In Python programming, the “chain()
” function is a versatile tool that has been designed for simplifying the iteration process. It can be used for handling multiple iterables or combining and iterating over diverse data sources.
Today’s guide is all about Python’s “chain()
” function, its usage, its relation with Iteration, chaining lists and iterables, and chaining lists with other functions.
Moreover, we will also provide a head-to-head comparison between the chain()
function and the other alternative approaches.
What is chain() Function in Python
The Python “chain()
” function is a robust tool that permits you to combine multiple iterables into single streams of elements. Additionally, it simplifies the iteration process over several data sources, which resultantly makes the code more readable and concise.
With the “chain()
” function, you can concatenate lists, strings, tuples, and other iterable objects. This creates a unified sequence that can simplify complex iteration operations.
Relationship Between Iteration and Chaining in Python
Knowing the relationship between iteration and chaining is one of the most fundamental concepts in Python. Iteration is based on the sequential processing of elements in an iterable.
Whereas, “chain()
” takes this concept further by permitting you to iterate over multiple iterables as a single unit.
Therefore, by understanding the relationship between iteration and chaining, you can efficiently manipulate diverse data sources within a single loop.
How to Use chain() Function in Python
In order to use the “chain()
” method, import the “itertools
” mobile and then specify the iterables (as arguments) that you want to chain. This function outputs an iterator that iterates through all elements of the input iterable in the provided order.
More specifically, by using the chain()
function you can merge different data structures and perform complex tasks that are based on multiple sequences.
In the below code, we will invoke the “chain()” function for concatenating the elements from the “list1“, “list2
“, and “text into a single iterable.
As a result, you will see a combined sequence that also retains the order of the original elements.
from itertools import chain # Chaining lists and strings list1 = [1, 2, 3] list2 = [4, 5, 6] text = "Python" result = list(chain(list1, list2, text)) print(result)
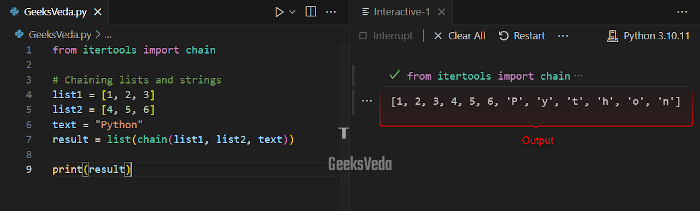
Chaining Lists and Iterables in Python
Chaining lists and other iterable objects can simplify the combination of multiple data sources into a single unified sequence. Moreover, by chaining lists, tuples, and other objects, you can maintain the code flow and make your program more efficient for handling data collections.
For instance, in the following example, we have three different iterables named “list1“, “list2“, and “list3“. All of these lists will be chained together with the “chain()
” function.
from itertools import chain list1 = [1, 2, 3] list2 = [4, 5, 6] tuple1 = (7, 8, 9) result = list(chain(list1, list2, tuple1)) print(result)
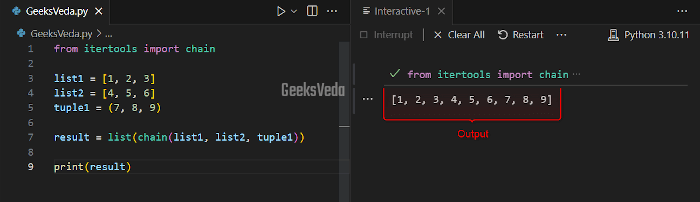
Chaining Iterables of Different Types in Python
You can have an idea about the versatility of the “chain()
” function with this statement that the “chain()
” function can be extended to combine iterable different types.
This permits you to create a single stream from disparate data structures.
Whether you need to merge strings, dictionaries, lists, or custom iterable objects, the “chain()
” function offers a solution for handling diverse data with a single iteration loop.
Here, the “chain()
” function combines a string, dictionary values, and a list of numbers into a single iterable.
from itertools import chain string = "Python" dictionary = {'a': 1, 'b': 2} numbers = [3, 4, 5] result = list(chain(string, dictionary.values(), numbers)) print(result)
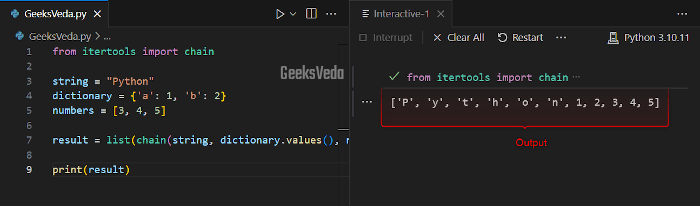
Combine chain() with Other Python Functions
The pure functionality of the “chain()
” function becomes evident when it is combined with other Python functions such as map(), filter(), and zip(). These combined approaches allow you to create sophisticated pipelines that can efficiently manipulate and process data.
1. Using chain() Function with map() Function
Python’s “map()
” function is utilized for applying a particular operation to each element of an iterable. Moreover, when this function is combined with “chain()
“, it transforms and concatenates multiple iterables in a single loop.
In the provided code, the map()
function is invoked for calculating the length of the names present in the “names” list. This results in an iterable comprising the length of the names.
