In today’s guide, we will discuss the Python “zip()
” function. This function allows you to perform data synchronization in all possible ways. Moreover, it assists in aligning multiple iterables and revolutionizing the procedure to manipulate data.
By the end of this guide, we will be able to transform your data and enhance your coding skills even more. So, let’s get started!
What is zip() Function in Python
Python’s “zip()
” function is a robust tool that enables you to combine multiple iterables, such as tuples
, and lists
, into a single zipped object. This function aligns the respective elements from each iterable for synchronized data manipulation.
With the help of the “zip()
” function, you can merge the datasets, iterate through paired values and perform several operations that are based on parallel data processing.
Synchronize Data with Python zip() Function
Data synchronization is an essential aspect of Python programming, especially when you are dealing with multiple datasets that need to be aligned. In such scenarios, the “zip()
” function serves as the synchronization mechanism. It permits you to pair the elements from different iterables according to their positions.
With the “zip()
” function, you can make sure that the respective data points remain aligned throughout the code, which ultimately improves the accuracy and reliability of the data-driven operations.
For instance, in the following example, the “zip()
” function is invoked within the for loop for iterating through the synchronized pairs of names and scores.
names = ['Sharqa', 'Hanaf', 'Zoha'] scores = [85, 92, 78] for name, score in zip(names, scores): print(f"{name}: {score}")
It can be observed that the loop successfully printed each name and its respective score.
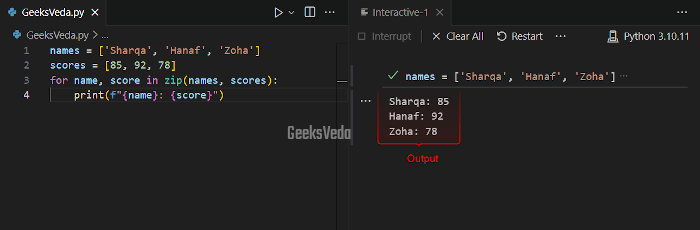
How to Use zip() Function in Python
Python “zip()
” function accepts one or more iterables as arguments and outputs a zipped object. This object pairs the corresponding elements from each iterable.
Moreover, to work with the zipped data, you can convert it to a list, tuple, or any other iterable.
Whether you want to combine lists, tuples, or any other iterable objects, utilize “zip()
” to synchronize and manipulate data across multiple dimensions.
Here, the “zip()
” function combines the “names” and “ages” lists into a zipped object, which will be then converted into a list
with the list()
” function.
names = ['Sharqa', 'Hanaf', 'Zoha'] ages = [25, 30, 28] zipped_data = zip(names, ages) zipped_list = list(zipped_data) print(zipped_list)
The resultant “zipped_list
” comprises tuple pairing, each name with its respective age.
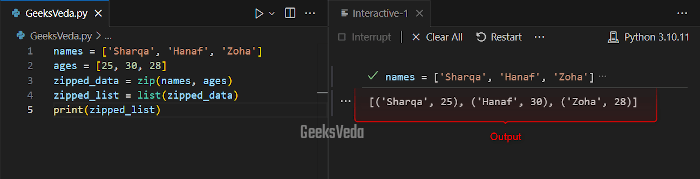
Create Zipped and Unzipped Objects in Python
In order to create zipped objects, use the “zip()
” function to merge multiple iterables into a synchronized entity. After zipping the objects, you can easily reverse this process for regaining the original individual iterables. This technique is called “unzipping“.
Zipping and unzipping objects enable you to manage and manipulate the aligned data efficiently.
Here, the “zip()
” function creates a zipped object “zipped_data” by combining “names” and “scores” lists.
*
” operator is utilized for unpacking the zipped data into two different separate iterables named “unzipped_names” and “unzipped_scores“.Lastly, the unzipped iterables will be printed on the console.
names = ['Sharqa', 'Hanaf', 'Zoha'] scores = [85, 92, 78] zipped_data = zip(names, scores) # Creating a zipped object unzipped_names, unzipped_scores = zip(*zipped_data) # Unzipping print(list(unzipped_names)) print(list(unzipped_scores))
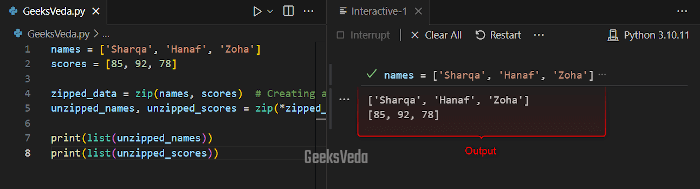
Handling Unequal Length Iterables
You can also utilize the “zip()
” function for gracefully handling cases where the input iterables are of different lengths. This function aligns the elements according to the shortest iterable.
