized for extracting elements that meet the defined criteria.
Whether you are working with dictionaries, lists, or data structures, “filter()
” provides a concise solution that improves the readability of code and also optimizes resource usage.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!