After that, the “chain.from_iterable()
” method is called that concatenates the individual name length into a single sequence.
from itertools import chain names = ['Sharqa', 'Zoha', 'Hanaf'] name_lengths = map(len, names) result = list(chain.from_iterable([name_lengths])) print(result)
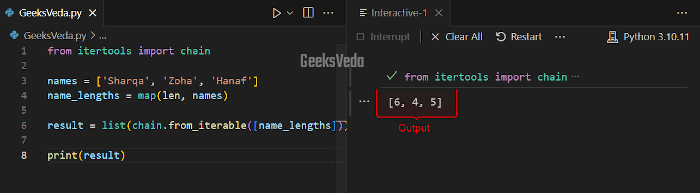
2. Using filter() with chain() Function
The “filter()
” function can be used for selectively including elements from an iterable according to the specified condition. When combined with “chain()
“, this function can easily filter and concatenate multiple iterable and create a cohesive sequence.
Here, the “filter()
” function is utilized for separating the odd and even number from the “numbers” list. As a result, two separate filtered iterables “odd” and “evens” will be created.
Then, by applying the “chain()
” function to these filtered iterable, the even and odd numbers will be concatenated into a single sequence with the same order.
from itertools import chain numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9] evens = filter(lambda x: x % 2 == 0, numbers) odds = filter(lambda x: x % 2 != 0, numbers) result = list(chain(evens, odds)) print(result)
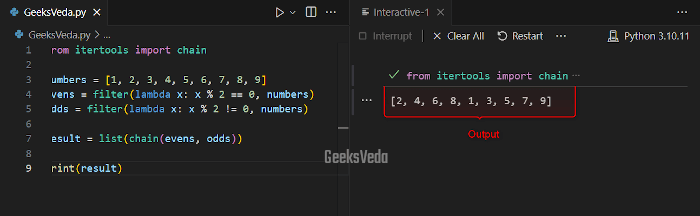
3. Using zip() with chain() Function
The zip() function pairs the respective elements from the multiple iterables and creates a tuple of matched values. More specifically, when it gets combined with the “chain()
” function, you can concatenate and interleave multiple iterables.
According to the given program, the “zip()
” function pairs elements from the “names” and “scores” lists and creates tuples with the respective values.
By invoking the “chain.from_iterable()
” method, the tuple will be flattened into a single sequence. This will interleave names and core while maintaining the respective order.
from itertools import chain names = ['Sharqa', 'Zoha', 'Tulsi'] scores = [85, 92, 68] result = list(chain.from_iterable(zip(names, scores))) print(result)
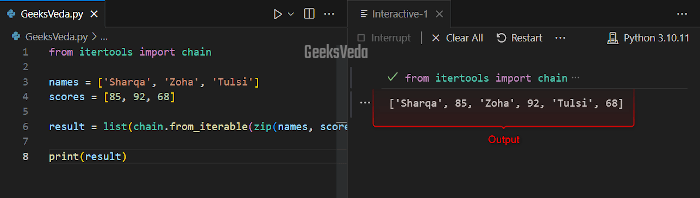
Benefits of Using Python chain() Function
Here are some of the commendable benefits of utilizing Python “chain()
” function:
- Efficient Iteration: Simplifies handling multiple iterables in a single sequence.
- Code Simplicity: Reduces nesting, leading to cleaner and more readable code.
- Versatile Data Manipulation: Seamlessly combines various iterable types.
- Dynamic Pipelines: Collaborates with functions like
map()
,filter()
, and zip() for dynamic iterations. - Optimized Resource Usage: Prevents unnecessary memory consumption.
- Flexible Concatenation: Easily merges different iterable objects.
- Improved Performance: Reduces overhead and enhances memory efficiency.
- Modular Code Design: Enables reusable and adaptable code components.
Compare chain() with Alternative Approaches
Let’s compare the Python chain()
function with the other alternative approaches such as nested loops and list concatenation:
Aspect | chain() Function | Nested Loops | List Concatenation |
Code Readability | Simplifies code with cleaner and concise syntax. | May result in nested loops, reducing readability. | May lead to complex and less readable code. |
Iteration Efficiency | Optimizes memory usage by processing one at a time. | May create intermediate lists, consuming memory. | Can result in memory inefficiency. |
Versatility | Combines various iterables regardless of types. | Limited to specific data types or structures. | May restrict flexibility in data handling. |
Dynamic Pipelines | Easily integrates with functions like map(), filter(), and zip(). | Requires extra code for combining functions. | May complicate code and require additional functions. |
Modularity | Promotes modular design with reusable code. | May lead to duplicated or hard-to-maintain code. | Can result in code duplication and lack of reusability. |
Performance | Efficiently handles large data without performance loss. | May slow down with increasing data size. | Can lead to reduced performance and longer execution times. |
Complexity | Reduces nested structures and simplifies code. | May introduce complexity with nested loops. | Can create complex and harder-to-maintain code. |
Resource Usage | Optimizes memory and processing resources. | Can consume more memory and processing power. | May result in higher resource consumption. |
That brought us to the end of this informative guide related to the Python “chain()” function.
Conclusion
Python’s “chain()
” function can be utilized for simplifying complex operations related to iteration. It also assists in combining multiple iterables into a single sequence. This consequently improves the code readability and also optimized memory usage.
Additionally, you can also combine the chain() function with other Python functions, such as map(), filter(), and zip() for enabling dynamic iteration pipelines.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!