By doing so, the “zip()
” function makes sure that synchronization occurs only up to the length of the shortest sequence.
For instance, in the provided code, the “zip()
” function synchronizes “names” and “scores” lists.
As the “scores” list has fewer elements, so only the first two pairs will be considered in the loop. This consequently prevents index-related errors.
names = ['Sharqa', 'Hanaf', 'Zoha'] scores = [85, 92] zipped_data = zip(names, scores) for name, score in zipped_data: print(f"{name}: {score}")
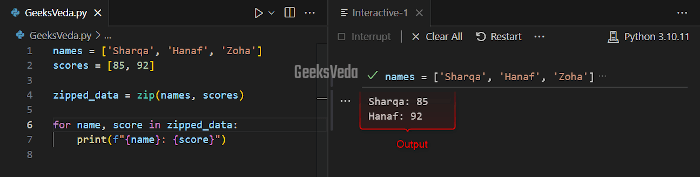
Combine zip() with Other Python Functions
Python allows you to combine the “zip()
” function with other Python functions such as “map()
” and “filter()
“. By using these combinations, you can transform and filter synchronized data with ease.
1. Using map() with zip() Function
Here, the zip()
function has been combined with map() Function for the assignment of grades based on the scores.
names = ['Sharqa', 'Hanaf', 'Zoha'] scores = [85, 92, 78] def grade(score): if score >= 90: return 'A' elif score >= 80: return 'B' else: return 'C' zipped_data = zip(names, scores) graded_data = list(map(lambda x: (x[0], grade(x[1])), zipped_data)) print(graded_data)
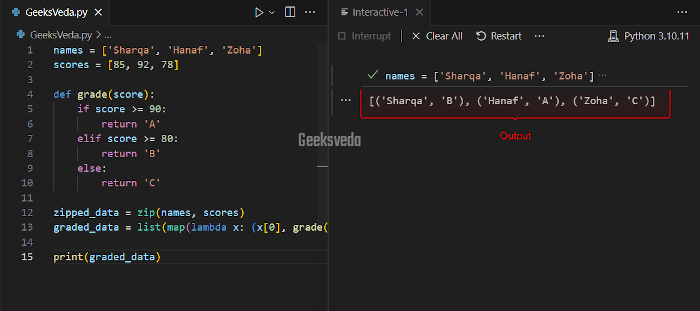
2. Using filter() with zip() Function
In this example, we have combined the “zip()
” function with filter() Function for retaining only the high-achiever. Through this approach, we will get a list of tuples containing the names and scores that meet the specified filtering criteria.
names = ['Sharqa', 'Hanaf', 'Zoha'] scores = [85, 92, 78] zipped_data = zip(names, scores) high_achievers = list(filter(lambda x: x[1] >= 90, zipped_data)) print(high_achievers)
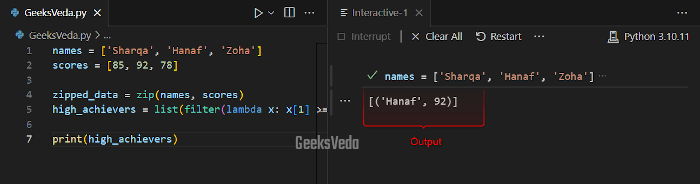
Explore Advanced zip() Techniques
You can also explore the advanced techniques of the “zip()
” function. For instance, from transposing matrices to parallel iteration, these approaches demonstrate the creative possibilities and versatility of the “zip()
” function.
1. Transpose a Matrix Using zip() Function
According to the provided code, the “zip()
” function transposes a matrix by combining its columns into rows. Here, the “*
” operator helps in unpacking the matrix columns, which will be then unzipped and converted back into rows with list comprehension.
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] transposed_matrix = [list(row) for row in zip(*matrix)] print(transposed_matrix)
As a result, you will observe a matrix that has the following rows and columns.
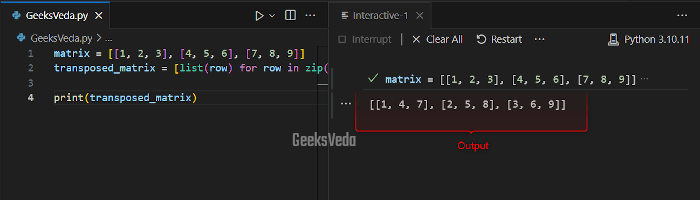
2. Using zip() Function for Parallel Iteration
In this example, the “zip()” function is utilized for performing the parallel iteration through the “names“, “scores“, and “grades” list. This enables simultaneous access to respective elements.
names = ['Sharqa', 'Hanaf', 'Zoha'] scores = [85, 92, 78] grades = ['B', 'A', 'C'] for name, score, grade in zip(names, scores, grades): print(f"{name}: Score - {score}, Grade - {grade}")
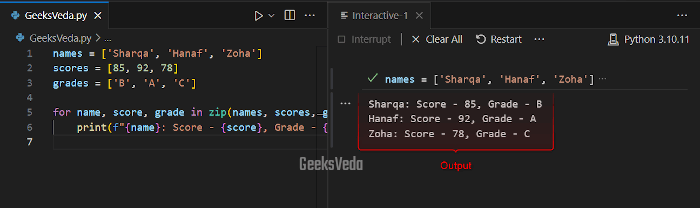
3. Combine with Dictionary Comprehension
Here, the “zip()
” function collaborates with the dictionary comprehension for creating a dictionary named “person_dict” by pairing keys and values.
keys = ['name', 'age', 'country'] values = ['Sharqa', 25, 'Pakistan'] person_dict = {key: value for key, value in zip(keys, values)} print(person_dict)
Resultantly, you will get a dictionary where each key is matched with its corresponding value.
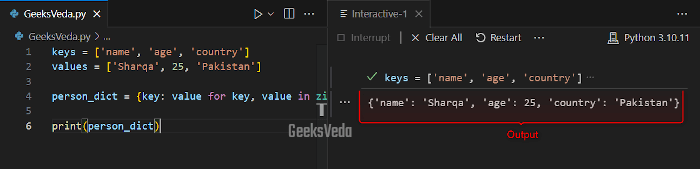
Compare zip() Function with Alternative Approaches
Let’s compare Python’s “zip()
: function with its alternative approaches which are manual iteration and using “zip()
” with list comprehension:
Aspect | Python zip() Function | Manual Iteration | zip() with List Comprehension |
Synchronization | Effortlessly synchronizes iterables. | Requires explicit iteration management. | Streamlines synchronization and pairing. |
Readability | Enhances code clarity with concise syntax. | Involves more verbose and complex code. | Offers a balanced blend of readability and brevity. |
Flexibility | Handles varying data sources uniformly. | May need separate logic for different cases. | Maintains flexibility while simplifying the process. |
Complexity Reduction | Simplifies complex data pairing tasks. | Involves manual pairing and indexing. | Reduces complexity through streamlined pairing. |
Error Avoidance | Minimizes the chances of indexing errors. | Prone to off-by-one errors and typos. | Helps prevent common errors through parallel pairing. |
Performance Efficiency | Efficient for large-scale data handling. | May lead to multiple iterations. | Efficiently manages parallel data processing. |
Elegance and Expressiveness | Offers an elegant and expressive solution. | Lacks the elegance of a built-in function. | Balances elegance with simplicity. |
Resource Optimization | Optimizes memory and processing resources. | May consume more resources with multiple loops. | Efficiently utilizes resources for parallel tasks. |
Parallel Processing | Supports simultaneous iteration through iterables. | Requires separate loops for parallel processing. | Facilitates parallel iteration with synchronization. |
Function Chaining | Easily integrates with other functions. | Requires manual integration and transformation. | Enables seamless chaining for complex operations. |
That’s all from this effective guide related to the Python zip() function.
Conclusion
With the help of the Python “zip()
” function, you can synchronize and pair different pieces of information for organizing data. Moreover, by utilizing this function, you can smoothly bring together the data.
So, understand the zip()
function through our today’s guide and apply it to your Python project and solve your complex problems effortlessly.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!